FFmpeg
|
mpeg4videoenc.c
Go to the documentation of this file.
60 * @param[in] block_last_index last index in scantable order that refers to a non zero element in block.
62 static inline int get_block_rate(MpegEncContext * s, int16_t block[64], int block_last_index, uint8_t scantable[64]){
94 static inline void restore_ac_coeffs(MpegEncContext * s, int16_t block[6][64], const int dir[6], uint8_t *st[6], const int zigzag_last_index[6])
123 * @param[out] zigzag_last_index index referring to the last non zero coefficient in zigzag order
125 static inline int decide_ac_pred(MpegEncContext * s, int16_t block[6][64], const int dir[6], uint8_t *st[6], int zigzag_last_index[6])
156 block[n][s->dsp.idct_permutation[i ]] = level - ROUNDED_DIV(ac_val[i + 8]*qscale_table[xy], s->qscale);
178 block[n][s->dsp.idct_permutation[i<<3]]= level - ROUNDED_DIV(ac_val[i]*qscale_table[xy], s->qscale);
212 /* ok, come on, this isn't funny anymore, there's more code for handling this mpeg4 mess than for the actual adaptive quantization */
232 if(qscale_table[mb_xy] != qscale_table[s->mb_index2xy[i-1]] && (s->mb_type[mb_xy]&CANDIDATE_MB_TYPE_DIRECT)){
330 put_bits(ac_pb, 7+2+1+6+1+12+1, (3<<23)+(3<<21)+(0<<20)+(run<<14)+(1<<13)+(((level-64)&0xfff)<<1)+1);
343 put_bits(ac_pb, 7+2+1+6+1+12+1, (3<<23)+(3<<21)+(1<<20)+(run<<14)+(1<<13)+(((level-64)&0xfff)<<1)+1);
399 static inline void mpeg4_encode_blocks(MpegEncContext * s, int16_t block[6][64], int intra_dc[6],
417 skip_put_bits(&s->pb, mpeg4_get_block_length(s, block[i], i, 0, s->intra_scantable.permutated));
477 PutBitContext * const tex_pb = s->data_partitioning && s->pict_type!=AV_PICTURE_TYPE_B ? &s->tex_pb : &s->pb;
478 PutBitContext * const dc_pb = s->data_partitioning && s->pict_type!=AV_PICTURE_TYPE_I ? &s->pb2 : &s->pb;
762 ff_h263_encode_motion_vector(s, s->current_picture.motion_val[0][ s->block_index[i] ][0] - pred_x,
1052 if(s->strict_std_compliance < FF_COMPLIANCE_VERY_STRICT || picture_number==0) //HACK, the reference sw is buggy
1338 { "data_partitioning", "Use data partitioning.", OFFSET(data_partitioning), AV_OPT_TYPE_INT, { .i64 = 0 }, 0, 1, VE },
1339 { "alternate_scan", "Enable alternate scantable.", OFFSET(alternate_scan), AV_OPT_TYPE_INT, { .i64 = 0 }, 0, 1, VE },
static void mpeg4_encode_visual_object_header(MpegEncContext *s)
Definition: mpeg4videoenc.c:894
Definition: start.py:1
int time_increment_bits
number of bits to represent the fractional part of time
Definition: mpegvideo.h:555
Definition: pixfmt.h:67
static uint8_t uni_mpeg4_intra_rl_len[64 *64 *2 *2]
Definition: mpeg4videoenc.c:40
static void mpeg4_encode_block(MpegEncContext *s, int16_t *block, int n, int intra_dc, uint8_t *scan_table, PutBitContext *dc_pb, PutBitContext *ac_pb)
Encode an 8x8 block.
Definition: mpeg4videoenc.c:297
Definition: put_bits.h:41
int last_mv[2][2][2]
last MV, used for MV prediction in MPEG1 & B-frame MPEG4
Definition: mpegvideo.h:433
void ff_clean_mpeg4_qscales(MpegEncContext *s)
modify mb_type & qscale so that encoding is actually possible in mpeg4
Definition: mpeg4videoenc.c:204
#define CODEC_FLAG_PASS1
Use internal 2pass ratecontrol in first pass mode.
Definition: libavcodec/avcodec.h:704
Definition: opt.h:222
int16_t(*[3] ac_val)[16]
used for for mpeg4 AC prediction, all 3 arrays must be continuous
Definition: mpegvideo.h:357
static int get_block_rate(MpegEncContext *s, int16_t block[64], int block_last_index, uint8_t scantable[64])
Return the number of bits that encoding the 8x8 block in block would need.
Definition: mpeg4videoenc.c:62
static void skip_put_bits(PutBitContext *s, int n)
Skip the given number of bits.
