FFmpeg
|
jpeglsenc.c
Go to the documentation of this file.
66 static inline void ls_encode_runterm(JLSState *state, PutBitContext *pb, int RItype, int err, int limit_add){
96 static inline void ls_encode_run(JLSState *state, PutBitContext *pb, int run, int comp, int trail){
116 static inline void ls_encode_line(JLSState *state, PutBitContext *pb, void *last, void *cur, int last2, int w, int stride, int comp, int bits){
179 context = ff_jpegls_quantize(state, D0) * 81 + ff_jpegls_quantize(state, D1) * 9 + ff_jpegls_quantize(state, D2);
217 if(state->T1 == state2.T1 && state->T2 == state2.T2 && state->T3 == state2.T3 && state->reset == state2.reset)
390 if(ctx->pix_fmt != AV_PIX_FMT_GRAY8 && ctx->pix_fmt != AV_PIX_FMT_GRAY16 && ctx->pix_fmt != AV_PIX_FMT_RGB24 && ctx->pix_fmt != AV_PIX_FMT_BGR24){
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
Definition: pixfmt.h:67
Definition: put_bits.h:41
static void ls_encode_regular(JLSState *state, PutBitContext *pb, int Q, int err)
Encode error from regular symbol.
Definition: jpeglsenc.c:41
Definition: jpegls.h:34
void avpriv_align_put_bits(PutBitContext *s)
Pad the bitstream with zeros up to the next byte boundary.
Definition: bitstream.c:46
Definition: mjpeg.h:74
MJPEG encoder and decoder.
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
Definition: jpegls.h:39
static void ls_encode_line(JLSState *state, PutBitContext *pb, void *last, void *cur, int last2, int w, int stride, int comp, int bits)
Encode one line of image.
Definition: jpeglsenc.c:116
bitstream reader API header.
static void ls_encode_run(JLSState *state, PutBitContext *pb, int run, int comp, int trail)
Encode run value as specified by JPEG-LS standard.
Definition: jpeglsenc.c:96
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
void ff_jpegls_reset_coding_parameters(JLSState *s, int reset_all)
Calculate JPEG-LS codec values.
Definition: jpegls.c:57
static int ff_jpegls_update_state_regular(JLSState *state, int Q, int err)
Definition: jpegls.h:89
static int encode_picture_ls(AVCodecContext *avctx, AVPacket *pkt, const AVFrame *pict, int *got_packet)
Definition: jpeglsenc.c:230
static void put_bits(J2kEncoderContext *s, int val, int n)
put n times val bit
Definition: j2kenc.c:160
external API header
Definition: mjpeg.h:76
#define FF_MIN_BUFFER_SIZE
minimum encoding buffer size Used to avoid some checks during header writing.
Definition: libavcodec/avcodec.h:568
static void ls_encode_runterm(JLSState *state, PutBitContext *pb, int RItype, int err, int limit_add)
Encode error from run termination.
Definition: jpeglsenc.c:66
static void set_ur_golomb_jpegls(PutBitContext *pb, int i, int k, int limit, int esc_len)
write unsigned golomb rice code (jpegls).
Definition: golomb.h:509
int ff_alloc_packet2(AVCodecContext *avctx, AVPacket *avpkt, int size)
Check AVPacket size and/or allocate data.
Definition: libavcodec/utils.c:1377
Definition: mjpeg.h:75
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
JPEG-LS common code.
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
Definition: get_bits.h:54
common internal api header.
static void flush_put_bits(PutBitContext *s)
Pad the end of the output stream with zeros.
Definition: put_bits.h:81
static void init_put_bits(PutBitContext *s, uint8_t *buffer, int buffer_size)
Initialize the PutBitContext s.
Definition: put_bits.h:54
Definition: libavcodec/avcodec.h:114
static int ff_jpegls_quantize(JLSState *s, int v)
Calculate quantized gradient value, used for context determination.
Definition: jpegls.h:57
static void comp(unsigned char *dst, int dst_stride, unsigned char *src, int src_stride, int add)
Definition: eamad.c:71
Definition: avutil.h:143
void ff_jpegls_init_state(JLSState *state)
Calculate initial JPEG-LS parameters.
Definition: jpegls.c:30
exp golomb vlc stuff
static void ff_jpegls_downscale_state(JLSState *state, int Q)
Definition: jpegls.h:80
struct JpeglsContext JpeglsContext
bitstream writer API
Generated on Mon Nov 18 2024 06:51:56 for FFmpeg by
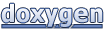