FFmpeg
|
Vorbis I decoder. More...
#include <inttypes.h>
#include <math.h>
#include "libavutil/float_dsp.h"
#include "libavutil/avassert.h"
#include "avcodec.h"
#include "get_bits.h"
#include "fft.h"
#include "fmtconvert.h"
#include "internal.h"
#include "vorbis.h"
#include "vorbisdsp.h"
#include "xiph.h"
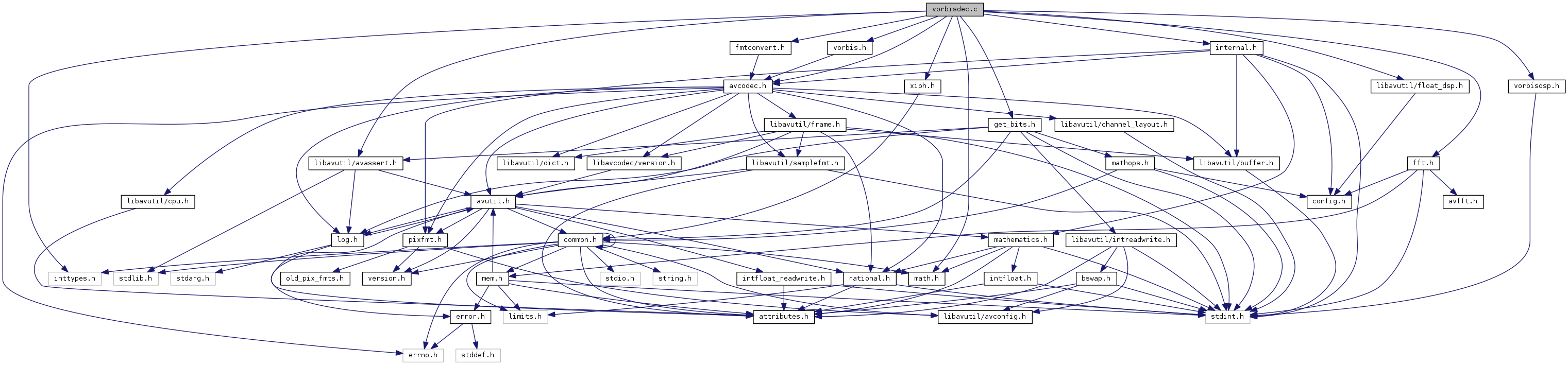
Go to the source code of this file.
Data Structures | |
struct | vorbis_codebook |
struct | vorbis_floor |
union | vorbis_floor::vorbis_floor_u |
struct | vorbis_floor::vorbis_floor_u::vorbis_floor0_s |
struct | vorbis_floor::vorbis_floor_u::vorbis_floor1_s |
struct | vorbis_residue |
struct | vorbis_mapping |
struct | vorbis_mode |
struct | vorbis_context_s |
Macros | |
#define | BITSTREAM_READER_LE |
#define | V_NB_BITS 8 |
#define | V_NB_BITS2 11 |
#define | V_MAX_VLCS (1 << 16) |
#define | V_MAX_PARTITIONS (1 << 20) |
#define | BARK(x) (13.1f * atan(0.00074f * (x)) + 2.24f * atan(1.85e-8f * (x) * (x)) + 1e-4f * (x)) |
#define | VALIDATE_INDEX(idx, limit) |
#define | GET_VALIDATED_INDEX(idx, bits, limit) |
Typedefs | |
typedef union vorbis_floor_u | vorbis_floor_data |
typedef struct vorbis_floor0_s | vorbis_floor0 |
typedef struct vorbis_floor1_s | vorbis_floor1 |
typedef int(* | vorbis_floor_decode_func) (struct vorbis_context_s *, vorbis_floor_data *, float *) |
typedef struct vorbis_context_s | vorbis_context |
Variables | |
static const char | idx_err_str [] = "Index value %d out of range (0 - %d) for %s at %s:%i\n" |
AVCodec | ff_vorbis_decoder |
Detailed Description
Vorbis I decoder.
This file is part of FFmpeg.
FFmpeg is free software; you can redistribute it and/or modify it under the terms of the GNU Lesser General Public License as published by the Free Software Foundation; either version 2.1 of the License, or (at your option) any later version.
FFmpeg is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public License along with FFmpeg; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
Definition in file vorbisdec.c.
Macro Definition Documentation
#define BARK | ( | x | ) | (13.1f * atan(0.00074f * (x)) + 2.24f * atan(1.85e-8f * (x) * (x)) + 1e-4f * (x)) |
Definition at line 161 of file vorbisdec.c.
Referenced by create_map().
#define BITSTREAM_READER_LE |
Definition at line 32 of file vorbisdec.c.
