FFmpeg
|
aviobuf.c
Go to the documentation of this file.
832 av_log(s, AV_LOG_DEBUG, "Statistics: %d seeks, %d writeouts\n", s->seek_count, s->writeout_count);
834 av_log(s, AV_LOG_DEBUG, "Statistics: %"PRId64" bytes read, %d seeks\n", s->bytes_read, s->seek_count);
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
AVIOContext * avio_alloc_context(unsigned char *buffer, int buffer_size, int write_flag, void *opaque, int(*read_packet)(void *opaque, uint8_t *buf, int buf_size), int(*write_packet)(void *opaque, uint8_t *buf, int buf_size), int64_t(*seek)(void *opaque, int64_t offset, int whence))
Allocate and initialize an AVIOContext for buffered I/O.
Definition: aviobuf.c:109
static int64_t dyn_buf_seek(void *opaque, int64_t offset, int whence)
Definition: aviobuf.c:940
Buffered I/O operations.
#define GET_UTF8(val, GET_BYTE, ERROR)
Convert a UTF-8 character (up to 4 bytes) to its 32-bit UCS-4 encoded form.
Definition: common.h:296
uint32_t av_crc(const AVCRC *ctx, uint32_t crc, const uint8_t *buffer, size_t length)
Calculate the CRC of a block.
Definition: crc.c:275
void * av_realloc_f(void *ptr, size_t nelem, size_t elsize)
Allocate or reallocate a block of memory.
Definition: mem.c:168
int writeout_count
writeout statistic This field is internal to libavformat and access from outside is not allowed...
Definition: avio.h:148
unsigned char * buf_end
End of the data, may be less than buffer+buffer_size if the read function returned less data than req...
Definition: avio.h:85
int ffurl_write(URLContext *h, const unsigned char *buf, int size)
Write size bytes from buf to the resource accessed by h.
Definition: avio.c:317
static int write_packet(AVFormatContext *s, AVPacket *pkt)
Definition: libavformat/assenc.c:63
int ffio_read_partial(AVIOContext *s, unsigned char *buf, int size)
Read size bytes from AVIOContext into buf.
Definition: aviobuf.c:526
int avio_closep(AVIOContext **s)
Close the resource accessed by the AVIOContext *s, free it and set the pointer pointing to it to NULL...
Definition: aviobuf.c:839
static const AVClass * ffio_url_child_class_next(const AVClass *prev)
Definition: aviobuf.c:50
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
int avio_put_str(AVIOContext *s, const char *str)
Write a NULL-terminated string.
Definition: aviobuf.c:307
const char * class_name
The name of the class; usually it is the same name as the context structure type to which the AVClass...
Definition: log.h:55
Public dictionary API.
#define av_assert2(cond)
assert() equivalent, that does lie in speed critical code.
Definition: avassert.h:63
AVOptions.
const AVCRC * av_crc_get_table(AVCRCId crc_id)
Get an initialized standard CRC table.
Definition: crc.c:261
void avio_flush(AVIOContext *s)
Force flushing of buffered data to the output s.
Definition: aviobuf.c:193
int ffio_init_context(AVIOContext *s, unsigned char *buffer, int buffer_size, int write_flag, void *opaque, int(*read_packet)(void *opaque, uint8_t *buf, int buf_size), int(*write_packet)(void *opaque, uint8_t *buf, int buf_size), int64_t(*seek)(void *opaque, int64_t offset, int whence))
Definition: aviobuf.c:71
int64_t bytes_read
Bytes read statistic This field is internal to libavformat and access from outside is not allowed...
Definition: avio.h:136
Definition: dict.c:28
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
static int dyn_packet_buf_write(void *opaque, uint8_t *buf, int buf_size)
Definition: aviobuf.c:925
simple assert() macros that are a bit more flexible than ISO C assert().
#define SHORT_SEEK_THRESHOLD
Do seeks within this distance ahead of the current buffer by skipping data instead of calling the pro...
Definition: aviobuf.c:42
int direct
avio_read and avio_write should if possible be satisfied directly instead of going through a buffer...
Definition: avio.h:130
int seek_count
seek statistic This field is internal to libavformat and access from outside is not allowed...
Definition: avio.h:142
int avio_pause(AVIOContext *s, int pause)
Pause and resume playing - only meaningful if using a network streaming protocol (e.g.
Definition: aviobuf.c:859
#define FF_INPUT_BUFFER_PADDING_SIZE
Required number of additionally allocated bytes at the end of the input bitstream for decoding...
Definition: libavcodec/avcodec.h:561
int(* read_packet)(void *opaque, uint8_t *buf, int buf_size)
Definition: avio.h:91
int seekable
A combination of AVIO_SEEKABLE_ flags or 0 when the stream is not seekable.
Definition: avio.h:117
int64_t avio_seek(AVIOContext *s, int64_t offset, int whence)
fseek() equivalent for AVIOContext.
Definition: aviobuf.c:199
#define av_assert1(cond)
assert() equivalent, that does not lie in speed critical code.
Definition: avassert.h:53
int avio_close_dyn_buf(AVIOContext *s, uint8_t **pbuffer)
Return the written size and a pointer to the buffer.