Definition: put_bits.h:220
void ff_init_rl(RLTable *rl, uint8_t static_store[2][2 *MAX_RUN+MAX_LEVEL+3])
Definition: mpegvideo.c:1304
void avpriv_copy_bits(PutBitContext *pb, const uint8_t *src, int length)
Copy the content of src to the bitstream.
Definition: bitstream.c:61
AVRational sample_aspect_ratio
sample aspect ratio (0 if unknown) That is the width of a pixel divided by the height of the pixel...
Definition: libavcodec/avcodec.h:1505
Definition: libavcodec/avcodec.h:115
mpegvideo header.
static uint32_t uni_mpeg4_intra_rl_bits[64 *64 *2 *2]
Definition: mpeg4videoenc.c:39
int16_t * ff_h263_pred_motion(MpegEncContext *s, int block, int dir, int *px, int *py)
Definition: h263.c:315
void ff_mpeg4_encode_picture_header(MpegEncContext *s, int picture_number)
Definition: mpeg4videoenc.c:1043
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
void ff_mpeg4_stuffing(PutBitContext *pbc)
add mpeg4 stuffing bits (01...1)
Definition: mpeg4videoenc.c:847
const char * class_name
The name of the class; usually it is the same name as the context structure type to which the AVClass...
Definition: log.h:55
#define MV_DIRECT
bidirectional mode where the difference equals the MV of the last P/S/I-Frame (mpeg4) ...
Definition: mpegvideo.h:419
#define av_assert2(cond)
assert() equivalent, that does lie in speed critical code.
Definition: avassert.h:63
AVOptions.
#define CODEC_FLAG_GLOBAL_HEADER
Place global headers in extradata instead of every keyframe.
Definition: libavcodec/avcodec.h:714
#define FF_COMPLIANCE_VERY_STRICT
Strictly conform to an older more strict version of the spec or reference software.
Definition: libavcodec/avcodec.h:2405
static int decide_ac_pred(MpegEncContext *s, int16_t block[6][64], const int dir[6], uint8_t *st[6], int zigzag_last_index[6])
Return the optimal value (0 or 1) for the ac_pred element for the given MB in mpeg4.
Definition: mpeg4videoenc.c:125
int64_t pts
Presentation timestamp in time_base units (time when frame should be shown to user).
Definition: frame.h:159
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
int no_rounding
apply no rounding to motion compensation (MPEG4, msmpeg4, ...) for b-frames rounding mode is always 0...
Definition: mpegvideo.h:439
#define CODEC_FLAG_BITEXACT
Use only bitexact stuff (except (I)DCT).
Definition: libavcodec/avcodec.h:715
#define CODEC_FLAG_AC_PRED
H.263 advanced intra coding / MPEG-4 AC prediction.
Definition: libavcodec/avcodec.h:717
static uint32_t uni_mpeg4_inter_rl_bits[64 *64 *2 *2]
Definition: mpeg4videoenc.c:41
static int ff_mpeg4_pred_dc(MpegEncContext *s, int n, int level, int *dir_ptr, int encoding)
Predict the dc.
Definition: mpeg4video.h:129
static void mpeg4_encode_gop_header(MpegEncContext *s)
Definition: mpeg4videoenc.c:865
void ff_mpeg4_init_partitions(MpegEncContext *s)
Definition: mpeg4videoenc.c:1283
static uint8_t * put_bits_ptr(PutBitContext *s)
Return the pointer to the byte where the bitstream writer will put the next bit.
Definition: put_bits.h:199
static void restore_ac_coeffs(MpegEncContext *s, int16_t block[6][64], const int dir[6], uint8_t *st[6], const int zigzag_last_index[6])
Restore the ac coefficients in block that have been changed by decide_ac_pred().
Definition: mpeg4videoenc.c:94
uint8_t * inter_ac_vlc_last_length
Definition: mpegvideo.h:485
void ff_mpeg4_merge_partitions(MpegEncContext *s)
Definition: mpeg4videoenc.c:1296
#define CODEC_CAP_DELAY
Encoder or decoder requires flushing with NULL input at the end in order to give the complete and cor...
Definition: libavcodec/avcodec.h:770
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
void ff_mpeg4_encode_mb(MpegEncContext *s, int16_t block[6][64], int motion_x, int motion_y)
Definition: mpeg4videoenc.c:471
av_const int ff_h263_aspect_to_info(AVRational aspect)
Return the 4 bit value that specifies the given aspect ratio.
