FFmpeg
|
rtpdec.c
Go to the documentation of this file.
316 uint32_t middle_32_bits = s->last_rtcp_ntp_time >> 16; // this is valid, right? do we need to handle 64 bit values special?
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
void ff_rtp_parse_set_crypto(RTPDemuxContext *s, const char *suite, const char *params)
Definition: rtpdec.c:527
RTPDynamicProtocolHandler ff_quicktime_rtp_aud_handler
void ff_rtp_send_punch_packets(URLContext *rtp_handle)
Send a dummy packet on both port pairs to set up the connection state in potential NAT routers...
Definition: rtpdec.c:352
int avio_close_dyn_buf(AVIOContext *s, uint8_t **pbuffer)
Return the written size and a pointer to the buffer.
Definition: aviobuf.c:988
static void rtcp_update_jitter(RTPStatistics *s, uint32_t sent_timestamp, uint32_t arrival_timestamp)
Definition: rtpdec.c:231
int ffurl_write(URLContext *h, const unsigned char *buf, int size)
Write size bytes from buf to the resource accessed by h.
Definition: avio.c:317
static int rtp_parse_packet_internal(RTPDemuxContext *s, AVPacket *pkt, const uint8_t *buf, int len)
Definition: rtpdec.c:573
Definition: rtpdec.h:119
RTPDynamicProtocolHandler ff_mp4a_latm_dynamic_handler
Definition: rtpdec_latm.c:181
void av_register_rtp_dynamic_payload_handlers(void)
Definition: rtpdec.c:61
RTPDynamicProtocolHandler ff_ms_rtp_asf_pfa_handler
static int rtcp_parse_packet(RTPDemuxContext *s, const unsigned char *buf, int len)
Definition: rtpdec.c:122
RTPDynamicProtocolHandler ff_jpeg_dynamic_handler
Definition: rtpdec_jpeg.c:384
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
RTPDynamicProtocolHandler ff_h263_1998_dynamic_handler
Definition: rtpdec_h263.c:99
RTPDynamicProtocolHandler ff_amr_wb_dynamic_handler
Definition: rtpdec_amr.c:201
RTPDynamicProtocolHandler ff_h263_2000_dynamic_handler
Definition: rtpdec_h263.c:107
static int find_missing_packets(RTPDemuxContext *s, uint16_t *first_missing, uint16_t *missing_mask)
Definition: rtpdec.c:390
RTPDemuxContext * ff_rtp_parse_open(AVFormatContext *s1, AVStream *st, int payload_type, int queue_size)
open a new RTP parse context for stream 'st'.
Definition: rtpdec.c:488
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
Definition: libavcodec/avcodec.h:417
Definition: libavcodec/avcodec.h:360
PayloadContext * dynamic_protocol_context
Definition: rtpdec.h:194
int(* need_keyframe)(PayloadContext *context)
Definition: rtpdec.h:138
RTPDynamicProtocolHandler ff_ilbc_dynamic_handler
Definition: rtpdec_ilbc.c:68
int av_packet_from_data(AVPacket *pkt, uint8_t *data, int size)
Initialize a reference-counted packet from av_malloc()ed data.
Definition: avpacket.c:136
int ff_rtsp_next_attr_and_value(const char **p, char *attr, int attr_size, char *value, int value_size)
RTPDynamicProtocolHandler ff_theora_dynamic_handler
Definition: rtpdec_xiph.c:390
static int rtp_parse_queued_packet(RTPDemuxContext *s, AVPacket *pkt)
Definition: rtpdec.c:704
bitstream reader API header.
Definition: rtpdec.h:151
void avio_write(AVIOContext *s, const unsigned char *buf, int size)
Definition: aviobuf.c:173
int64_t av_rescale_q(int64_t a, AVRational bq, AVRational cq)
Rescale a 64-bit integer by 2 rational numbers.
Definition: mathematics.c:130
int av_new_packet(AVPacket *pkt, int size)
Allocate the payload of a packet and initialize its fields with default values.
Definition: avpacket.c:73
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
static void rtp_init_sequence(RTPStatistics *s, uint16_t seq)
Definition: rtpdec.c:171
FFmpeg currently uses a custom build this text attempts to document some of its obscure features and options Makefile the full command issued by make and its output will be shown on the screen DESTDIR Destination directory for the install useful to prepare packages or install FFmpeg in cross environments Makefile builds all the libraries and the executables fate Run the fate test suite
Definition: build_system.txt:1
Definition: avutil.h:144
RTPDynamicProtocolHandler ff_vorbis_dynamic_handler
Definition: rtpdec_xiph.c:400
RTPDynamicProtocolHandler ff_ms_rtp_asf_pfv_handler
RTPDynamicProtocolHandler ff_svq3_dynamic_handler
Definition: rtpdec_svq3.c:127
DynamicPayloadPacketHandlerProc parse_packet
Parse handler for this dynamic packet.
Definition: rtpdec.h:137
RTPDynamicProtocolHandler * ff_rtp_handler_find_by_id(int id, enum AVMediaType codec_type)
Definition: rtpdec.c:110
Definition: rtp.h:101
RTPDynamicProtocolHandler ff_qt_rtp_vid_handler
int void avio_flush(AVIOContext *s)
Force flushing of buffered data to the output s.
Definition: aviobuf.c:193
int ff_srtp_decrypt(struct SRTPContext *s, uint8_t *buf, int *lenptr)
Definition: srtp.c:126
int64_t av_rescale(int64_t a, int64_t b, int64_t c)
Rescale a 64-bit integer with rounding to nearest.
