FFmpeg
|
alac.c
Go to the documentation of this file.
521 alac->direct_output = alac->sample_size > 16 && av_sample_fmt_is_planar(alac->avctx->sample_fmt);
582 req_packed = LIBAVCODEC_VERSION_MAJOR < 55 && !av_sample_fmt_is_planar(avctx->request_sample_fmt);
Definition: bytestream.h:32
static int decode_element(AVCodecContext *avctx, AVFrame *frame, int ch_index, int channels)
Definition: alac.c:256
static av_always_inline void bytestream2_init(GetByteContext *g, const uint8_t *buf, int buf_size)
Definition: bytestream.h:130
int bits_per_raw_sample
Bits per sample/pixel of internal libavcodec pixel/sample format.
Definition: libavcodec/avcodec.h:2553
Definition: libavcodec/avcodec.h:397
static int get_sbits_long(GetBitContext *s, int n)
Read 0-32 bits as a signed integer.
Definition: get_bits.h:344
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
void void avpriv_request_sample(void *avc, const char *msg,...) av_printf_format(2
Log a generic warning message about a missing feature.
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
static av_always_inline void bytestream2_skipu(GetByteContext *g, unsigned int size)
Definition: bytestream.h:165
bitstream reader API header.
static int alac_decode_frame(AVCodecContext *avctx, void *data, int *got_frame_ptr, AVPacket *avpkt)
Definition: alac.c:437
Definition: alac.c:59
static void append_extra_bits(int32_t *buffer[2], int32_t *extra_bits_buffer[2], int extra_bits, int channels, int nb_samples)
Definition: alac.c:246
static void lpc_prediction(int32_t *error_buffer, int32_t *buffer_out, int nb_samples, int bps, int16_t *lpc_coefs, int lpc_order, int lpc_quant)
Definition: alac.c:164
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: avutil.h:144
external API header
static void decorrelate_stereo(int32_t *buffer[2], int nb_samples, int decorr_shift, int decorr_left_weight)
Definition: alac.c:227
audio channel layout utility functions
const uint64_t ff_alac_channel_layouts[ALAC_MAX_CHANNELS+1]
Definition: alac_data.c:35
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip please avoid problems through this extra level of scrutiny For cosmetics only commits you should e g by running git config global user name My Name git config global user email my email which is either set in your personal configuration file through git config core editor or set by one of the following environment VISUAL or EDITOR Log messages should be concise but descriptive Explain why you made a what you did will be obvious from the changes themselves most of the time Saying just bug fix or is bad Remember that people of varying skill levels look at and educate themselves while reading through your code Don t include filenames in log Git provides that information Possibly make the commit message have a descriptive first an empty line and then a full description The first line will be used to name the patch by git format patch Renaming moving copying files or contents of making those normal commits mv cp path file otherpath otherfile git add[-A] git commit Do not rename or copy files of which you are not the maintainer without discussing it on the mailing list first Reverting broken commits git revert< commit > git revert will generate a revert commit This will not make the faulty commit disappear from the history git reset< commit > git reset will uncommit the changes till< commit > rewriting the current branch history git commit amend allows to amend the last commit details quickly git rebase i origin master will replay local commits over the main repository allowing to merge or remove some of them in the process Note that the commit amend and rebase rewrite history
Definition: git-howto.txt:153
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
int av_sample_fmt_is_planar(enum AVSampleFormat sample_fmt)
Check if the sample format is planar.
Definition: samplefmt.c:118
static unsigned int decode_scalar(GetBitContext *gb, int k, int bps)
Definition: alac.c:80
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
static unsigned int get_bits_long(GetBitContext *s, int n)
Read 0-32 bits.
Definition: get_bits.h:306
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
const uint8_t ff_alac_channel_layout_offsets[ALAC_MAX_CHANNELS][ALAC_MAX_CHANNELS]
Definition: alac_data.c:24
Definition: get_bits.h:54
common internal api header.
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
static int rice_decompress(ALACContext *alac, int32_t *output_buffer, int nb_samples, int bps, int rice_history_mult)
Definition: alac.c:102
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
Generated on Wed May 8 2024 06:52:01 for FFmpeg by
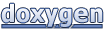