FFmpeg
|
bitstream api. More...
#include "libavutil/avassert.h"
#include "avcodec.h"
#include "mathops.h"
#include "get_bits.h"
#include "put_bits.h"
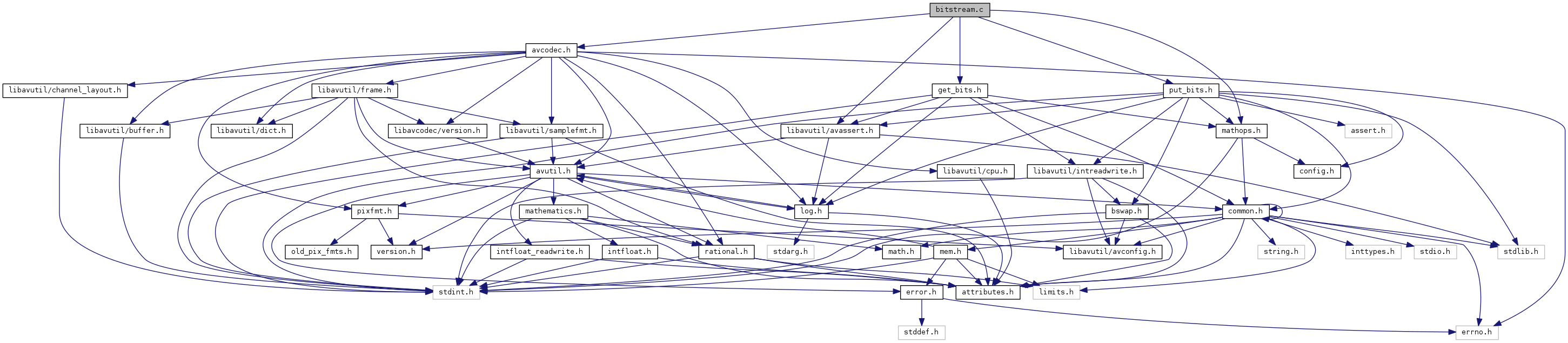
Go to the source code of this file.
Data Structures | |
struct | VLCcode |
Macros | |
#define | GET_DATA(v, table, i, wrap, size) |
#define | COPY(condition) |
Functions | |
void | avpriv_align_put_bits (PutBitContext *s) |
Pad the bitstream with zeros up to the next byte boundary. More... | |
void | avpriv_put_string (PutBitContext *pb, const char *string, int terminate_string) |
Put the string string in the bitstream. More... | |
void | avpriv_copy_bits (PutBitContext *pb, const uint8_t *src, int length) |
Copy the content of src to the bitstream. More... | |
static int | alloc_table (VLC *vlc, int size, int use_static) |
static av_always_inline uint32_t | bitswap_32 (uint32_t x) |
static int | compare_vlcspec (const void *a, const void *b) |
static int | build_table (VLC *vlc, int table_nb_bits, int nb_codes, VLCcode *codes, int flags) |
Build VLC decoding tables suitable for use with get_vlc(). More... | |
int | ff_init_vlc_sparse (VLC *vlc, int nb_bits, int nb_codes, const void *bits, int bits_wrap, int bits_size, const void *codes, int codes_wrap, int codes_size, const void *symbols, int symbols_wrap, int symbols_size, int flags) |
void | ff_free_vlc (VLC *vlc) |
Variables | |
const uint8_t | ff_log2_run [41] |
Detailed Description
bitstream api.
Definition in file bitstream.c.
Macro Definition Documentation
#define COPY | ( | condition | ) |
Referenced by avfilter_register_all(), and ff_init_vlc_sparse().
Function Documentation
|
static |
Definition at line 101 of file bitstream.c.
Referenced by build_table(), and mp_decode_layer2().
void avpriv_align_put_bits | ( | PutBitContext * | s | ) |
Pad the bitstream with zeros up to the next byte boundary.
Definition at line 46 of file bitstream.c.
