FFmpeg
|
Cook compatible decoder. More...
#include "libavutil/channel_layout.h"
#include "libavutil/lfg.h"
#include "avcodec.h"
#include "get_bits.h"
#include "dsputil.h"
#include "bytestream.h"
#include "fft.h"
#include "internal.h"
#include "sinewin.h"
#include "cookdata.h"
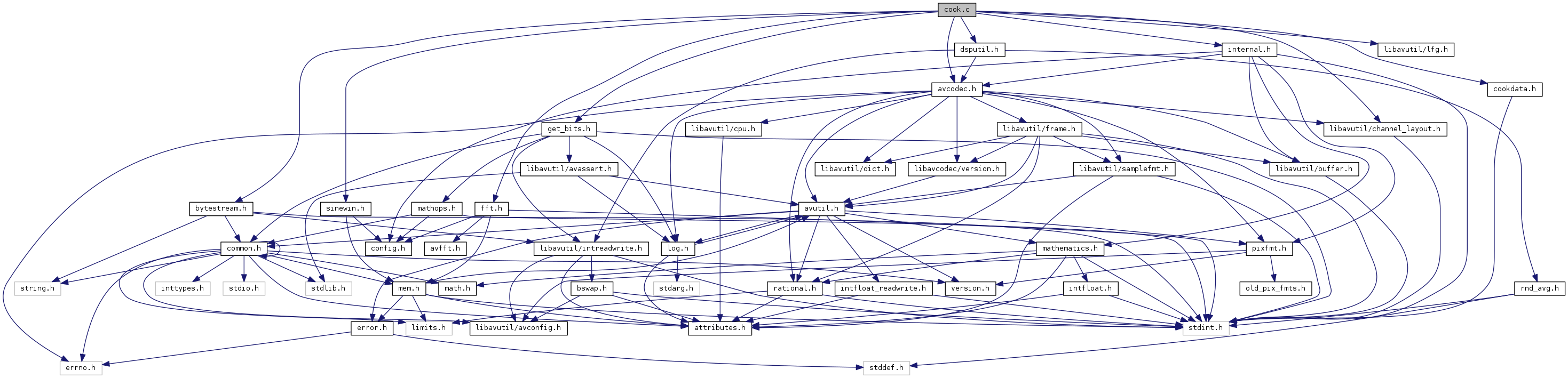
Go to the source code of this file.
Data Structures | |
struct | cook_gains |
struct | COOKSubpacket |
struct | cook |
Macros | |
#define | MONO 0x1000001 |
#define | STEREO 0x1000002 |
#define | JOINT_STEREO 0x1000003 |
#define | MC_COOK 0x2000000 |
#define | SUBBAND_SIZE 20 |
#define | MAX_SUBPACKETS 5 |
#define | DECODE_BYTES_PAD1(bytes) (3 - ((bytes) + 3) % 4) |
#define | DECODE_BYTES_PAD2(bytes) ((bytes) % 4 + DECODE_BYTES_PAD1(2 * (bytes))) |
Typedefs | |
typedef struct cook | COOKContext |
Functions | |
static av_cold void | init_pow2table (void) |
static av_cold void | init_gain_table (COOKContext *q) |
static av_cold int | init_cook_vlc_tables (COOKContext *q) |
static av_cold int | init_cook_mlt (COOKContext *q) |
static av_cold void | init_cplscales_table (COOKContext *q) |
static int | decode_bytes (const uint8_t *inbuffer, uint8_t *out, int bytes) |
Cook indata decoding, every 32 bits are XORed with 0x37c511f2. More... | |
static av_cold int | cook_decode_close (AVCodecContext *avctx) |
static void | decode_gain_info (GetBitContext *gb, int *gaininfo) |
Fill the gain array for the timedomain quantization. More... | |
static int | decode_envelope (COOKContext *q, COOKSubpacket *p, int *quant_index_table) |
Create the quant index table needed for the envelope. More... | |
static void | categorize (COOKContext *q, COOKSubpacket *p, const int *quant_index_table, int *category, int *category_index) |
Calculate the category and category_index vector. More... | |
static void | expand_category (COOKContext *q, int *category, int *category_index) |
Expand the category vector. More... | |
static void | scalar_dequant_float (COOKContext *q, int index, int quant_index, int *subband_coef_index, int *subband_coef_sign, float *mlt_p) |
The real requantization of the mltcoefs. More... | |
static int | unpack_SQVH (COOKContext *q, COOKSubpacket *p, int category, int *subband_coef_index, int *subband_coef_sign) |
Unpack the subband_coef_index and subband_coef_sign vectors. More... | |
static void | decode_vectors (COOKContext *q, COOKSubpacket *p, int *category, int *quant_index_table, float *mlt_buffer) |
Fill the mlt_buffer with mlt coefficients. More... | |
static int | mono_decode (COOKContext *q, COOKSubpacket *p, float *mlt_buffer) |
static void | interpolate_float (COOKContext *q, float *buffer, int gain_index, int gain_index_next) |
the actual requantization of the timedomain samples More... | |
static void | imlt_window_float (COOKContext *q, float *inbuffer, cook_gains *gains_ptr, float *previous_buffer) |
Apply transform window, overlap buffers. More... | |