Definition: ituh263enc.c:87
static void put_bits(J2kEncoderContext *s, int val, int n)
put n times val bit
Definition: j2kenc.c:160
void ff_clean_h263_qscales(MpegEncContext *s)
modify qscale so that encoding is actually possible in h263 (limit difference to -2..2)
Definition: ituh263enc.c:272
int av_reduce(int *dst_num, int *dst_den, int64_t num, int64_t den, int64_t max)
Reduce a fraction.
Definition: rational.c:36
uint8_t * intra_ac_vlc_last_length
Definition: mpegvideo.h:483
uint8_t ff_mpeg4_static_rl_table_store[3][2][2 *MAX_RUN+MAX_LEVEL+3]
Definition: mpeg4video.c:27
static uint8_t uni_mpeg4_inter_rl_len[64 *64 *2 *2]
Definition: mpeg4videoenc.c:42
static int get_b_cbp(MpegEncContext *s, int16_t block[6][64], int motion_x, int motion_y, int mb_type)
Definition: mpeg4videoenc.c:428
static void set_put_bits_buffer_size(PutBitContext *s, int size)
Change the end of the buffer.
Definition: put_bits.h:232
void ff_write_quant_matrix(PutBitContext *pb, uint16_t *matrix)
Definition: mpegvideo_enc.c:161
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
static void init_uni_mpeg4_rl_tab(RLTable *rl, uint32_t *bits_tab, uint8_t *len_tab)
Definition: mpeg4videoenc.c:1150
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
static int mpeg4_get_block_length(MpegEncContext *s, int16_t *block, int n, int intra_dc, uint8_t *scan_table)
Definition: mpeg4videoenc.c:348
static void ff_h263_encode_motion_vector(MpegEncContext *s, int x, int y, int f_code)
Definition: h263.h:137
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
int pict_type
AV_PICTURE_TYPE_I, AV_PICTURE_TYPE_P, AV_PICTURE_TYPE_B, ...
Definition: mpegvideo.h:377
int ff_mpeg4_get_video_packet_prefix_length(MpegEncContext *s)
Definition: mpeg4video.c:29
int mv[2][4][2]
motion vectors for a macroblock first coordinate : 0 = forward 1 = backward second " : depend...
Definition: mpegvideo.h:431
planar YUV 4:2:0, 12bpp, (1 Cr & Cb sample per 2x2 Y samples)
Definition: pixfmt.h:68
#define CODEC_CAP_SLICE_THREADS
Codec supports slice-based (or partition-based) multithreading.
Definition: libavcodec/avcodec.h:814
int mb_stride
mb_width+1 used for some arrays to allow simple addressing of left & top MBs without sig11 ...
Definition: mpegvideo.h:278
static void flush_put_bits(PutBitContext *s)
Pad the end of the output stream with zeros.
Definition: put_bits.h:81
static void init_put_bits(PutBitContext *s, uint8_t *buffer, int buffer_size)
Initialize the PutBitContext s.
Definition: put_bits.h:54
static int get_rl_index(const RLTable *rl, int last, int run, int level)
Definition: rl.h:75
void ff_mpeg4_encode_video_packet_header(MpegEncContext *s)
Definition: mpeg4videoenc.c:1323
int top_field_first
If the content is interlaced, is top field displayed first.
Definition: frame.h:275
static int get_p_cbp(MpegEncContext *s, int16_t block[6][64], int motion_x, int motion_y)
Definition: h263.h:148
Picture ** reordered_input_picture
pointer to the next pictures in codedorder for encoding
Definition: mpegvideo.h:287
void avpriv_put_string(PutBitContext *pb, const char *string, int terminate_string)
Put the string string in the bitstream.
Definition: bitstream.c:51
int workaround_bugs
workaround bugs in encoders which cannot be detected automatically
Definition: mpegvideo.h:266
Definition: avutil.h:143
static void mpeg4_encode_blocks(MpegEncContext *s, int16_t block[6][64], int intra_dc[6], uint8_t **scan_table, PutBitContext *dc_pb, PutBitContext *ac_pb)
Definition: mpeg4videoenc.c:399
static void mpeg4_encode_dc(PutBitContext *s, int level, int n)
Encode the dc value.
Definition: mpeg4videoenc.c:244
static void mpeg4_encode_vol_header(MpegEncContext *s, int vo_number, int vol_number)
Definition: mpeg4videoenc.c:939
int ff_MPV_encode_picture(AVCodecContext *avctx, AVPacket *pkt, AVFrame *frame, int *got_packet)
Definition: mpegvideo_enc.c:1443
#define FF_BUG_MS
Work around various bugs in Microsoft's broken decoders.
Definition: libavcodec/avcodec.h:2389
Generated on Thu Jun 19 2025 06:53:10 for FFmpeg by
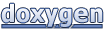