FFmpeg
|
wmaenc.c
Go to the documentation of this file.
161 static int encode_block(WMACodecContext *s, float (*src_coefs)[BLOCK_MAX_SIZE], int total_gain){
165 static const int fixed_exp[25]={20,20,20,20,20,20,20,20,20,20,20,20,20,20,20,20,20,20,20,20,20,20,20,20,20};
326 static int encode_frame(WMACodecContext *s, float (*src_coefs)[BLOCK_MAX_SIZE], uint8_t *buf, int buf_size, int total_gain){
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
static int encode_superframe(AVCodecContext *avctx, AVPacket *avpkt, const AVFrame *frame, int *got_packet_ptr)
Definition: wmaenc.c:341
void avpriv_align_put_bits(PutBitContext *s)
Pad the bitstream with zeros up to the next byte boundary.
Definition: bitstream.c:46
int block_align
number of bytes per packet if constant and known or 0 Used by some WAV based audio codecs...
Definition: libavcodec/avcodec.h:1898
Definition: samplefmt.h:50
#define av_assert2(cond)
assert() equivalent, that does lie in speed critical code.
Definition: avassert.h:63
float WMACoef
type for decoded coefficients, int16_t would be enough for wma 1/2
Definition: wma.h:56
void(* vector_fmul)(float *dst, const float *src0, const float *src1, int len)
Calculate the product of two vectors of floats and store the result in a vector of floats...
Definition: float_dsp.h:38
int64_t pts
Presentation timestamp in time_base units (time when frame should be shown to user).
Definition: frame.h:159
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
static int encode_frame(WMACodecContext *s, float(*src_coefs)[BLOCK_MAX_SIZE], uint8_t *buf, int buf_size, int total_gain)
Definition: wmaenc.c:326
static uint8_t * put_bits_ptr(PutBitContext *s)
Return the pointer to the byte where the bitstream writer will put the next bit.
Definition: put_bits.h:199
static void encode_exp_vlc(WMACodecContext *s, int ch, const int *exp_param)
Definition: wmaenc.c:135
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
static void apply_window_and_mdct(AVCodecContext *avctx, const AVFrame *frame)
Definition: wmaenc.c:92
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
static void put_bits(J2kEncoderContext *s, int val, int n)
put n times val bit
Definition: j2kenc.c:160
external API header
int exponent_high_bands[BLOCK_NB_SIZES][HIGH_BAND_MAX_SIZE]
Definition: wma.h:83
Definition: fft.h:62
#define av_assert1(cond)
assert() equivalent, that does not lie in speed critical code.
Definition: avassert.h:53
Definition: libavcodec/avcodec.h:388
int ff_alloc_packet2(AVCodecContext *avctx, AVPacket *avpkt, int size)
Check AVPacket size and/or allocate data.
Definition: libavcodec/utils.c:1377
static void init_exp(WMACodecContext *s, int ch, const int *exp_param)
Definition: wmaenc.c:114
int frame_size
Number of samples per channel in an audio frame.
Definition: libavcodec/avcodec.h:1881
1i.*Xphase exp()
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
void(* vector_fmul_scalar)(float *dst, const float *src, float mul, int len)
Multiply a vector of floats by a scalar float.
Definition: float_dsp.h:69
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
static int encode_block(WMACodecContext *s, float(*src_coefs)[BLOCK_MAX_SIZE], int total_gain)
Definition: wmaenc.c:161
struct WMACodecContext WMACodecContext
common internal api header.
static void flush_put_bits(PutBitContext *s)
Pad the end of the output stream with zeros.
Definition: put_bits.h:81
static void init_put_bits(PutBitContext *s, uint8_t *buffer, int buffer_size)
Initialize the PutBitContext s.
Definition: put_bits.h:54
Definition: libavcodec/avcodec.h:389
Definition: wma.h:66
static av_always_inline int64_t ff_samples_to_time_base(AVCodecContext *avctx, int64_t samples)
Rescale from sample rate to AVCodecContext.time_base.
Definition: libavcodec/internal.h:186
int high_band_coded[MAX_CHANNELS][HIGH_BAND_MAX_SIZE]
Definition: wma.h:87
int64_t pts
Presentation timestamp in AVStream->time_base units; the time at which the decompressed packet will b...
Definition: libavcodec/avcodec.h:1044
void(* vector_fmul_reverse)(float *dst, const float *src0, const float *src1, int len)
Calculate the product of two vectors of floats, and store the result in a vector of floats...
Definition: float_dsp.h:140
Generated on Tue Jan 21 2025 06:52:30 for FFmpeg by
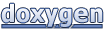