static void | imlt_gain (COOKContext *q, float *inbuffer, cook_gains *gains_ptr, float *previous_buffer) |
The modulated lapped transform, this takes transform coefficients and transforms them into timedomain samples. More... | |
static int | decouple_info (COOKContext *q, COOKSubpacket *p, int *decouple_tab) |
function for getting the jointstereo coupling information More... | |
static void | decouple_float (COOKContext *q, COOKSubpacket *p, int subband, float f1, float f2, float *decode_buffer, float *mlt_buffer1, float *mlt_buffer2) |
function decouples a pair of signals from a single signal via multiplication. More... | |
static int | joint_decode (COOKContext *q, COOKSubpacket *p, float *mlt_buffer_left, float *mlt_buffer_right) |
function for decoding joint stereo data More... | |
static void | decode_bytes_and_gain (COOKContext *q, COOKSubpacket *p, const uint8_t *inbuffer, cook_gains *gains_ptr) |
First part of subpacket decoding: decode raw stream bytes and read gain info. More... | |
static void | saturate_output_float (COOKContext *q, float *out) |
Saturate the output signal and interleave. More... | |
static void | mlt_compensate_output (COOKContext *q, float *decode_buffer, cook_gains *gains_ptr, float *previous_buffer, float *out) |
Final part of subpacket decoding: Apply modulated lapped transform, gain compensation, clip and convert to integer. More... | |
static int | decode_subpacket (COOKContext *q, COOKSubpacket *p, const uint8_t *inbuffer, float **outbuffer) |
Cook subpacket decoding. More... | |
static int | cook_decode_frame (AVCodecContext *avctx, void *data, int *got_frame_ptr, AVPacket *avpkt) |
static av_cold int | cook_decode_init (AVCodecContext *avctx) |
Cook initialization. More... | |
Variables | |
static float | pow2tab [127] |
static float | rootpow2tab [127] |
AVCodec | ff_cook_decoder |
Detailed Description
Cook compatible decoder.
Bastardization of the G.722.1 standard. This decoder handles RealNetworks, RealAudio G2 data. Cook is identified by the codec name cook in RM files.
To use this decoder, a calling application must supply the extradata bytes provided from the RM container; 8+ bytes for mono streams and 16+ for stereo streams (maybe more).
Codec technicalities (all this assume a buffer length of 1024): Cook works with several different techniques to achieve its compression. In the timedomain the buffer is divided into 8 pieces and quantized. If two neighboring pieces have different quantization index a smooth quantization curve is used to get a smooth overlap between the different pieces. To get to the transformdomain Cook uses a modulated lapped transform. The transform domain has 50 subbands with 20 elements each. This means only a maximum of 50*20=1000 coefficients are used out of the 1024 available.
Definition in file cook.c.
Macro Definition Documentation
#define DECODE_BYTES_PAD1 | ( | bytes | ) | (3 - ((bytes) + 3) % 4) |
Definition at line 249 of file cook.c.
