FFmpeg
|
overlay one video on top of another More...
#include "avfilter.h"
#include "formats.h"
#include "libavutil/common.h"
#include "libavutil/eval.h"
#include "libavutil/avstring.h"
#include "libavutil/opt.h"
#include "libavutil/pixdesc.h"
#include "libavutil/imgutils.h"
#include "libavutil/mathematics.h"
#include "libavutil/timestamp.h"
#include "internal.h"
#include "bufferqueue.h"
#include "drawutils.h"
#include "video.h"
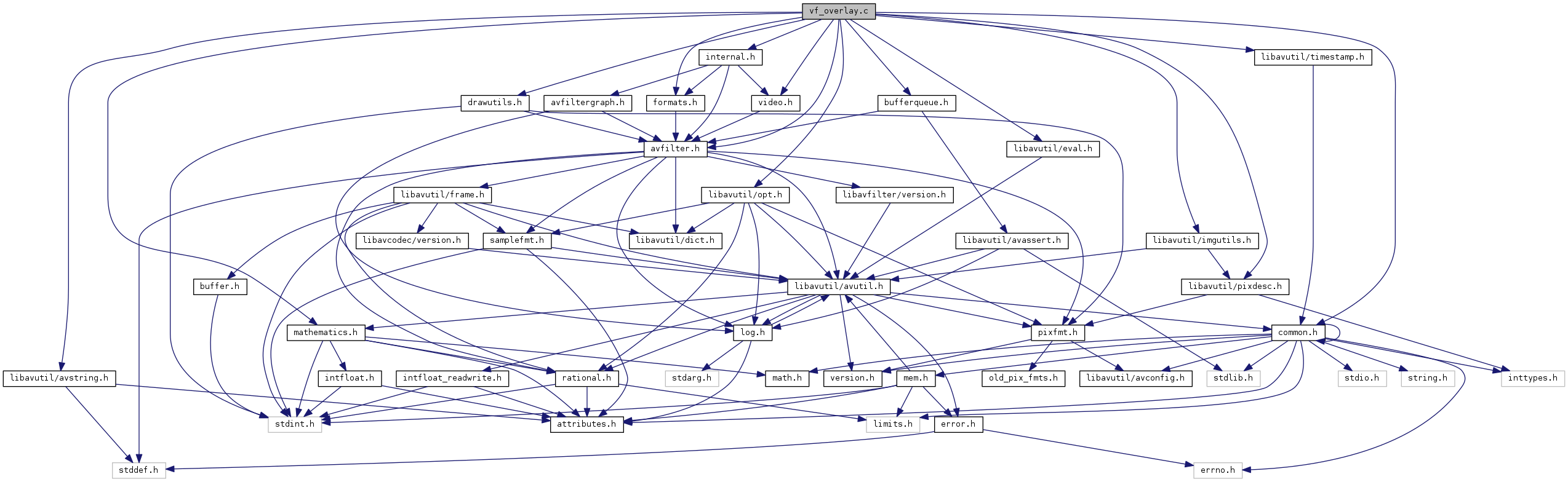
Go to the source code of this file.
Data Structures | |
struct | OverlayContext |
Macros | |
#define | MAIN 0 |
#define | OVERLAY 1 |
#define | R 0 |
#define | G 1 |
#define | B 2 |
#define | A 3 |
#define | Y 0 |
#define | U 1 |
#define | V 2 |
#define | FAST_DIV255(x) ((((x) + 128) * 257) >> 16) |
#define | UNPREMULTIPLY_ALPHA(x, y) ((((x) << 16) - ((x) << 9) + (x)) / ((((x) + (y)) << 8) - ((x) + (y)) - (y) * (x))) |
#define | OFFSET(x) offsetof(OverlayContext, x) |
#define | FLAGS AV_OPT_FLAG_VIDEO_PARAM|AV_OPT_FLAG_FILTERING_PARAM |
Functions | |
static av_cold int | init (AVFilterContext *ctx) |
static av_cold void | uninit (AVFilterContext *ctx) |
static int | normalize_xy (double d, int chroma_sub) |
static void | eval_expr (AVFilterContext *ctx, enum EvalTarget eval_tgt) |
static int | set_expr (AVExpr **pexpr, const char *expr, const char *option, void *log_ctx) |
static int | process_command (AVFilterContext *ctx, const char *cmd, const char *args, char *res, int res_len, int flags) |
static int | query_formats (AVFilterContext *ctx) |
static int | config_input_main (AVFilterLink *inlink) |
static int | config_input_overlay (AVFilterLink *inlink) |
static int | config_output (AVFilterLink *outlink) |
static void | blend_image (AVFilterContext *ctx, AVFrame *dst, AVFrame *src, int x, int y) |
Blend image in src to destination buffer dst at position (x, y). More... | |
static int | try_filter_frame (AVFilterContext *ctx, AVFrame *mainpic) |
static int | try_filter_next_frame (AVFilterContext *ctx) |
static int | flush_frames (AVFilterContext *ctx) |
static int | filter_frame_main (AVFilterLink *inlink, AVFrame *inpicref) |
static int | filter_frame_over (AVFilterLink *inlink, AVFrame *inpicref) |
static int | request_frame (AVFilterLink *outlink) |
AVFILTER_DEFINE_CLASS (overlay) | |
Variables | |
static const char *const | var_names [] |
static enum AVPixelFormat | alpha_pix_fmts [] |
static const AVOption | overlay_options [] |
static const AVFilterPad | avfilter_vf_overlay_inputs [] |
static const AVFilterPad | avfilter_vf_overlay_outputs [] |
AVFilter | avfilter_vf_overlay |
Detailed Description
overlay one video on top of another
Definition in file vf_overlay.c.
