FFmpeg
|
h261enc.c
Go to the documentation of this file.
Definition: pixfmt.h:67
void ff_h261_encode_mb(MpegEncContext *s, int16_t block[6][64], int motion_x, int motion_y)
Definition: h261enc.c:228
void avpriv_align_put_bits(PutBitContext *s)
Pad the bitstream with zeros up to the next byte boundary.
Definition: bitstream.c:46
mpegvideo header.
void ff_h261_encode_picture_header(MpegEncContext *s, int picture_number)
Definition: h261enc.c:47
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
static void h261_encode_gob_header(MpegEncContext *s, int mb_line)
Encode a group of blocks header.
Definition: h261enc.c:85
static void ff_update_block_index(MpegEncContext *s)
Definition: mpegvideo.h:861
void ff_set_qscale(MpegEncContext *s, int qscale)
set qscale and update qscale dependent variables.
Definition: mpegvideo.c:3300
static void h261_encode_block(H261Context *h, int16_t *block, int n)
Encode an 8x8 block.
Definition: h261enc.c:160
Definition: libavcodec/avcodec.h:106
static uint8_t * put_bits_ptr(PutBitContext *s)
Return the pointer to the byte where the bitstream writer will put the next bit.
Definition: put_bits.h:199
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
simple assert() macros that are a bit more flexible than ISO C assert().
static void put_bits(J2kEncoderContext *s, int val, int n)
put n times val bit
Definition: j2kenc.c:160
external API header
#define av_assert1(cond)
assert() equivalent, that does not lie in speed critical code.
Definition: avassert.h:53
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample format(the sample packing is implied by the sample format) and sample rate.The lists are not just lists
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
int ff_h261_get_picture_format(int width, int height)
Definition: h261enc.c:34
h261codec.
planar YUV 4:2:0, 12bpp, (1 Cr & Cb sample per 2x2 Y samples)
Definition: pixfmt.h:68
static int get_rl_index(const RLTable *rl, int last, int run, int level)
Definition: rl.h:75
Definition: avutil.h:143
int ff_MPV_encode_picture(AVCodecContext *avctx, AVPacket *pkt, AVFrame *frame, int *got_packet)
Definition: mpegvideo_enc.c:1443
Generated on Tue Jan 21 2025 06:52:22 for FFmpeg by
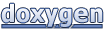