FFmpeg
|
cook.c
Go to the documentation of this file.
1093 av_log(avctx, AV_LOG_ERROR, "js_subband_start %d is too large\n", q->subpacket[s].js_subband_start);
1214 av_log(avctx, AV_LOG_ERROR, "Too many subpackets %d for channels %d\n", q->num_subpackets, q->avctx->channels);
static void mlt_compensate_output(COOKContext *q, float *decode_buffer, cook_gains *gains_ptr, float *previous_buffer, float *out)
Final part of subpacket decoding: Apply modulated lapped transform, gain compensation, clip and convert to integer.
Definition: cook.c:895
Definition: start.py:1
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
av_cold void ff_dsputil_init(DSPContext *c, AVCodecContext *avctx)
Definition: dsputil.c:2675
void(* imlt_window)(struct cook *q, float *buffer1, cook_gains *gains_ptr, float *previous_buffer)
Definition: cook.c:116
void(* interpolate)(struct cook *q, float *buffer, int gain_index, int gain_index_next)
Definition: cook.c:119
static const uint16_t envelope_quant_index_huffcodes[13][24]
Definition: cookdata.h:97
if max(w)>1 w=0.9 *w/max(w)
static void categorize(COOKContext *q, COOKSubpacket *p, const int *quant_index_table, int *category, int *category_index)
Calculate the category and category_index vector.
Definition: cook.c:399
Definition: cook.c:71
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
struct cook COOKContext
int block_align
number of bytes per packet if constant and known or 0 Used by some WAV based audio codecs...
Definition: libavcodec/avcodec.h:1898
Definition: libavcodec/avcodec.h:401
Definition: af_biquads.c:76
static void imlt_gain(COOKContext *q, float *inbuffer, cook_gains *gains_ptr, float *previous_buffer)
The modulated lapped transform, this takes transform coefficients and transforms them into timedomain...
Definition: cook.c:712
void(* scalar_dequant)(struct cook *q, int index, int quant_index, int *subband_coef_index, int *subband_coef_sign, float *mlt_p)
Definition: cook.c:105
void void avpriv_request_sample(void *avc, const char *msg,...) av_printf_format(2
Log a generic warning message about a missing feature.
Definition: samplefmt.h:50
static int decouple_info(COOKContext *q, COOKSubpacket *p, int *decouple_tab)
function for getting the jointstereo coupling information
Definition: cook.c:742
static void decode_vectors(COOKContext *q, COOKSubpacket *p, int *category, int *quant_index_table, float *mlt_buffer)
Fill the mlt_buffer with mlt coefficients.
Definition: cook.c:591
static void expand_category(COOKContext *q, int *category, int *category_index)
Expand the category vector.
Definition: cook.c:496
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
bitstream reader API header.
static int decode_subpacket(COOKContext *q, COOKSubpacket *p, const uint8_t *inbuffer, float **outbuffer)
Cook subpacket decoding.
Definition: cook.c:913
static int cook_decode_frame(AVCodecContext *avctx, void *data, int *got_frame_ptr, AVPacket *avpkt)
Definition: cook.c:955
static void decode_bytes_and_gain(COOKContext *q, COOKSubpacket *p, const uint8_t *inbuffer, cook_gains *gains_ptr)
First part of subpacket decoding: decode raw stream bytes and read gain info.
Definition: cook.c:855
void(* vector_clipf)(float *dst, const float *src, float min, float max, int len)
Definition: dsputil.h:215
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
int av_get_channel_layout_nb_channels(uint64_t channel_layout)
Return the number of channels in the channel layout.
Definition: channel_layout.c:191
Definition: avutil.h:144
static int decode_envelope(COOKContext *q, COOKSubpacket *p, int *quant_index_table)
Create the quant index table needed for the envelope.
Definition: cook.c:358
void(* imdct_calc)(struct FFTContext *s, FFTSample *output, const FFTSample *input)
Definition: fft.h:81
external API header
Definition: get_bits.h:63
static void saturate_output_float(COOKContext *q, float *out)
Saturate the output signal and interleave.
Definition: cook.c:877
#define FF_INPUT_BUFFER_PADDING_SIZE
Required number of additionally allocated bytes at the end of the input bitstream for decoding...
Definition: libavcodec/avcodec.h:561
static int unpack_SQVH(COOKContext *q, COOKSubpacket *p, int category, int *subband_coef_index, int *subband_coef_sign)
Unpack the subband_coef_index and subband_coef_sign vectors.
Definition: cook.c:547
Definition: fft.h:62
audio channel layout utility functions
static int mono_decode(COOKContext *q, COOKSubpacket *p, float *mlt_buffer)
Definition: cook.c:627
void(* decouple)(struct cook *q, COOKSubpacket *p, int subband, float f1, float f2, float *decode_buffer, float *mlt_buffer1, float *mlt_buffer2)
Definition: cook.c:109
static av_cold int cook_decode_init(AVCodecContext *avctx)
Cook initialization.
Definition: cook.c:1050
static av_always_inline int get_vlc2(GetBitContext *s, VLC_TYPE(*table)[2], int bits, int max_depth)
Parse a vlc code.
Definition: get_bits.h:524
void ff_sine_window_init(float *window, int n)
Generate a sine window.
Definition: sinewin_tablegen.h:54
static void interpolate_float(COOKContext *q, float *buffer, int gain_index, int gain_index_next)
the actual requantization of the timedomain samples
Definition: cook.c:657
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
#define init_vlc(vlc, nb_bits, nb_codes,bits, bits_wrap, bits_size,codes, codes_wrap, codes_size,flags)
Definition: get_bits.h:426
static unsigned int av_lfg_get(AVLFG *c)
Get the next random unsigned 32-bit number using an ALFG.
Definition: lfg.h:38
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
static int joint_decode(COOKContext *q, COOKSubpacket *p, float *mlt_buffer_left, float *mlt_buffer_right)
function for decoding joint stereo data
Definition: cook.c:803
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
static const uint8_t envelope_quant_index_huffbits[13][24]
Definition: cookdata.h:81
#define PRINT(a, b)
static int decode_bytes(const uint8_t *inbuffer, uint8_t *out, int bytes)
Cook indata decoding, every 32 bits are XORed with 0x37c511f2.
Definition: cook.c:272
static void scalar_dequant_float(COOKContext *q, int index, int quant_index, int *subband_coef_index, int *subband_coef_sign, float *mlt_p)
The real requantization of the mltcoefs.
Definition: cook.c:518
Definition: get_bits.h:54
common internal api header.
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
DSP utils.
DECLARE_ALIGNED(32, float, mono_mdct_output)[2048]
Definition: cook.c:66
Filter the word “frame” indicates either a video frame or a group of audio samples
Definition: filter_design.txt:2
static void decode_gain_info(GetBitContext *gb, int *gaininfo)
Fill the gain array for the timedomain quantization.
Definition: cook.c:330
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> out
Definition: audio_convert.c:194
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
static void imlt_window_float(COOKContext *q, float *inbuffer, cook_gains *gains_ptr, float *previous_buffer)
Apply transform window, overlap buffers.
Definition: cook.c:684
static void decouple_float(COOKContext *q, COOKSubpacket *p, int subband, float f1, float f2, float *decode_buffer, float *mlt_buffer1, float *mlt_buffer2)
function decouples a pair of signals from a single signal via multiplication.
Definition: cook.c:781
Cook AKA RealAudio G2 compatible decoderdata.
Definition: cook.c:100
Generated on Thu May 29 2025 06:52:45 for FFmpeg by
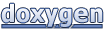