#define GET_VALIDATED_INDEX | ( | idx, | |
bits, | |||
limit | |||
) |
Definition at line 172 of file vorbisdec.c.
Referenced by vorbis_parse_audio_packet(), vorbis_parse_setup_hdr_floors(), vorbis_parse_setup_hdr_mappings(), vorbis_parse_setup_hdr_modes(), and vorbis_parse_setup_hdr_residues().
#define V_MAX_PARTITIONS (1 << 20) |
Definition at line 48 of file vorbisdec.c.
Referenced by vorbis_parse_setup_hdr_residues().
#define V_MAX_VLCS (1 << 16) |
Definition at line 47 of file vorbisdec.c.
Referenced by vorbis_parse_setup_hdr_codebooks().
#define V_NB_BITS 8 |
Definition at line 45 of file vorbisdec.c.
Referenced by vorbis_parse_setup_hdr_codebooks().
#define V_NB_BITS2 11 |
Definition at line 46 of file vorbisdec.c.
Referenced by vorbis_parse_setup_hdr_codebooks().
#define VALIDATE_INDEX | ( | idx, | |
limit | |||
) |
Definition at line 165 of file vorbisdec.c.
Referenced by vorbis_parse_setup_hdr_floors().
Typedef Documentation
typedef struct vorbis_context_s vorbis_context |
typedef struct vorbis_floor0_s vorbis_floor0 |
Definition at line 60 of file vorbisdec.c.
typedef struct vorbis_floor1_s vorbis_floor1 |
Definition at line 61 of file vorbisdec.c.
typedef union vorbis_floor_u vorbis_floor_data |
Definition at line 59 of file vorbisdec.c.
typedef int(* vorbis_floor_decode_func) (struct vorbis_context_s *, vorbis_floor_data *, float *) |
Definition at line 65 of file vorbisdec.c.
Function Documentation
|
static |
Definition at line 835 of file vorbisdec.c.
Referenced by vorbis_parse_setup_hdr_floors(), and vorbis_parse_setup_hdr_tdtransforms().
void ff_vorbis_inverse_coupling | ( | float * | mag, |
float * | ang, | ||
intptr_t | blocksize | ||
) |
Definition at line 1491 of file vorbisdec.c.
Referenced by ff_vorbisdsp_init().
|
static |
Definition at line 1768 of file vorbisdec.c.
|
static |
Definition at line 1777 of file vorbisdec.c.
|
static |
Definition at line 1678 of file vorbisdec.c.
|
static |
Definition at line 1012 of file vorbisdec.c.
|
static |
Definition at line 1079 of file vorbisdec.c.
Referenced by vorbis_parse_setup_hdr_floors(), and vorbis_parse_setup_hdr_tdtransforms().
|
static |
Definition at line 1187 of file vorbisdec.c.
Referenced by vorbis_parse_setup_hdr_floors(), and vorbis_parse_setup_hdr_tdtransforms().
|
static |
Definition at line 190 of file vorbisdec.c.
Referenced by vorbis_decode_close(), vorbis_decode_frame(), and vorbis_decode_init().
|
static |
Definition at line 1517 of file vorbisdec.c.
Referenced by vorbis_decode_frame().
|
static |
Definition at line 945 of file vorbisdec.c.
Referenced by vorbis_decode_frame(), and vorbis_decode_init().
|
static |
Definition at line 899 of file vorbisdec.c.
Referenced by vorbis_decode_frame(), and vorbis_decode_init().
|
static |
Definition at line 239 of file vorbisdec.c.
Referenced by vorbis_parse_setup_hdr().
|
static |
Definition at line 493 of file vorbisdec.c.
Referenced by vorbis_parse_setup_hdr().
|
static |
Definition at line 760 of file vorbisdec.c.
Referenced by vorbis_parse_setup_hdr().
|
static |
Definition at line 870 of file vorbisdec.c.
Referenced by vorbis_parse_setup_hdr().
|
static |
Definition at line 678 of file vorbisdec.c.
Referenced by vorbis_parse_setup_hdr().
|
static |
Definition at line 467 of file vorbisdec.c.
Referenced by vorbis_parse_setup_hdr().
|
inlinestatic |
Definition at line 1473 of file vorbisdec.c.
Referenced by vorbis_parse_audio_packet().
|
static |
Definition at line 1313 of file vorbisdec.c.
Referenced by vorbis_residue_decode().
|
static |
Definition at line 178 of file vorbisdec.c.
Referenced by vorbis_parse_setup_hdr_codebooks().
Variable Documentation
AVCodec ff_vorbis_decoder |
Definition at line 1788 of file vorbisdec.c.
Generated on Sat Feb 22 2025 06:54:51 for FFmpeg by
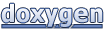