FFmpeg
|
vorbisdec.c
Go to the documentation of this file.
75 uint32_t map_size[2];
88 int16_t subclass_books[16][8];
103 int16_t books[64][8];
396 codebook_setup->codevectors[j * dim + k] = codebook_multiplicands[multiplicand_offset] * codebook_delta_value + codebook_minimum_value + last;
440 codebook_setup->maxdepth = (codebook_setup->maxdepth+codebook_setup->nb_bits - 1) / codebook_setup->nb_bits;
562 floor_setup->data.t1.x_list_dim+=floor_setup->data.t1.class_dimensions[floor_setup->data.t1.partition_class[j]];
581 for (k = 0; k < floor_setup->data.t1.class_dimensions[floor_setup->data.t1.partition_class[j]]; ++k, ++floor1_values) {
707 "partition out of bounds: type, begin, end, size, blocksize: %"PRIu16", %"PRIu32", %"PRIu32", %u, %"PRIu32"\n",
776 av_log(vc->avctx, AV_LOG_ERROR, "Other mappings than type 0 are not compliant with the Vorbis I specification. \n");
795 GET_VALIDATED_INDEX(mapping_setup->magnitude[j], ilog(vc->audio_channels - 1), vc->audio_channels)
907 av_log(vc->avctx, AV_LOG_ERROR, " Vorbis setup header packet corrupt (no vorbis signature). \n");
916 av_log(vc->avctx, AV_LOG_ERROR, " Vorbis setup header packet corrupt (time domain transforms). \n");
987 vc->channel_residues = av_malloc((vc->blocksize[1] / 2) * vc->audio_channels * sizeof(*vc->channel_residues));
997 av_dlog(NULL, " vorbis version %d \n audio_channels %d \n audio_samplerate %d \n bitrate_max %d \n bitrate_nom %d \n bitrate_min %d \n blk_0 %d blk_1 %d \n ",
998 vc->version, vc->audio_channels, vc->audio_samplerate, vc->bitrate_maximum, vc->bitrate_nominal, vc->bitrate_minimum, vc->blocksize[0], vc->blocksize[1]);
1003 vc->win[0][i] = sin(0.5*3.14159265358*(sin(((float)i + 0.5) / (float)BLK*3.14159265358))*(sin(((float)i + 0.5) / (float)BLK*3.14159265358)));
1034 if ((ret = avpriv_split_xiph_headers(headers, headers_len, 30, header_start, header_len)) < 0) {
1304 ff_vorbis_floor1_render_list(vf->list, vf->x_list_dim, floor1_Y_final, floor1_flag, vf->multiplier, vec, vf->list[1].x);
1368 classifs[j_times_ptns_to_read + partition_count + c_p_c - 1 - i] = temp - temp2 * vr->classifications;
1408 } else if (vr_type == 2 && ch == 2 && (voffset & 1) == 0 && (dim & 1) == 0) { // most frequent case optimized
1558 memset(ch_res_ptr, 0, sizeof(float) * vc->audio_channels * vlen); //FIXME can this be removed ?
1667 vc->fdsp.vector_fmul_window(ret + (bs1 - bs0) / 4, saved + (bs1 - bs0) / 4, buf, win, bs0 / 4);
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
unsigned int ff_vorbis_nth_root(unsigned int x, unsigned int n)
Definition: vorbis.c:39
static int vorbis_parse_setup_hdr_floors(vorbis_context *vc)
Definition: vorbisdec.c:493
Definition: vorbisdec.c:69
void ff_vorbis_inverse_coupling(float *mag, float *ang, intptr_t blocksize)
Definition: vorbisdec.c:1491
Definition: vorbisdec.c:50
int(* vorbis_floor_decode_func)(struct vorbis_context_s *, vorbis_floor_data *, float *)
Definition: vorbisdec.c:65
static av_cold int vorbis_decode_close(AVCodecContext *avctx)
Definition: vorbisdec.c:1768
uint8_t * book_list
Definition: vorbisdec.c:79
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
uint8_t class_subclasses[16]
Definition: vorbisdec.c:86
uint8_t multiplier
Definition: vorbisdec.c:89
static av_always_inline int vorbis_residue_decode_internal(vorbis_context *vc, vorbis_residue *vr, unsigned ch, uint8_t *do_not_decode, float *vec, unsigned vlen, unsigned ch_left, int vr_type)
Definition: vorbisdec.c:1313
FFmpeg currently uses a custom build this text attempts to document some of its obscure features and options Makefile the full command issued by make and its output will be shown on the screen DESTDIR Destination directory for the install useful to prepare packages or install FFmpeg in cross environments Makefile builds all the libraries and the executables fate Run the fate test note you must have installed it fate list Will list all fate regression test targets install Install headers
Definition: build_system.txt:1
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
Definition: vorbisdec.c:96
Definition: samplefmt.h:50
static int vorbis_floor0_decode(vorbis_context *vc, vorbis_floor_data *vfu, float *vec)
Definition: vorbisdec.c:1079
void(* vector_fmul)(float *dst, const float *src0, const float *src1, int len)
Calculate the product of two vectors of floats and store the result in a vector of floats...
Definition: float_dsp.h:38
Definition: fmtconvert.h:28
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
void(* vorbis_inverse_coupling)(float *mag, float *ang, intptr_t blocksize)
Definition: vorbisdsp.h:26
bitstream reader API header.
#define CODEC_FLAG_BITEXACT
Use only bitexact stuff (except (I)DCT).
