FFmpeg
|
libavcodec/pcm.c
Go to the documentation of this file.
607 PCM_CODEC (PCM_S16BE_PLANAR, AV_SAMPLE_FMT_S16P,pcm_s16be_planar, "PCM signed 16-bit big-endian planar");
609 PCM_CODEC (PCM_S16LE_PLANAR, AV_SAMPLE_FMT_S16P,pcm_s16le_planar, "PCM signed 16-bit little-endian planar");
613 PCM_CODEC (PCM_S24LE_PLANAR, AV_SAMPLE_FMT_S32P,pcm_s24le_planar, "PCM signed 24-bit little-endian planar");
616 PCM_CODEC (PCM_S32LE_PLANAR, AV_SAMPLE_FMT_S32P,pcm_s32le_planar, "PCM signed 32-bit little-endian planar");
Definition: libavcodec/avcodec.h:312
Definition: libavcodec/avcodec.h:315
Definition: libavcodec/avcodec.h:303
Definition: libavcodec/avcodec.h:301
#define DECODE_PLANAR(size, endian, src, dst, n, shift, offset)
Definition: libavcodec/pcm.c:284
static int pcm_encode_frame(AVCodecContext *avctx, AVPacket *avpkt, const AVFrame *frame, int *got_packet_ptr)
Definition: libavcodec/pcm.c:93
int bits_per_raw_sample
Bits per sample/pixel of internal libavcodec pixel/sample format.
Definition: libavcodec/avcodec.h:2553
int block_align
number of bytes per packet if constant and known or 0 Used by some WAV based audio codecs...
Definition: libavcodec/avcodec.h:1898
Macro definitions for various function/variable attributes.
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
Definition: libavcodec/pcm.c:232
Definition: libavcodec/avcodec.h:313
int bits_per_coded_sample
bits per sample/pixel from the demuxer (needed for huffyuv).
Definition: libavcodec/avcodec.h:2546
Definition: libavcodec/avcodec.h:318
static int pcm_decode_frame(AVCodecContext *avctx, void *data, int *got_frame_ptr, AVPacket *avpkt)
Definition: libavcodec/pcm.c:296
Definition: libavcodec/avcodec.h:306
int av_get_bits_per_sample(enum AVCodecID codec_id)
Return codec bits per sample.
Definition: libavcodec/utils.c:2690
#define DECODE(size, endian, src, dst, n, shift, offset)
Read PCM samples macro.
Definition: libavcodec/pcm.c:277
#define ENCODE_PLANAR(type, endian, dst, n, shift, offset)
Definition: libavcodec/pcm.c:82
external API header
static av_cold int pcm_encode_close(AVCodecContext *avctx)
Definition: libavcodec/pcm.c:58
AVFrame * avcodec_alloc_frame(void)
Allocate an AVFrame and set its fields to default values.
Definition: libavcodec/utils.c:951
#define ENCODE(type, endian, src, dst, n, shift, offset)
Write PCM samples macro.
Definition: libavcodec/pcm.c:75
static av_cold int pcm_encode_init(AVCodecContext *avctx)
Definition: libavcodec/pcm.c:34
Definition: libavcodec/avcodec.h:314
Definition: libavcodec/avcodec.h:302
Definition: libavcodec/avcodec.h:321
static av_always_inline unsigned int bytestream_get_buffer(const uint8_t **b, uint8_t *dst, unsigned int size)
Definition: bytestream.h:328
Definition: libavcodec/avcodec.h:300
int ff_alloc_packet2(AVCodecContext *avctx, AVPacket *avpkt, int size)
Check AVPacket size and/or allocate data.
Definition: libavcodec/utils.c:1377
Definition: libavcodec/avcodec.h:304
int frame_size
Number of samples per channel in an audio frame.
Definition: libavcodec/avcodec.h:1881
struct PCMDecode PCMDecode
Definition: libavcodec/avcodec.h:305
Definition: libavcodec/avcodec.h:309
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
Definition: libavcodec/avcodec.h:319
Definition: libavcodec/avcodec.h:324
static av_cold int pcm_decode_init(AVCodecContext *avctx)
Definition: libavcodec/pcm.c:236
Definition: libavcodec/avcodec.h:311
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
Definition: libavcodec/avcodec.h:322
Definition: libavcodec/avcodec.h:320
common internal api header.
Definition: libavcodec/avcodec.h:310
Definition: libavcodec/avcodec.h:299
static av_always_inline void bytestream_put_buffer(uint8_t **b, const uint8_t *src, unsigned int size)
Definition: bytestream.h:337
Definition: libavcodec/avcodec.h:316
Filter the word “frame” indicates either a video frame or a group of audio samples
Definition: filter_design.txt:2
Definition: libavcodec/avcodec.h:307
enum AVSampleFormat * sample_fmts
array of supported sample formats, or NULL if unknown, array is terminated by -1
Definition: libavcodec/avcodec.h:2877
Definition: libavcodec/avcodec.h:308
Generated on Fri Aug 1 2025 06:53:45 for FFmpeg by
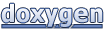