FFmpeg
|
tiff.c
Go to the documentation of this file.
193 ap = av_strdup(search_keyval(ff_tiff_proj_cs_type_codes, FF_ARRAY_ELEMS(ff_tiff_proj_cs_type_codes), val));
197 ap = av_strdup(search_keyval(ff_tiff_projection_codes, FF_ARRAY_ELEMS(ff_tiff_projection_codes), val));
int ff_lzw_decode(LZWState *p, uint8_t *buf, int len)
Decode given number of bytes NOTE: the algorithm here is inspired from the LZW GIF decoder written by...
Definition: lzw.c:170
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
Definition: bytestream.h:32
const TiffGeoTagKeyName ff_tiff_projection_codes[]
Definition: tiff_data.c:1497
Definition: tiff.h:62
Definition: tiff.h:103
Definition: tiff.h:96
static int add_doubles_metadata(int count, const char *name, const char *sep, TiffContext *s, AVFrame *frame)
Definition: tiff.c:268
static int tiff_unpack_strip(TiffContext *s, uint8_t *dst, int stride, const uint8_t *src, int size, int lines)
Definition: tiff.c:414
misc image utilities
TIFF tables.
void avcodec_set_dimensions(AVCodecContext *s, int width, int height)
Definition: libavcodec/utils.c:177
static int add_string_metadata(int count, const char *name, TiffContext *s, AVFrame *frame)
Definition: tiff.c:321
static const char * search_keyval(const TiffGeoTagKeyName *keys, int n, int id)
Definition: tiff.c:139
Definition: lzw.h:39
static av_always_inline void bytestream2_init(GetByteContext *g, const uint8_t *buf, int buf_size)
Definition: bytestream.h:130
void av_fast_padded_malloc(void *ptr, unsigned int *size, size_t min_size)
Same behaviour av_fast_malloc but the buffer has additional FF_INPUT_BUFFER_PADDING_SIZE at the end w...
Definition: libavcodec/utils.c:101
static av_always_inline unsigned int bytestream2_get_bufferu(GetByteContext *g, uint8_t *dst, unsigned int size)
Definition: bytestream.h:268
static int decode_frame(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: tiff.c:1035
Macro definitions for various function/variable attributes.
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
Definition: tiff.h:51
Definition: musixmatch-master/docs/conf.py:1
packed RGB 16:16:16, 48bpp, 16R, 16G, 16B, the 2-byte value for each R/G/B component is stored as lit...
Definition: pixfmt.h:112
Definition: tiff.h:118
Definition: tiff.h:175
packed RGBA 16:16:16:16, 64bpp, 16R, 16G, 16B, 16A, the 2-byte value for each R/G/B/A component is st...
Definition: pixfmt.h:209
Definition: tiff.h:77
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
Definition: tiff.h:94
static int add_metadata(int count, int type, const char *name, const char *sep, TiffContext *s, AVFrame *frame)
Definition: tiff.c:340
Definition: tiff.h:112
Definition: tiff.h:83
Definition: tiff.h:82
Definition: tiff.h:123
static av_always_inline int bytestream2_size(GetByteContext *g)
Definition: bytestream.h:193
Definition: tiff.h:44
Definition: tiff.h:132
Definition: tiff.h:49
Definition: tiff.h:38
Definition: tiff.h:70
Definition: tiff.h:43
Definition: tiff.h:102
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
AVDictionary ** avpriv_frame_get_metadatap(AVFrame *frame)
Definition: frame.c:49
Definition: tiff.h:95
Definition: tiff.h:42
TIFF data tables.
static const uint8_t type_sizes[14]
sizes of various TIFF field types (string size = 100)
Definition: tiff.h:171
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: tiff.h:58
struct TiffContext TiffContext
static av_always_inline unsigned int bytestream2_get_bytes_left(GetByteContext *g)
Definition: bytestream.h:149
Definition: tiff.h:136
Definition: tiff.h:138
int ff_ccitt_unpack(AVCodecContext *avctx, const uint8_t *src, int srcsize, uint8_t *dst, int height, int stride, enum TiffCompr compr, int opts)
unpack data compressed with CCITT Group 3 1/2-D or Group 4 method
Definition: faxcompr.c:277
external API header
Definition: tiff.h:80
Definition: tiff.h:47
#define FF_INPUT_BUFFER_PADDING_SIZE
Required number of additionally allocated bytes at the end of the input bitstream for decoding...
