FFmpeg
|
#include <stdint.h>
#include <string.h>
#include "libavutil/common.h"
#include "libavutil/intreadwrite.h"
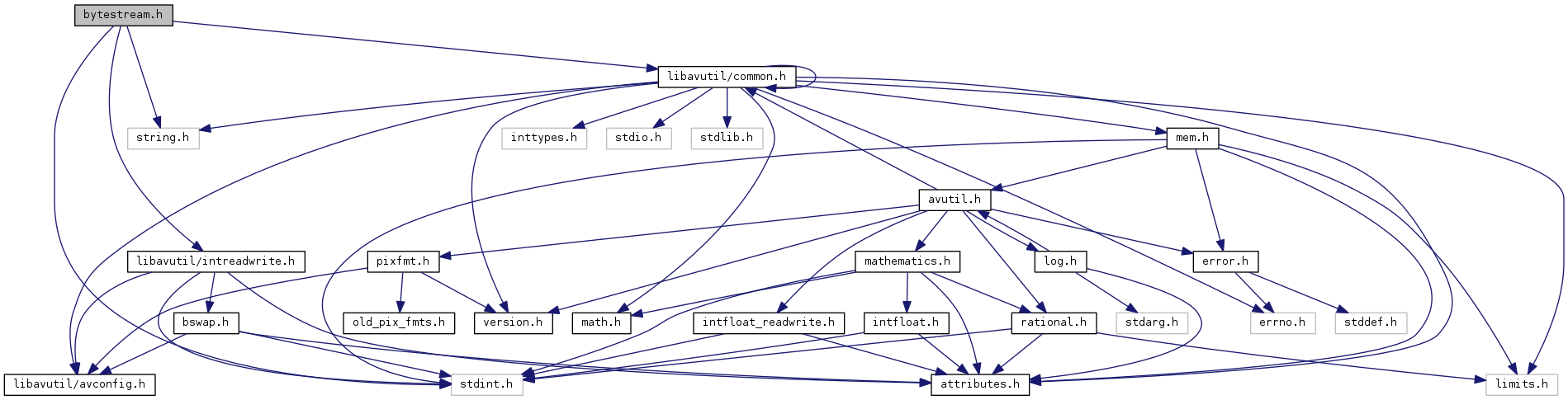
Go to the source code of this file.
Data Structures | |
struct | GetByteContext |
struct | PutByteContext |
Macros | |
#define | DEF(type, name, bytes, read, write) |
#define | bytestream2_get_ne16 bytestream2_get_le16 |
#define | bytestream2_get_ne24 bytestream2_get_le24 |
#define | bytestream2_get_ne32 bytestream2_get_le32 |
#define | bytestream2_get_ne64 bytestream2_get_le64 |
#define | bytestream2_get_ne16u bytestream2_get_le16u |
#define | bytestream2_get_ne24u bytestream2_get_le24u |
#define | bytestream2_get_ne32u bytestream2_get_le32u |
#define | bytestream2_get_ne64u bytestream2_get_le64u |
#define | bytestream2_put_ne16 bytestream2_put_le16 |
#define | bytestream2_put_ne24 bytestream2_put_le24 |
#define | bytestream2_put_ne32 bytestream2_put_le32 |
#define | bytestream2_put_ne64 bytestream2_put_le64 |
#define | bytestream2_peek_ne16 bytestream2_peek_le16 |
#define | bytestream2_peek_ne24 bytestream2_peek_le24 |
#define | bytestream2_peek_ne32 bytestream2_peek_le32 |
#define | bytestream2_peek_ne64 bytestream2_peek_le64 |
Typedefs | |
typedef struct GetByteContext | GetByteContext |
typedef struct PutByteContext | PutByteContext |
Macro Definition Documentation
#define bytestream2_get_ne16 bytestream2_get_le16 |
Definition at line 112 of file bytestream.h.
#define bytestream2_get_ne16u bytestream2_get_le16u |
Definition at line 116 of file bytestream.h.
