FFmpeg
|
j2kdec.c
Go to the documentation of this file.
481 if (ret = ff_j2k_init_component(comp, codsty, qntsty, s->cbps[compno], s->cdx[compno], s->cdy[compno]))
514 static int decode_packet(J2kDecoderContext *s, J2kCodingStyle *codsty, J2kResLevel *rlevel, int precno,
535 for(cblknx = prec->xi0, cblkno = cblkny * band->cblknx + cblknx; cblknx < prec->xi1; cblknx++, cblkno++, pos++){
549 cblk->nonzerobits = expn[bandno] + numgbits - 1 - tag_tree_decode(s, prec->zerobits + pos, 100);
616 static void decode_sigpass(J2kT1Context *t1, int width, int height, int bpno, int bandno, int bpass_csty_symbol,
713 static int decode_cblk(J2kDecoderContext *s, J2kCodingStyle *codsty, J2kT1Context *t1, J2kCblk *cblk,
806 yy0 = bandno == 0 ? 0 : comp->reslevel[reslevelno-1].coord[1][1] - comp->reslevel[reslevelno-1].coord[1][0];
808 yy1 = FFMIN(ff_j2k_ceildiv(band->coord[1][0] + 1, band->codeblock_height) * band->codeblock_height,
820 xx1 = FFMIN(ff_j2k_ceildiv(band->coord[0][0] + 1, band->codeblock_width) * band->codeblock_width,
983 av_log(s->avctx, AV_LOG_ERROR, "unsupported marker 0x%.4X at pos 0x%x\n", marker, bytestream2_tell(&s->g) - 4);
Definition: j2kdec.c:50
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
Definition: bytestream.h:32
Definition: j2k_dwt.h:37
static int getnpasses(J2kDecoderContext *s)
read the number of coding passes
Definition: j2kdec.c:488
static int get_qcc(J2kDecoderContext *s, int n, J2kQuantStyle *q, uint8_t *properties)
get quantization parameters for a component in the whole image on in a particular tile ...
Definition: j2kdec.c:423
Definition: j2k.h:103
Definition: j2k.h:115
static void decode_clnpass(J2kDecoderContext *s, J2kT1Context *t1, int width, int height, int bpno, int bandno, int seg_symbols)
Definition: j2kdec.c:663
static av_always_inline void bytestream2_init(GetByteContext *g, const uint8_t *buf, int buf_size)
Definition: bytestream.h:130
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
Definition: j2k_dwt.h:36
static av_always_inline unsigned int bytestream2_get_bufferu(GetByteContext *g, uint8_t *dst, unsigned int size)
Definition: bytestream.h:268
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
Definition: af_biquads.c:76
JPEG2000 tables.
static int get_coc(J2kDecoderContext *s, J2kCodingStyle *c, uint8_t *properties)
get coding parameters for a component in the whole image on a particular tile
Definition: j2kdec.c:348
static av_always_inline void bytestream2_skipu(GetByteContext *g, unsigned int size)
Definition: bytestream.h:165
void ff_j2k_set_significant(J2kT1Context *t1, int x, int y, int negative)
Definition: j2k.c:181
int ff_j2k_init_component(J2kComponent *comp, J2kCodingStyle *codsty, J2kQuantStyle *qntsty, int cbps, int dx, int dy)
Definition: j2k.c:202
static int get_qcx(J2kDecoderContext *s, int n, J2kQuantStyle *q)
get common part for QCD and QCC segments
Definition: j2kdec.c:366
static int get_siz(J2kDecoderContext *s)
get sizes and offsets of image, tiles; number of components
Definition: j2kdec.c:205
static int decode_packets(J2kDecoderContext *s, J2kTile *tile)
Definition: j2kdec.c:589
static int get_cod(J2kDecoderContext *s, J2kCodingStyle *c, uint8_t *properties)
get coding parameters for a particular tile or whole image
Definition: j2kdec.c:318
static av_always_inline void bytestream2_skip(GetByteContext *g, unsigned int size)
Definition: bytestream.h:159
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
static av_always_inline unsigned int bytestream2_get_bytes_left(GetByteContext *g)
Definition: bytestream.h:149
Definition: j2kdec.c:43
static int get_cox(J2kDecoderContext *s, J2kCodingStyle *c)
get common part for COD and COC segments
Definition: j2kdec.c:295
external API header
Definition: j2k.h:175
static void decode_sigpass(J2kT1Context *t1, int width, int height, int bpno, int bandno, int bpass_csty_symbol, int vert_causal_ctx_csty_symbol)
Definition: j2kdec.c:616
Definition: j2k.h:128
static int ff_j2k_getnbctxno(int flag, int bandno, int vert_causal_ctx_csty_symbol)
Definition: j2k.h:211
static av_always_inline int bytestream2_tell(GetByteContext *g)
Definition: bytestream.h:183
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
int ff_mqc_decode(MqcState *mqc, uint8_t *cxstate)
returns decoded bit with context cx
Definition: mqcdec.c:81
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
static int get_qcd(J2kDecoderContext *s, int n, J2kQuantStyle *q, uint8_t *properties)
get quantization parameters for a particular tile or a whole image
Definition: j2kdec.c:409
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
static int decode_frame(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: j2kdec.c:1018
Definition: libavcodec/avcodec.h:191
static int tag_tree_decode(J2kDecoderContext *s, J2kTgtNode *node, int threshold)
decode the value stored in node
Definition: j2kdec.c:166
static int decode_packet(J2kDecoderContext *s, J2kCodingStyle *codsty, J2kResLevel *rlevel, int precno, int layno, uint8_t *expn, int numgbits)
Definition: j2kdec.c:514
common internal api header.
common internal and external API header
#define CODEC_CAP_EXPERIMENTAL
Codec is experimental and is thus avoided in favor of non experimental encoders.
Definition: libavcodec/avcodec.h:796
Definition: j2k.h:109
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip please avoid problems through this extra level of scrutiny For cosmetics only commits you should e g by running git config global user name My Name git config global user email my email which is either set in your personal configuration file through git config core editor or set by one of the following environment VISUAL or EDITOR Log messages should be concise but descriptive Explain why you made a what you did will be obvious from the changes themselves most of the time Saying just bug fix or is bad Remember that people of varying skill levels look at and educate themselves while reading through your code Don t include filenames in log Git provides that information Possibly make the commit message have a descriptive first line
Definition: git-howto.txt:153
static av_always_inline int bytestream2_seek(GetByteContext *g, int offset, int whence)
Definition: bytestream.h:203
static void comp(unsigned char *dst, int dst_stride, unsigned char *src, int src_stride, int add)
Definition: eamad.c:71
Definition: avutil.h:143
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
Definition: j2k.h:167
static int decode_cblk(J2kDecoderContext *s, J2kCodingStyle *codsty, J2kT1Context *t1, J2kCblk *cblk, int width, int height, int bandpos)
Definition: j2kdec.c:713
static void decode_refpass(J2kT1Context *t1, int width, int height, int bpno)
Definition: j2kdec.c:643
Generated on Fri Aug 1 2025 06:53:42 for FFmpeg by
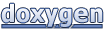