Referenced by cook_decode_init().
#define DECODE_BYTES_PAD2 | ( | bytes | ) | ((bytes) % 4 + DECODE_BYTES_PAD1(2 * (bytes))) |
#define JOINT_STEREO 0x1000003 |
Definition at line 60 of file cook.c.
Referenced by cook_decode_init().
#define MAX_SUBPACKETS 5 |
Definition at line 64 of file cook.c.
Referenced by cook_decode_init().
#define MC_COOK 0x2000000 |
Definition at line 61 of file cook.c.
Referenced by cook_decode_init().
#define MONO 0x1000001 |
Definition at line 58 of file cook.c.
Referenced by cook_decode_init(), libshine_encode_init(), and mp3lame_encode_init().
#define STEREO 0x1000002 |
Definition at line 59 of file cook.c.
Referenced by cook_decode_frame(), and cook_decode_init().
#define SUBBAND_SIZE 20 |
Definition at line 63 of file cook.c.
Referenced by decode_vectors(), decouple_float(), joint_decode(), and scalar_dequant_float().
Typedef Documentation
typedef struct cook COOKContext |
Function Documentation
|
static |
Calculate the category and category_index vector.
- Parameters
-
q pointer to the COOKContext quant_index_table pointer to the array category pointer to the category array category_index pointer to the category_index array
Definition at line 399 of file cook.c.
Referenced by mono_decode().
|
static |
|
static |
|
static |
Cook initialization.
- Parameters
-
avctx pointer to the AVCodecContext
Cook indata decoding, every 32 bits are XORed with 0x37c511f2.
Why? No idea, some checksum/error detection method maybe.
Out buffer size: extra bytes are needed to cope with padding/misalignment. Subpackets passed to the decoder can contain two, consecutive half-subpackets, of identical but arbitrary size. 1234 1234 1234 1234 extraA extraB Case 1: AAAA BBBB 0 0 Case 2: AAAA ABBB BB– 3 3 Case 3: AAAA AABB BBBB 2 2 Case 4: AAAA AAAB BBBB BB– 1 5
Nice way to waste CPU cycles.
- Parameters
-
inbuffer pointer to byte array of indata out pointer to byte array of outdata bytes number of bytes
Definition at line 272 of file cook.c.
Referenced by decode_bytes_and_gain().
|
inlinestatic |
First part of subpacket decoding: decode raw stream bytes and read gain info.
- Parameters
-
q pointer to the COOKContext inbuffer pointer to raw stream data gains_ptr array of current/prev gain pointers
Definition at line 855 of file cook.c.
Referenced by decode_subpacket().
|
static |
Create the quant index table needed for the envelope.
- Parameters
-
q pointer to the COOKContext quant_index_table pointer to the array
Definition at line 358 of file cook.c.
Referenced by mono_decode().
|
static |
Fill the gain array for the timedomain quantization.
- Parameters
-
gb pointer to the GetBitContext gaininfo array[9] of gain indexes
Definition at line 330 of file cook.c.
Referenced by decode_bytes_and_gain().
|
static |
Cook subpacket decoding.
This function returns one decoded subpacket, usually 1024 samples per channel.
- Parameters
-
q pointer to the COOKContext inbuffer pointer to the inbuffer outbuffer pointer to the outbuffer
Definition at line 913 of file cook.c.
Referenced by cook_decode_frame().
|
static |
Fill the mlt_buffer with mlt coefficients.
- Parameters
-
q pointer to the COOKContext category pointer to the category array quant_index_table pointer to the array mlt_buffer pointer to mlt coefficients
Definition at line 591 of file cook.c.
Referenced by mono_decode().
|
static |
function decouples a pair of signals from a single signal via multiplication.
- Parameters
-
q pointer to the COOKContext subband index of the current subband f1 multiplier for channel 1 extraction f2 multiplier for channel 2 extraction decode_buffer input buffer mlt_buffer1 pointer to left channel mlt coefficients mlt_buffer2 pointer to right channel mlt coefficients
Definition at line 781 of file cook.c.