Referenced by read_uncompressed_sgi().
#define bytestream2_get_ne24 bytestream2_get_le24 |
Definition at line 113 of file bytestream.h.
#define bytestream2_get_ne24u bytestream2_get_le24u |
Definition at line 117 of file bytestream.h.
#define bytestream2_get_ne32 bytestream2_get_le32 |
Definition at line 114 of file bytestream.h.
#define bytestream2_get_ne32u bytestream2_get_le32u |
Definition at line 118 of file bytestream.h.
#define bytestream2_get_ne64 bytestream2_get_le64 |
Definition at line 115 of file bytestream.h.
#define bytestream2_get_ne64u bytestream2_get_le64u |
Definition at line 119 of file bytestream.h.
#define bytestream2_peek_ne16 bytestream2_peek_le16 |
Definition at line 124 of file bytestream.h.
#define bytestream2_peek_ne24 bytestream2_peek_le24 |
Definition at line 125 of file bytestream.h.
#define bytestream2_peek_ne32 bytestream2_peek_le32 |
Definition at line 126 of file bytestream.h.
#define bytestream2_peek_ne64 bytestream2_peek_le64 |
Definition at line 127 of file bytestream.h.
#define bytestream2_put_ne16 bytestream2_put_le16 |
Definition at line 120 of file bytestream.h.
#define bytestream2_put_ne24 bytestream2_put_le24 |
Definition at line 121 of file bytestream.h.
#define bytestream2_put_ne32 bytestream2_put_le32 |
Definition at line 122 of file bytestream.h.
#define bytestream2_put_ne64 bytestream2_put_le64 |
Definition at line 123 of file bytestream.h.
Definition at line 41 of file bytestream.h.
Referenced by ff_avg_pixels16(), ff_avg_pixels16_x2(), ff_avg_pixels16_xy2(), ff_avg_pixels16_y2(), ff_put_no_rnd_pixels16_x2(), ff_put_no_rnd_pixels16_y2(), and ff_put_pixels16_y2().
Typedef Documentation
typedef struct GetByteContext GetByteContext |
typedef struct PutByteContext PutByteContext |
Function Documentation
|
static |
Definition at line 258 of file bytestream.h.
Referenced by bethsoftvid_decode_frame(), bfi_decode_frame(), copy4h(), decode_bdlt(), decode_copy(), decode_format80(), decode_frame(), decode_tdlt(), decode_wdlt(), ff_amf_read_string(), flic_decode_frame_8BPP(), ipvideo_decode_block_opcode_0x9(), ipvideo_decode_block_opcode_0xA(), ipvideo_decode_block_opcode_0xB(), old_codec37(), paf_vid_decode(), pcm_bluray_decode_frame(), pcx_rle_decode(), targa_decode_rle(), tgq_decode_mb(), txd_decode_frame(), vb_decode_framedata(), vmd_decode(), vqa_decode_chunk(), and xan_unpack().
|
static |
Definition at line 268 of file bytestream.h.
Referenced by add_string_metadata(), decode_packet(), gif_read_header1(), old_codec37(), old_codec47(), op(), paf_vid_decode(), process_block(), rle_decode(), rle_unpack(), tiff_decode_tag(), vmd_decode(), and xwd_decode_frame().
|
static |
Definition at line 149 of file bytestream.h.