Definition: libavcodec/avcodec.h:715
Definition: vorbisdsp.h:24
uint8_t amplitude_bits
Definition: vorbisdec.c:76
int16_t subclass_books[16][8]
Definition: vorbisdec.c:88
void(* vector_fmul_window)(float *dst, const float *src0, const float *src1, const float *win, int len)
Overlap/add with window function.
Definition: float_dsp.h:103
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
static int create_map(vorbis_context *vc, unsigned floor_number)
Definition: vorbisdec.c:835
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: vorbisdec.c:66
static int vorbis_floor1_decode(vorbis_context *vc, vorbis_floor_data *vfu, float *vec)
Definition: vorbisdec.c:1187
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
external API header
Definition: get_bits.h:63
Definition: fft.h:62
union vorbis_floor::vorbis_floor_u data
uint8_t partitions
Definition: vorbisdec.c:83
int ff_vorbis_len2vlc(uint8_t *bits, uint32_t *codes, unsigned num)
Definition: vorbis.c:57
Harmonics mapping(fx) Xmag(ploc)
static av_always_inline int get_vlc2(GetBitContext *s, VLC_TYPE(*table)[2], int bits, int max_depth)
Parse a vlc code.
Definition: get_bits.h:524
Definition: float_dsp.h:24
uint16_t bark_map_size
Definition: vorbisdec.c:73
static int vorbis_parse_setup_hdr_residues(vorbis_context *vc)
Definition: vorbisdec.c:678
static int vorbis_parse_setup_hdr_mappings(vorbis_context *vc)
Definition: vorbisdec.c:760
1i.*Xphase exp()
static int vorbis_decode_frame(AVCodecContext *avctx, void *data, int *got_frame_ptr, AVPacket *avpkt)
Definition: vorbisdec.c:1678
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
#define init_vlc(vlc, nb_bits, nb_codes,bits, bits_wrap, bits_size,codes, codes_wrap, codes_size,flags)
Definition: get_bits.h:426
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
static int vorbis_parse_setup_hdr(vorbis_context *vc)
Definition: vorbisdec.c:899
int avpriv_split_xiph_headers(uint8_t *extradata, int extradata_size, int first_header_size, uint8_t *header_start[3], int header_len[3])
Split a single extradata buffer into the three headers that most Xiph codecs use. ...
Definition: xiph.c:24
void av_frame_unref(AVFrame *frame)
Unreference all the buffers referenced by frame and reset the frame fields.
Definition: frame.c:330
uint8_t amplitude_offset
Definition: vorbisdec.c:77
static unsigned int get_bits_long(GetBitContext *s, int n)
Read 0-32 bits.
Definition: get_bits.h:306
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
void(* imdct_half)(struct FFTContext *s, FFTSample *output, const FFTSample *input)
Definition: fft.h:82
vorbis_floor1_entry * list
Definition: vorbisdec.c:91
uint8_t class_dimensions[16]
Definition: vorbisdec.c:85
static av_cold int vorbis_decode_init(AVCodecContext *avctx)
Definition: vorbisdec.c:1012
uint8_t class_masterbook[16]
Definition: vorbisdec.c:87
Definition: get_bits.h:54
common internal api header.
struct vorbis_floor::vorbis_floor_u::vorbis_floor1_s t1
Definition: vorbisdec.c:119
struct vorbis_context_s vorbis_context
uint8_t partition_class[32]
Definition: vorbisdec.c:84
static int vorbis_residue_decode(vorbis_context *vc, vorbis_residue *vr, unsigned ch, uint8_t *do_not_decode, float *vec, unsigned vlen, unsigned ch_left)
Definition: vorbisdec.c:1473
static int vorbis_parse_setup_hdr_tdtransforms(vorbis_context *vc)
Definition: vorbisdec.c:467
static int vorbis_parse_setup_hdr_modes(vorbis_context *vc)
Definition: vorbisdec.c:870
av_cold void ff_fmt_convert_init(FmtConvertContext *c, AVCodecContext *avctx)
Definition: fmtconvert.c:79
uint16_t x_list_dim
Definition: vorbisdec.c:90
void ff_vorbis_floor1_render_list(vorbis_floor1_entry *list, int values, uint16_t *y_list, int *flag, int multiplier, float *out, int samples)
Definition: vorbis.c:214
struct vorbis_floor::vorbis_floor_u::vorbis_floor0_s t0
Definition: vorbisdec.c:109
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
Definition: vorbisdec.c:126
void avpriv_float_dsp_init(AVFloatDSPContext *fdsp, int bit_exact)
Initialize a float DSP context.
Definition: float_dsp.c:118
const uint8_t ff_vorbis_channel_layout_offsets[8][8]
Definition: vorbis_data.c:25
static av_cold void vorbis_decode_flush(AVCodecContext *avctx)
Definition: vorbisdec.c:1777
Definition: vorbis.h:32
static int vorbis_parse_setup_hdr_codebooks(vorbis_context *vc)
Definition: vorbisdec.c:239
static int vorbis_parse_audio_packet(vorbis_context *vc, float **floor_ptr)
Definition: vorbisdec.c:1517
Definition: libavcodec/avcodec.h:386
trying all byte sequences megabyte in length and selecting the best looking sequence will yield cases to try But a word about which is also called distortion Distortion can be quantified by almost any quality measurement one chooses the sum of squared differences is used but more complex methods that consider psychovisual effects can be used as well It makes no difference in this discussion First step
Definition: rate_distortion.txt:12
int ff_vorbis_ready_floor1_list(AVCodecContext *avctx, vorbis_floor1_entry *list, int values)
Definition: vorbis.c:124
Generated on Fri Dec 20 2024 06:56:09 for FFmpeg by
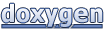