Macro Definition Documentation
#define A 3 |
Definition at line 82 of file vf_overlay.c.
Referenced by blend_image().
#define B 2 |
Definition at line 81 of file vf_overlay.c.
Referenced by blend_image().
Definition at line 361 of file vf_overlay.c.
Referenced by blend_image().
#define FLAGS AV_OPT_FLAG_VIDEO_PARAM|AV_OPT_FLAG_FILTERING_PARAM |
Definition at line 712 of file vf_overlay.c.
#define G 1 |
Definition at line 80 of file vf_overlay.c.
Referenced by blend_image().
#define MAIN 0 |
Definition at line 76 of file vf_overlay.c.
Referenced by config_input_overlay(), config_output(), Faac_encode_init(), query_formats(), request_frame(), and try_filter_frame().
#define OFFSET | ( | x | ) | offsetof(OverlayContext, x) |
Definition at line 711 of file vf_overlay.c.
#define OVERLAY 1 |
Definition at line 77 of file vf_overlay.c.
Referenced by avfilter_register_all(), config_input_overlay(), query_formats(), request_frame(), and try_filter_frame().
#define R 0 |
Definition at line 79 of file vf_overlay.c.
Referenced by blend_image().
#define U 1 |
Definition at line 85 of file vf_overlay.c.
#define UNPREMULTIPLY_ALPHA | ( | x, | |
y | |||
) | ((((x) << 16) - ((x) << 9) + (x)) / ((((x) + (y)) << 8) - ((x) + (y)) - (y) * (x))) |
Definition at line 367 of file vf_overlay.c.
Referenced by blend_image().
#define V 2 |
Definition at line 86 of file vf_overlay.c.
#define Y 0 |
Definition at line 84 of file vf_overlay.c.
Enumeration Type Documentation
enum EvalTarget |
Enumerator | |
---|---|
EVAL_XY | |
EVAL_ENABLE | |
EVAL_ALL |
Definition at line 152 of file vf_overlay.c.
enum var_name |
Definition at line 61 of file vf_overlay.c.
Function Documentation
AVFILTER_DEFINE_CLASS | ( | overlay | ) |
|
static |
Blend image in src to destination buffer dst at position (x, y).
< the amount of overlay to blend on to main
< the amount of overlay to blend on to main
Definition at line 372 of file vf_overlay.c.
Referenced by try_filter_frame().
|
static |
Definition at line 283 of file vf_overlay.c.
|
static |
Definition at line 299 of file vf_overlay.c.
|
static |
Definition at line 348 of file vf_overlay.c.
|
static |
Definition at line 154 of file vf_overlay.c.
Referenced by config_input_overlay(), process_command(), and try_filter_frame().
|
static |
Definition at line 647 of file vf_overlay.c.
|
static |
Definition at line 668 of file vf_overlay.c.
|
static |
Definition at line 639 of file vf_overlay.c.
Referenced by filter_frame_main(), and filter_frame_over().
|
static |
Definition at line 121 of file vf_overlay.c.
|
inlinestatic |
Definition at line 145 of file vf_overlay.c.
Referenced by eval_expr().
|
static |
Definition at line 191 of file vf_overlay.c.
|
static |
Definition at line 219 of file vf_overlay.c.
|
static |
Definition at line 681 of file vf_overlay.c.
|
static |
Definition at line 170 of file vf_overlay.c.
Referenced by config_input_overlay(), and process_command().
|
static |
Definition at line 560 of file vf_overlay.c.
Referenced by filter_frame_main(), and try_filter_next_frame().
|
static |
Definition at line 625 of file vf_overlay.c.
Referenced by filter_frame_over(), flush_frames(), and request_frame().
|
static |
Definition at line 133 of file vf_overlay.c.
Variable Documentation
|
static |
Definition at line 277 of file vf_overlay.c.
Referenced by config_input_main(), and config_input_overlay().
AVFilter avfilter_vf_overlay |
Definition at line 761 of file vf_overlay.c.
|
static |
Definition at line 733 of file vf_overlay.c.
|
static |
Definition at line 751 of file vf_overlay.c.
|
static |
Definition at line 714 of file vf_overlay.c.
|
static |
Definition at line 46 of file vf_overlay.c.
Referenced by set_expr().
Generated on Wed May 8 2024 06:52:21 for FFmpeg by
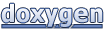