Referenced by add_doubles_metadata(), add_shorts_metadata(), add_string_metadata(), adpcm_decode_frame(), bfi_decode_frame(), brpix_decode_frame(), codec2subblock(), decode_0(), decode_6(), decode_bdlt(), decode_codestream(), decode_dds1(), decode_dsw1(), decode_frame(), decode_init(), decode_mvc1(), decode_mvc2(), decode_p_block(), decode_packet(), decode_rgb8(), decode_rgbn(), decode_tdlt(), decode_tsw1(), decode_wdlt(), dfa_decode_frame(), expand_rle_row(), flic_decode_frame_15_16BPP(), flic_decode_frame_8BPP(), get_coc(), get_cod(), get_cox(), get_qcc(), get_qcx(), get_siz(), get_sot(), gif_parse_next_image(), gif_read_extension(), gif_read_header1(), gif_read_image(), handle_notify(), ipvideo_decode_opcodes(), jp2_find_codestream(), kmvc_decode_inter_8x8(), kmvc_decode_intra_8x8(), lz_unpack(), mm_decode_inter(), mm_decode_intra(), msrle_decode_8_16_24_32(), msrle_decode_pal4(), mss3_decode_frame(), mss4_decode_frame(), old_codec1(), old_codec37(), old_codec47(), op(), opcode_0xf7(), opcode_0xf8(), paf_vid_decode(), pcx_palette(), png_decode_idat(), process_block(), qpeg_decode_inter(), qpeg_decode_intra(), read_connect(), read_frame_header(), read_rle_sgi(), read_uncompressed_sgi(), rle_decode(), rle_unpack(), roqvideo_decode_frame(), set_palette(), targa_decode_rle(), tgq_decode_mb(), tiff_decode_tag(), tscc2_decode_frame(), txd_decode_frame(), ulti_decode_frame(), vb_decode_framedata(), vmd_decode(), vqa_decode_chunk(), xan_decode_chroma(), xan_decode_frame(), xan_unpack(), xan_unpack_luma(), and xwd_decode_frame().
|
static |
Definition at line 154 of file bytestream.h.
|
static |
Definition at line 323 of file bytestream.h.
|
static |
Definition at line 130 of file bytestream.h.
Referenced by aasc_decode_frame(), adpcm_decode_frame(), alac_set_info(), bethsoftvid_decode_frame(), bfi_decode_frame(), bmp_decode_frame(), brpix_decode_frame(), decode_frame(), decode_frame_headers(), decode_i2_frame(), decode_init(), decode_p_frame(), dfa_decode_frame(), dpcm_decode_frame(), find_tracked_method(), flic_decode_frame_15_16BPP(), flic_decode_frame_8BPP(), gif_decode_frame(), handle_notify(), ipvideo_decode_frame(), lz_unpack(), mimic_decode_frame(), mm_decode_frame(), mm_decode_inter(), msrle_decode_frame(), mss2_decode_frame(), mss3_decode_frame(), mss4_decode_frame(), mvc_decode_frame(), paf_vid_decode(), pcm_bluray_decode_frame(), pcx_decode_frame(), qtrle_decode_frame(), read_connect(), rle_unpack(), roq_decode_frame(), rtmp_send_packet(), send_invoke_response(), smc_decode_frame(), tgq_decode_frame(), tm2_read_stream(), tscc2_decode_frame(), txd_decode_frame(), ulti_decode_frame(), vmd_decode(), vqa_decode_chunk(), vqa_decode_frame(), xan_decode_frame(), xan_unpack(), and xwd_decode_frame().
|
static |
Definition at line 139 of file bytestream.h.
Referenced by encode_frame(), handle_notify(), jpeg_create_header(), send_invoke_response(), sunrast_encode_frame(), and utvideo_encode_frame().
|
static |
Definition at line 277 of file bytestream.h.
Referenced by encode_plane(), handle_notify(), jpeg_create_header(), and sunrast_image_write_image().
|
static |
Definition at line 292 of file bytestream.h.
|
static |
Definition at line 203 of file bytestream.h.
Referenced by adpcm_decode_frame(), decode_frame(), pcx_decode_frame(), process_block(), qtrle_decode_frame(), read_rle_sgi(), tiff_decode_tag(), vqa_decode_chunk(), xan_decode_chroma(), xan_decode_frame_type0(), xan_decode_frame_type1(), and xan_unpack_luma().
|
static |
Definition at line 227 of file bytestream.h.
Referenced by encode_plane().
|
static |
Definition at line 301 of file bytestream.h.
Referenced by encode_frame().
|
static |
Definition at line 315 of file bytestream.h.
|
static |
Definition at line 193 of file bytestream.h.
