FFmpeg
|
bethsoftvideo.c
Go to the documentation of this file.
Definition: bytestream.h:32
static av_always_inline void bytestream2_init(GetByteContext *g, const uint8_t *buf, int buf_size)
Definition: bytestream.h:130
Definition: bethsoftvideo.c:36
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
Definition: libavcodec/avcodec.h:206
Definition: libavcodec/avcodec.h:918
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
static av_always_inline unsigned int bytestream2_get_buffer(GetByteContext *g, uint8_t *dst, unsigned int size)
Definition: bytestream.h:258
static av_always_inline unsigned int bytestream2_get_bytes_left(GetByteContext *g)
Definition: bytestream.h:149
static int bethsoftvid_decode_frame(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: bethsoftvideo.c:65
external API header
struct BethsoftvidContext BethsoftvidContext
int ff_reget_buffer(AVCodecContext *avctx, AVFrame *frame)
Identical in function to av_frame_make_writable(), except it uses ff_get_buffer() to allocate the buf...
Definition: libavcodec/utils.c:868
Definition: bethsoftvideo.h:32
Definition: bethsoftvideo.h:30
static av_always_inline int bytestream2_tell(GetByteContext *g)
Definition: bytestream.h:183
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
static av_cold int bethsoftvid_decode_end(AVCodecContext *avctx)
Definition: bethsoftvideo.c:145
static av_cold int bethsoftvid_decode_init(AVCodecContext *avctx)
Definition: bethsoftvideo.c:41
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
void avcodec_get_frame_defaults(AVFrame *frame)
Set the fields of the given AVFrame to default values.
Definition: libavcodec/utils.c:927
int palette_has_changed
Tell user application that palette has changed from previous frame.
Definition: frame.h:280
void av_frame_unref(AVFrame *frame)
Unreference all the buffers referenced by frame and reset the frame fields.
Definition: frame.c:330
int av_frame_ref(AVFrame *dst, AVFrame *src)
Setup a new reference to the data described by an given frame.
Definition: frame.c:228
common internal api header.
common internal and external API header
Definition: bethsoftvideo.h:27
struct AVPacket::@35 * side_data
Additional packet data that can be provided by the container.
Definition: avutil.h:143
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
Generated on Wed Jun 18 2025 06:52:58 for FFmpeg by
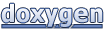