Referenced by cook_decode_init().
|
static |
function for getting the jointstereo coupling information
- Parameters
-
q pointer to the COOKContext decouple_tab decoupling array
Definition at line 742 of file cook.c.
Referenced by joint_decode().
|
inlinestatic |
Expand the category vector.
- Parameters
-
q pointer to the COOKContext category pointer to the category array category_index pointer to the category_index array
Definition at line 496 of file cook.c.
Referenced by mono_decode().
|
static |
The modulated lapped transform, this takes transform coefficients and transforms them into timedomain samples.
Apply transform window, overlap buffers, apply gain profile and buffer management.
- Parameters
-
q pointer to the COOKContext inbuffer pointer to the mltcoefficients gains_ptr current and previous gains previous_buffer pointer to the previous buffer to be used for overlapping
Definition at line 712 of file cook.c.
Referenced by mlt_compensate_output().
|
static |
Apply transform window, overlap buffers.
- Parameters
-
q pointer to the COOKContext inbuffer pointer to the mltcoefficients gains_ptr current and previous gains previous_buffer pointer to the previous buffer to be used for overlapping
Definition at line 684 of file cook.c.
Referenced by cook_decode_init().
|
static |
Definition at line 216 of file cook.c.
Referenced by cook_decode_init().
|
static |
Definition at line 185 of file cook.c.
Referenced by cook_decode_init().
|
static |
Definition at line 240 of file cook.c.
Referenced by cook_decode_init().
|
static |
Definition at line 175 of file cook.c.
Referenced by cook_decode_init().
Definition at line 165 of file cook.c.
Referenced by cook_decode_init().
|
static |
the actual requantization of the timedomain samples
- Parameters
-
q pointer to the COOKContext buffer pointer to the timedomain buffer gain_index index for the block multiplier gain_index_next index for the next block multiplier
Definition at line 657 of file cook.c.
Referenced by cook_decode_init().
|
static |
function for decoding joint stereo data
- Parameters
-
q pointer to the COOKContext mlt_buffer1 pointer to left channel mlt coefficients mlt_buffer2 pointer to right channel mlt coefficients
Definition at line 803 of file cook.c.
Referenced by decode_subpacket().
|
inlinestatic |
Final part of subpacket decoding: Apply modulated lapped transform, gain compensation, clip and convert to integer.
- Parameters
-
q pointer to the COOKContext decode_buffer pointer to the mlt coefficients gains_ptr array of current/prev gain pointers previous_buffer pointer to the previous buffer to be used for overlapping out pointer to the output buffer
Definition at line 895 of file cook.c.
Referenced by decode_subpacket().
|
static |
Definition at line 627 of file cook.c.
Referenced by decode_subpacket(), and joint_decode().
|
static |
Saturate the output signal and interleave.
- Parameters
-
q pointer to the COOKContext out pointer to the output vector
Definition at line 877 of file cook.c.
Referenced by cook_decode_init().
|
static |
The real requantization of the mltcoefs.
- Parameters
-
q pointer to the COOKContext index index quant_index quantisation index subband_coef_index array of indexes to quant_centroid_tab subband_coef_sign signs of coefficients mlt_p pointer into the mlt buffer
Definition at line 518 of file cook.c.
Referenced by cook_decode_init().
|
static |
Unpack the subband_coef_index and subband_coef_sign vectors.
- Parameters
-
q pointer to the COOKContext category pointer to the category array subband_coef_index array of indexes to quant_centroid_tab subband_coef_sign signs of coefficients
Definition at line 547 of file cook.c.
Referenced by decode_vectors().
Variable Documentation
AVCodec ff_cook_decoder |
|
static |
Definition at line 159 of file cook.c.
Referenced by imlt_window_float(), init_gain_table(), init_pow2table(), and interpolate_float().
|
static |
Definition at line 160 of file cook.c.
Referenced by init_pow2table(), and scalar_dequant_float().
Generated on Wed Apr 16 2025 06:53:25 for FFmpeg by
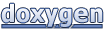