Definition: aviobuf.c:988
struct DynBuffer DynBuffer
static int dyn_buf_write(void *opaque, uint8_t *buf, int buf_size)
Definition: aviobuf.c:895
int ff_get_line(AVIOContext *s, char *buf, int maxlen)
Read a whole line of text from AVIOContext.
Definition: aviobuf.c:618
int avio_read(AVIOContext *s, unsigned char *buf, int size)
Read size bytes from AVIOContext into buf.
Definition: aviobuf.c:478
int ffio_open_dyn_packet_buf(AVIOContext **s, int max_packet_size)
Open a write only packetized memory stream with a maximum packet size of 'max_packet_size'.
Definition: aviobuf.c:981
int avio_open(AVIOContext **s, const char *filename, int flags)
Create and initialize a AVIOContext for accessing the resource indicated by url.
Definition: aviobuf.c:799
Definition: crc.h:34
int ffio_rewind_with_probe_data(AVIOContext *s, unsigned char **bufp, int buf_size)
Rewind the AVIOContext using the specified buffer containing the first buf_size bytes of the file...
Definition: aviobuf.c:755
int avio_close(AVIOContext *s)
Close the resource accessed by the AVIOContext s and free it.
Definition: aviobuf.c:821
int(* write_packet)(void *opaque, uint8_t *buf, int buf_size)
Definition: avio.h:92
Definition: url.h:41
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
void avio_write(AVIOContext *s, const unsigned char *buf, int size)
Definition: aviobuf.c:173
#define AVSEEK_FORCE
Oring this flag as into the "whence" parameter to a seek function causes it to seek by any means (lik...
Definition: avio.h:230
#define PUT_UTF16(val, tmp, PUT_16BIT)
Convert a 32-bit Unicode character to its UTF-16 encoded form (2 or 4 bytes).
Definition: common.h:383
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
int ff_get_v_length(uint64_t val)
Get the length in bytes which is needed to store val as v.
Definition: aviobuf.c:335
int avio_open2(AVIOContext **s, const char *filename, int flags, const AVIOInterruptCB *int_cb, AVDictionary **options)
Create and initialize a AVIOContext for accessing the resource indicated by url.
Definition: aviobuf.c:804
int(* read_pause)(void *opaque, int pause)
Pause or resume playback for network streaming protocols - e.g.
Definition: avio.h:106
Main libavformat public API header.
int avio_get_str(AVIOContext *s, int maxlen, char *buf, int buflen)
Read a string from pb into buf.
Definition: aviobuf.c:633
int64_t ffurl_seek(URLContext *h, int64_t pos, int whence)
Change the position that will be used by the next read/write operation on the resource accessed by h...
Definition: avio.c:328
int ffurl_open(URLContext **puc, const char *filename, int flags, const AVIOInterruptCB *int_cb, AVDictionary **options)
Create an URLContext for accessing to the resource indicated by url, and open it. ...
Definition: avio.c:247
unsigned long(* update_checksum)(unsigned long checksum, const uint8_t *buf, unsigned int size)
Definition: avio.h:101
int ffio_fdopen(AVIOContext **s, URLContext *h)
Create and initialize a AVIOContext for accessing the resource referenced by the URLContext h...
Definition: aviobuf.c:694
void ffio_init_checksum(AVIOContext *s, unsigned long(*update_checksum)(unsigned long c, const uint8_t *p, unsigned int len), unsigned long checksum)
Definition: aviobuf.c:457
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
#define AVSEEK_SIZE
Passing this as the "whence" parameter to a seek function causes it to return the filesize without se...
Definition: avio.h:222
void ff_put_v(AVIOContext *bc, uint64_t val)
Put val using a variable number of bytes.
Definition: aviobuf.c:345
static int url_open_dyn_buf_internal(AVIOContext **s, int max_packet_size)
Definition: aviobuf.c:954
int max_packet_size
if non zero, the stream is packetized with this max packet size
Definition: url.h:47
int64_t avio_skip(AVIOContext *s, int64_t offset)
Skip given number of bytes forward.
Definition: aviobuf.c:256
unbuffered private I/O API
int avio_put_str16le(AVIOContext *s, const char *str)
Convert an UTF-8 string to UTF-16LE and write it.
Definition: aviobuf.c:318
unsigned long ff_crc04C11DB7_update(unsigned long checksum, const uint8_t *buf, unsigned int len)
Definition: aviobuf.c:443
int ffurl_read(URLContext *h, unsigned char *buf, int size)
Read up to size bytes from the resource accessed by h, and store the read bytes in buf...
Definition: avio.c:303
int64_t(* read_seek)(void *opaque, int stream_index, int64_t timestamp, int flags)
Seek to a given timestamp in stream with the specified stream_index.
Definition: avio.h:112
Definition: aviobuf.c:888
int64_t avio_seek_time(AVIOContext *s, int stream_index, int64_t timestamp, int flags)
Seek to a given timestamp relative to some component stream.
Definition: aviobuf.c:866
int64_t(* url_read_seek)(URLContext *h, int stream_index, int64_t timestamp, int flags)
Definition: url.h:82
Generated on Wed May 8 2024 06:52:02 for FFmpeg by
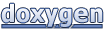