Referenced by avpriv_copy_pce_data(), encode_block(), encode_frame(), encode_picture_ls(), ff_flv_encode_picture_header(), ff_h261_encode_picture_header(), ff_h263_encode_picture_header(), ff_msmpeg4_encode_picture_header(), ff_rv10_encode_picture_header(), flush_put_bits(), latm_write_packet(), put_bitstream_info(), put_header(), write_slice_end(), xsub_encode(), and xsub_encode_rle().
void avpriv_copy_bits | ( | PutBitContext * | pb, |
const uint8_t * | src, | ||
int | length | ||
) |
Copy the content of src to the bitstream.
- Parameters
-
length the number of bits of src to copy
Definition at line 61 of file bitstream.c.
Referenced by copy_bits(), encode_thread(), ff_mpeg4_merge_partitions(), flush_put_bits(), latm_write_frame_header(), latm_write_packet(), merge_context_after_encode(), save_bits(), and svq1_encode_plane().
void avpriv_put_string | ( | PutBitContext * | pb, |
const char * | string, | ||
int | terminate_string | ||
) |
Put the string string in the bitstream.
- Parameters
-
terminate_string 0-terminates the written string if value is 1
Definition at line 51 of file bitstream.c.
Referenced by flush_put_bits(), jpeg_put_comments(), and mpeg4_encode_vol_header().
|
static |
Definition at line 118 of file bitstream.c.
Referenced by build_table().
|
static |
Build VLC decoding tables suitable for use with get_vlc().
- Parameters
-
vlc the context to be initted table_nb_bits max length of vlc codes to store directly in this table (Longer codes are delegated to subtables.) nb_codes number of elements in codes[] codes descriptions of the vlc codes These must be ordered such that codes going into the same subtable are contiguous. Sorting by VLCcode.code is sufficient, though not necessary.
Definition at line 153 of file bitstream.c.
Referenced by ff_init_vlc_sparse().
Definition at line 133 of file bitstream.c.
Referenced by ff_init_vlc_sparse().
Definition at line 344 of file bitstream.c.
Referenced by cook_decode_close(), decode_argb_frame(), decode_close(), decode_end(), decode_plane(), decode_rgb24_frame(), decode_rle(), dnxhd_decode_close(), dnxhd_init_vlc(), ff_dvvideo_init(), ff_init_vlc_sparse(), ff_ivi_dec_huff_desc(), ff_ivi_decode_close(), ff_mjpeg_decode_dht(), ff_mjpeg_decode_end(), ff_wma_end(), fraps2_decode_plane(), free_vlcs(), generate_joint_tables(), ivi_free_buffers(), mimic_decode_end(), mp_decode_frame(), mss4_free_vlcs(), read_huffman_tables(), read_old_huffman_tables(), smacker_decode_header_tree(), smka_decode_frame(), tm2_free_codes(), vorbis_free(), vp3_decode_end(), vp6_build_huff_tree(), and vp6_decode_free_context().
int ff_init_vlc_sparse | ( | VLC * | vlc, |
int | nb_bits, | ||
int | nb_codes, | ||
const void * | bits, | ||
int | bits_wrap, | ||
int | bits_size, | ||
const void * | codes, | ||
int | codes_wrap, | ||
int | codes_size, | ||
const void * | symbols, | ||
int | symbols_wrap, | ||
int | symbols_size, | ||
int | flags | ||
) |
Definition at line 262 of file bitstream.c.
Referenced by build_huff(), build_huff_tree(), build_vlc(), ff_ccitt_unpack_init(), ff_init_vlc_sparse(), generate_joint_tables(), init_ralf_vlc(), init_vlcs(), mpc8_decode_init(), mss4_init_vlc(), read_code_table(), rv34_gen_vlc(), and rv40_init_tables().
Variable Documentation
const uint8_t ff_log2_run[41] |
Definition at line 37 of file bitstream.c.
Referenced by decode_line(), encode_line(), ls_decode_line(), ls_encode_line(), and ls_encode_run().
Generated on Mon Nov 18 2024 06:52:05 for FFmpeg by
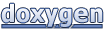