FFmpeg
|
wma.h
Go to the documentation of this file.
93 uint16_t *run_table[2];
95 uint16_t *int_table[2];
122 uint8_t last_superframe[MAX_CODED_SUPERFRAME_SIZE + FF_INPUT_BUFFER_PADDING_SIZE]; /* padding added */
Definition: put_bits.h:41
unsigned int ff_wma_get_large_val(GetBitContext *gb)
Decode an uncompressed coefficient.
Definition: wma.c:401
int ff_wma_run_level_decode(AVCodecContext *avctx, GetBitContext *gb, VLC *vlc, const float *level_table, const uint16_t *run_table, int version, WMACoef *ptr, int offset, int num_coefs, int block_len, int frame_len_bits, int coef_nb_bits)
Decode run level compressed coefficients.
Definition: wma.c:434
Definition: wma.h:58
float WMACoef
type for decoded coefficients, int16_t would be enough for wma 1/2
Definition: wma.h:56
Definition: fmtconvert.h:28
bitstream reader API header.
Definition: get_bits.h:63
#define FF_INPUT_BUFFER_PADDING_SIZE
Required number of additionally allocated bytes at the end of the input bitstream for decoding...
Definition: libavcodec/avcodec.h:561
Definition: fft.h:62
struct CoefVLCTable CoefVLCTable
Definition: float_dsp.h:24
static const int8_t noise_table[256]
Data table used for TrueHD noise generation function.
Definition: mlpdec.c:892
struct WMACodecContext WMACodecContext
Definition: get_bits.h:54
these buffered frames must be flushed immediately if a new input produces new output(Example:frame rate-doubling filter:filter_frame must(1) flush the second copy of the previous frame, if it is still there,(2) push the first copy of the incoming frame,(3) keep the second copy for later.) If the input frame is not enough to produce output
Definition: wma.h:66
bitstream writer API
Generated on Wed May 7 2025 06:52:49 for FFmpeg by
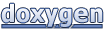