FFmpeg
|
rtpdec_jpeg.c
Go to the documentation of this file.
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
int avio_close_dyn_buf(AVIOContext *s, uint8_t **pbuffer)
Return the written size and a pointer to the buffer.
Definition: aviobuf.c:988
const uint8_t avpriv_mjpeg_bits_ac_luminance[17]
Definition: mjpeg.c:73
Definition: rtpdec.h:119
static void free_frame_if_needed(PayloadContext *jpeg)
Definition: rtpdec_jpeg.c:67
static av_always_inline void bytestream2_init_writer(PutByteContext *p, uint8_t *buf, int buf_size)
Definition: bytestream.h:139
Definition: mjpeg.h:74
const uint8_t avpriv_mjpeg_bits_dc_chrominance[17]
Definition: mjpeg.c:70
const uint8_t avpriv_mjpeg_bits_ac_chrominance[17]
Definition: mjpeg.c:99
MJPEG encoder and decoder.
static int jpeg_create_huffman_table(PutByteContext *p, int table_class, int table_id, const uint8_t *bits_table, const uint8_t *value_table)
Definition: rtpdec_jpeg.c:83
static void jpeg_put_marker(PutByteContext *pbc, int code)
Definition: rtpdec_jpeg.c:102
static av_always_inline int64_t avio_tell(AVIOContext *s)
ftell() equivalent for AVIOContext.
Definition: avio.h:248
Definition: libavcodec/avcodec.h:110
Definition: mjpeg.h:77
void avio_write(AVIOContext *s, const unsigned char *buf, int size)
Definition: aviobuf.c:173
Definition: mjpeg.h:83
RTPDynamicProtocolHandler ff_jpeg_dynamic_handler
Definition: rtpdec_jpeg.c:384
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
Definition: mjpeg.h:43
Definition: mjpeg.h:60
static av_always_inline int bytestream2_tell_p(PutByteContext *p)
Definition: bytestream.h:188
Definition: bytestream.h:36
Definition: mjpeg.h:76
const uint8_t avpriv_mjpeg_bits_dc_luminance[17]
Definition: mjpeg.c:65
static av_always_inline unsigned int bytestream2_put_buffer(PutByteContext *p, const uint8_t *src, unsigned int size)
Definition: bytestream.h:277
static void create_default_qtables(uint8_t *qtables, uint8_t q)
Definition: rtpdec_jpeg.c:199
Definition: mjpeg.h:75
static int jpeg_parse_packet(AVFormatContext *ctx, PayloadContext *jpeg, AVStream *st, AVPacket *pkt, uint32_t *timestamp, const uint8_t *buf, int len, uint16_t seq, int flags)
Definition: rtpdec_jpeg.c:220
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
#define type
static int jpeg_create_header(uint8_t *buf, int size, uint32_t type, uint32_t w, uint32_t h, const uint8_t *qtable, int nb_qtable)
Definition: rtpdec_jpeg.c:108
Main libavformat public API header.
int ff_rtp_finalize_packet(AVPacket *pkt, AVIOContext **dyn_buf, int stream_idx)
Close the dynamic buffer and make a packet from it.
Definition: rtpdec.c:865
const uint8_t avpriv_mjpeg_val_ac_chrominance[]
Definition: mjpeg.c:102
Definition: avutil.h:143
Generated on Wed May 7 2025 06:52:46 for FFmpeg by
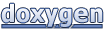