Definition: mathematics.c:118
int av_strcasecmp(const char *a, const char *b)
Locale-independent case-insensitive compare.
Definition: avstring.c:212
static RTPDynamicProtocolHandler * rtp_first_dynamic_payload_handler
Definition: rtpdec.c:53
RTPDynamicProtocolHandler ff_h263_rfc2190_dynamic_handler
Definition: rtpdec_h263_rfc2190.c:206
Definition: libavcodec/avcodec.h:394
Definition: libavcodec/avcodec.h:449
void ff_rtp_parse_set_dynamic_protocol(RTPDemuxContext *s, PayloadContext *ctx, RTPDynamicProtocolHandler *handler)
Definition: rtpdec.c:520
int ff_rtp_send_rtcp_feedback(RTPDemuxContext *s, URLContext *fd, AVIOContext *avio)
Definition: rtpdec.c:420
RTPDynamicProtocolHandler ff_g726_16_dynamic_handler
static int rtp_parse_one_packet(RTPDemuxContext *s, AVPacket *pkt, uint8_t **bufptr, int len)
Definition: rtpdec.c:726
static int parse_fmtp(AVStream *stream, PayloadContext *data, char *attr, char *value)
Definition: rtpdec_latm.c:148
void ff_register_dynamic_payload_handler(RTPDynamicProtocolHandler *handler)
Definition: rtpdec.c:55
RTPDynamicProtocolHandler ff_mp4v_es_dynamic_handler
Definition: rtpdec_mpeg4.c:263
Definition: url.h:41
Definition: rtpdec.h:143
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
RTPStatistics statistics
Statistics for this stream (used by RTCP receiver reports)
Definition: rtpdec.h:170
RTPDynamicProtocolHandler ff_qdm2_dynamic_handler
Definition: rtpdec_qdm2.c:312
int64_t ff_rtp_queued_packet_time(RTPDemuxContext *s)
Definition: rtpdec.c:699
int ff_rtp_check_and_send_back_rr(RTPDemuxContext *s, URLContext *fd, AVIOContext *avio, int count)
some rtp servers assume client is dead if they don't hear from them...
Definition: rtpdec.c:249
static void finalize_packet(RTPDemuxContext *s, AVPacket *pkt, uint32_t timestamp)
This was the second switch in rtp_parse packet.
Definition: rtpdec.c:538
RTPDynamicProtocolHandler ff_mpegts_dynamic_handler
Definition: rtpdec_mpegts.c:99
RTPDynamicProtocolHandler ff_g726_32_dynamic_handler
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
RTPDynamicProtocolHandler ff_qt_rtp_aud_handler
RTPDynamicProtocolHandler ff_mpeg_audio_dynamic_handler
Definition: rtpdec_mpeg12.c:58
RTPDynamicProtocolHandler ff_mpeg_video_dynamic_handler
Definition: rtpdec_mpeg12.c:66
Main libavformat public API header.
static RTPDynamicProtocolHandler realmedia_mp3_dynamic_handler
Definition: rtpdec.c:35
RTPDynamicProtocolHandler * ff_rtp_handler_find_by_name(const char *name, enum AVMediaType codec_type)
Definition: rtpdec.c:98
RTPDynamicProtocolHandler ff_g726_40_dynamic_handler
RTPDynamicProtocolHandler ff_qcelp_dynamic_handler
Definition: rtpdec_qcelp.c:222
void av_init_packet(AVPacket *pkt)
Initialize optional fields of a packet with default values.
Definition: avpacket.c:56
int ff_rtp_finalize_packet(AVPacket *pkt, AVIOContext **dyn_buf, int stream_idx)
Close the dynamic buffer and make a packet from it.
Definition: rtpdec.c:865
RTPDynamicProtocolHandler ff_g726_24_dynamic_handler
RTPDynamicProtocolHandler ff_h264_dynamic_handler
Definition: rtpdec_h264.c:390
int ff_srtp_set_crypto(struct SRTPContext *s, const char *suite, const char *params)
Definition: srtp.c:65
int64_t dts
Decompression timestamp in AVStream->time_base units; the time at which the packet is decompressed...
Definition: libavcodec/avcodec.h:1050
int ff_parse_fmtp(AVStream *stream, PayloadContext *data, const char *p, int(*parse_fmtp)(AVStream *stream, PayloadContext *data, char *attr, char *value))
Definition: rtpdec.c:829
unbuffered private I/O API
static void rtp_init_statistics(RTPStatistics *s, uint16_t base_sequence)
Definition: rtpdec.c:160
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: avformat.h:679
RTPDynamicProtocolHandler ff_quicktime_rtp_vid_handler
RTPDynamicProtocolHandler ff_mpeg4_generic_dynamic_handler
Definition: rtpdec_mpeg4.c:271
int ff_rtp_parse_packet(RTPDemuxContext *s, AVPacket *pkt, uint8_t **bufptr, int len)
Parse an RTP or RTCP packet directly sent as a buffer.
Definition: rtpdec.c:809
RTPDynamicProtocolHandler ff_amr_nb_dynamic_handler
Definition: rtpdec_amr.c:191
Definition: rtpdec.h:83
static int rtp_valid_packet_in_sequence(RTPStatistics *s, uint16_t seq)
Definition: rtpdec.c:185
int64_t pts
Presentation timestamp in AVStream->time_base units; the time at which the decompressed packet will b...
Definition: libavcodec/avcodec.h:1044
static void enqueue_packet(RTPDemuxContext *s, uint8_t *buf, int len)
Definition: rtpdec.c:669
Generated on Sat May 17 2025 06:53:40 for FFmpeg by
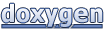