Referenced by smc_decode_stream(), tiff_decode_tag(), and xan_decode_frame_type0().
|
static |
Definition at line 198 of file bytestream.h.
|
static |
Definition at line 159 of file bytestream.h.
Referenced by adpcm_decode_frame(), bfi_decode_frame(), brpix_decode_frame(), brpix_decode_header(), decode_0(), decode_codestream(), decode_frame(), decode_frame_headers(), decode_mvc2(), decode_packet(), dfa_decode_frame(), flic_decode_frame_15_16BPP(), flic_decode_frame_8BPP(), get_nb_samples(), ipvideo_decode_opcodes(), j2k_flush(), mimic_decode_frame(), mm_decode_pal(), msrle_decode_8_16_24_32(), msrle_decode_pal4(), mss3_decode_frame(), mss4_decode_frame(), old_codec37(), old_codec47(), paf_vid_decode(), pcm_bluray_decode_frame(), png_decode_idat(), process_frame_obj(), qtrle_decode_2n4bpp(), qtrle_decode_frame(), read_frame_header(), read_uncompressed_sgi(), rle_unpack(), smc_decode_stream(), tgq_decode_frame(), tgq_decode_mb(), tm2_read_stream(), tscc2_decode_frame(), txd_decode_frame(), vmd_decode(), vqa_decode_chunk(), xan_decode_chroma(), xan_decode_frame(), and xan_unpack_luma().
|
static |
Definition at line 171 of file bytestream.h.
Referenced by handle_notify(), and sunrast_image_write_image().
|
static |
Definition at line 165 of file bytestream.h.
Referenced by adpcm_decode_frame(), alac_set_info(), brpix_decode_frame(), decode_0(), decode_frame(), decode_init(), dpcm_decode_frame(), get_sot(), gif_read_extension(), jp2_find_codestream(), lz_unpack(), pcx_decode_frame(), and xwd_decode_frame().
|
static |
Definition at line 183 of file bytestream.h.
Referenced by adpcm_decode_frame(), arith2_get_consumed_bytes(), bethsoftvid_decode_frame(), brpix_decode_frame(), bytestream2_seek(), decode_0(), decode_codestream(), decode_format80(), decode_frame(), flic_decode_frame_15_16BPP(), flic_decode_frame_8BPP(), ipvideo_decode_opcodes(), mss2_decode_frame(), old_codec1(), old_codec47(), pcm_bluray_decode_frame(), pcx_decode_frame(), process_block(), rle_unpack(), roqvideo_decode_frame(), tiff_decode_tag(), tm2_read_stream(), tscc2_decode_frame(), vqa_decode_chunk(), and xan_unpack_luma().
|
static |
Definition at line 188 of file bytestream.h.
Referenced by bytestream2_seek_p(), jpeg_create_header(), sunrast_encode_frame(), sunrast_image_write_image(), and utvideo_encode_frame().
|
static |
Definition at line 328 of file bytestream.h.
Referenced by cdg_decode_frame(), flac_read_header(), ogm_header(), pcm_decode_frame(), and rtmp_write().
|
static |
Definition at line 337 of file bytestream.h.
Referenced by adx_encode_header(), av_packet_merge_side_data(), encode_frame(), encode_packet(), ff_amf_write_field_name(), ff_amf_write_string(), ff_amf_write_string2(), ff_rtp_send_jpeg(), ff_vorbiscomment_write(), get_packet(), gif_image_write_image(), iff_read_header(), imx_dump_header(), libopus_write_header(), mjpega_dump_header(), ogg_build_flac_headers(), ogg_build_opus_headers(), ogg_build_speex_headers(), ogg_write_header(), pcm_encode_frame(), prores_encode_frame(), qtrle_encode_line(), read_packet(), write_codebooks(), write_typecode(), and xwd_encode_frame().
Generated on Fri Feb 21 2025 06:53:53 for FFmpeg by
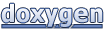