FFmpeg
|
ass_split.h
Go to the documentation of this file.
ASSSplitContext * ff_ass_split(const char *buf)
Split a full ASS file or a ASS header from a string buffer and store the split structure in a newly a...
Definition: ass_split.c:303
including without damages for loss of work computer failure or loss of data or any commercial damage and lost revenue or even if the UPF has been advised of the possibility of such damage the UPF will be liable under statutory product liability laws as far such laws apply to the Software The foregoing limitations will apply even if the above stated warranty disclaimer fails of its essential purpose Some jurisdictions do not allow the exclusion of indirect or consequential so some of the terms above may not be applicable to you AUDIT Upon days written UPF or a professional designated by UPF may audit your use of the Software You agree to cooperate with UPF s audit and provide reasonable assistance and access to information You agree to pay within days of written notification any fees applicable to your use of the Software in breach of your license rights If you do not UPF can end your license You agree that UPF shall not be responsible for any of your costs incurred in cooperating with the audit TERMINATION This Agreement is effective until terminated You may terminate this Agreement at any time by destroying all copies of Software This Agreement will terminate immediately without notice from UPF if you fail to comply with any provision of this Agreement Either party may terminate this Agreement immediately should any Software or in either party s opinion be likely to the subject of a claim of infringement of any intellectual or industrial property right Upon you must destroy all copies of Software Provisions that survive termination or expiration include those relating to limitation of liability limitation and others which by their nature are intended to and apply to both parties respective successors and assignees EXPORT REGULATIONS Software and technical data delivered under this Agreement may be subject to export or import regulations You agree to comply strictly with all such laws and regulations and acknowledge that you have the responsibility to obtain such licenses to re or import as may be required after delivery to you TRADEMARKS This Licence does not grant permission to use the trade service or names of the except as required for reasonable and customary use in describing the origin of the Software and reproducing the content of the copyright notice GOVERNING LAW JURISDICTION Any action related to this Agreement will be governed by Spanish law No choice of law rules of any jurisdiction will apply Any conflict or dispute resulting from this License and use of the Software will be subject to the exclusive jurisdiction of the courts of the City of Spain SEVERABILITY If any provision of the Licence is invalid or unenforceable under applicable this will not affect the validity or enforceability of the Licence as a whole Such provision will be construed and or reformed so as necessary to make it valid and enforceable If this is not the License will terminate ASSIGNMENT You may not assign this agreement or give or transfer the Software or an interest in it to another individual or entity If you grant a security interest in the the secured party has no right to use or transfer the Software FORCE nor is UPF responsible for any third party claims against Licensee PRIVACY desenvolupament i under the responsibility of Universitat Pompeu Fabra works or projects In but without the data is processed for the purpose of communicating with Licensee regarding any administrative and legal judicial purposes Collection We collect personal data including affiliation and email address Only the data marked with a star is obligatory Users must provide true and accurate personal profile data Users must NOT upload any sensitive data regarding racial origin
Definition: MELODIA - License.txt:31
text(-8, 1,'a)')
char * text
actual text which will be displayed as a subtitle, can include style override control codes (see ff_a...
Definition: ass_split.h:61
int alignment
position of the text (left, center, top...), defined after the layout of the numpad (1-3 sub...
Definition: ass_split.h:48
Definition: ass_split.c:168
ASSStyle * ff_ass_style_get(ASSSplitContext *ctx, const char *style)
Find an ASSStyle structure by its name.
Definition: ass_split.c:465
Set of callback functions corresponding to each override codes that can be encountered in a "Dialogue...
Definition: ass_split.h:119
void ff_ass_split_free(ASSSplitContext *ctx)
Free all the memory allocated for an ASSSplitContext.
Definition: ass_split.c:357
ASSDialog * ff_ass_split_dialog(ASSSplitContext *ctx, const char *buf, int cache, int *number)
Split one or several ASS "Dialogue" lines from a string buffer and store them in a already initialize...
Definition: ass_split.c:338
int ff_ass_split_override_codes(const ASSCodesCallbacks *callbacks, void *priv, const char *buf)
Split override codes out of a ASS "Dialogue" Text field.
Definition: ass_split.c:370
void(* alpha)(void *priv, int alpha, int alpha_id)
Definition: ass_split.h:128
void(* text)(void *priv, const char *text, int len)
Definition: ass_split.h:124
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip please avoid problems through this extra level of scrutiny For cosmetics only commits you should e g by running git config global user name My Name git config global user email my email which is either set in your personal configuration file through git config core editor or set by one of the following environment VISUAL or EDITOR Log messages should be concise but descriptive Explain why you made a what you did will be obvious from the changes themselves most of the time Saying just bug fix or is bad Remember that people of varying skill levels look at and educate themselves while reading through your code Don t include filenames in log Git provides that information Possibly make the commit message have a descriptive first an empty line and then a full description The first line will be used to name the patch by git format patch Renaming moving copying files or contents of making those normal commits mv cp path file otherpath otherfile git add[-A] git commit Do not move
Definition: git-howto.txt:153
Generated on Sat Aug 9 2025 06:52:47 for FFmpeg by
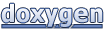