Definition: libavcodec/avcodec.h:561
const TiffGeoTagKeyName ff_tiff_proj_cs_type_codes[]
Definition: tiff_data.c:516
int av_image_check_size(unsigned int w, unsigned int h, int log_offset, void *log_ctx)
Check if the given dimension of an image is valid, meaning that all bytes of the image can be address...
Definition: imgutils.c:231
Definition: tiff.h:65
Definition: tiff.h:122
#define AV_DICT_DONT_STRDUP_VAL
Take ownership of a value that's been allocated with av_malloc() and chilren.
Definition: dict.h:72
Definition: tiff.h:59
Definition: tiff.h:120
Definition: tiff.h:158
Definition: tiff.h:79
Definition: tiff.h:54
Definition: tiff.h:55
static av_always_inline int bytestream2_tell(GetByteContext *g)
Definition: bytestream.h:183
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
Definition: tiff.h:97
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
Definition: tiff.h:45
Definition: tiff.h:66
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
Definition: tiff.h:101
Definition: tiff.h:81
int av_dict_set(AVDictionary **pm, const char *key, const char *value, int flags)
Set the given entry in *pm, overwriting an existing entry.
Definition: dict.c:62
Definition: tiff.h:161
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
Definition: tiff.h:134
static int add_shorts_metadata(int count, const char *name, const char *sep, TiffContext *s, AVFrame *frame)
Definition: tiff.c:295
Definition: tiff.h:78
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
#define type
Definition: tiff.h:64
Definition: tiff.h:124
Definition: tiff.h:126
Definition: tiff.h:69
Definition: libavcodec/avcodec.h:199
LZW decoding routines.
Y , 1bpp, 0 is black, 1 is white, in each byte pixels are ordered from the msb to the lsb...
Definition: pixfmt.h:78
Definition: tiff.h:41
common internal api header.
packed RGB 16:16:16, 48bpp, 16R, 16G, 16B, the 2-byte value for each R/G/B component is stored as big...
Definition: pixfmt.h:111
Definition: tiff.c:44
static void av_always_inline horizontal_fill(unsigned int bpp, uint8_t *dst, int usePtr, const uint8_t *src, uint8_t c, int width, int offset)
Definition: tiff.c:374
int ff_lzw_decode_init(LZWState *p, int csize, const uint8_t *buf, int buf_size, int mode)
Initialize LZW decoder.
Definition: lzw.c:131
#define RET_GEOKEY_VAL(TYPE, array)
Definition: tiff.h:117
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip please avoid problems through this extra level of scrutiny For cosmetics only commits you should e g by running git config global user name My Name git config global user email my email which is either set in your personal configuration file through git config core editor or set by one of the following environment VISUAL or EDITOR Log messages should be concise but descriptive Explain why you made a what you did will be obvious from the changes themselves most of the time Saying just bug fix or is bad Remember that people of varying skill levels look at and educate themselves while reading through your code Don t include filenames in log Git provides that information Possibly make the commit message have a descriptive first line
Definition: git-howto.txt:153
Definition: tiff.h:183
static av_always_inline int bytestream2_seek(GetByteContext *g, int offset, int whence)
Definition: bytestream.h:203
Definition: tiff.h:48
Definition: tiff.h:68
Definition: tiff.h:39
Definition: tiff.h:50
Definition: tiff.h:128
Definition: avutil.h:143
Definition: tiff.h:104
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
Definition: tiff.h:61
Definition: tiff.h:137
Definition: tiff.h:89
#define ADD_METADATA(count, name, sep)
Definition: tiff.h:71
CCITT Fax Group 3 and 4 decompression.
packed RGBA 16:16:16:16, 64bpp, 16R, 16G, 16B, 16A, the 2-byte value for each R/G/B/A component is st...
Definition: pixfmt.h:210
Generated on Tue Jul 8 2025 06:56:53 for FFmpeg by
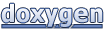