FFmpeg
|
TIFF image decoder. More...
#include "avcodec.h"
#include "bytestream.h"
#include "config.h"
#include "lzw.h"
#include "tiff.h"
#include "tiff_data.h"
#include "faxcompr.h"
#include "internal.h"
#include "mathops.h"
#include "libavutil/attributes.h"
#include "libavutil/intreadwrite.h"
#include "libavutil/imgutils.h"
#include "libavutil/avstring.h"
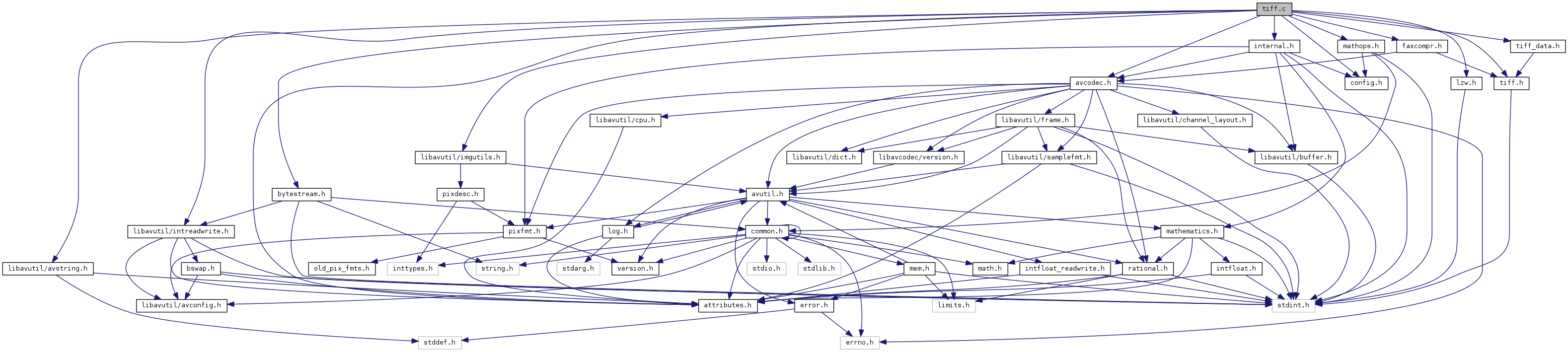
Go to the source code of this file.
Data Structures | |
struct | TiffContext |
Macros | |
#define | RET_GEOKEY(TYPE, array, element) |
#define | RET_GEOKEY_VAL(TYPE, array) |
#define | ADD_METADATA(count, name, sep) |
Typedefs | |
typedef struct TiffContext | TiffContext |
Functions | |
static unsigned | tget_short (GetByteContext *gb, int le) |
static unsigned | tget_long (GetByteContext *gb, int le) |
static double | tget_double (GetByteContext *gb, int le) |
static unsigned | tget (GetByteContext *gb, int type, int le) |
static void | free_geotags (TiffContext *const s) |
static const char * | get_geokey_name (int key) |
static int | get_geokey_type (int key) |
static int | cmp_id_key (const void *id, const void *k) |
static const char * | search_keyval (const TiffGeoTagKeyName *keys, int n, int id) |
static char * | get_geokey_val (int key, int val) |
static char * | doubles2str (double *dp, int count, const char *sep) |
static char * | shorts2str (int16_t *sp, int count, const char *sep) |
static int | add_doubles_metadata (int count, const char *name, const char *sep, TiffContext *s, AVFrame *frame) |
static int | add_shorts_metadata (int count, const char *name, const char *sep, TiffContext *s, AVFrame *frame) |
static int | add_string_metadata (int count, const char *name, TiffContext *s, AVFrame *frame) |
static int | add_metadata (int count, int type, const char *name, const char *sep, TiffContext *s, AVFrame *frame) |
static void av_always_inline | horizontal_fill (unsigned int bpp, uint8_t *dst, int usePtr, const uint8_t *src, uint8_t c, int width, int offset) |
static int | tiff_unpack_strip (TiffContext *s, uint8_t *dst, int stride, const uint8_t *src, int size, int lines) |
static int | init_image (TiffContext *s, AVFrame *frame) |
static int | tiff_decode_tag (TiffContext *s, AVFrame *frame) |
static int | decode_frame (AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt) |
static av_cold int | tiff_init (AVCodecContext *avctx) |
static av_cold int | tiff_end (AVCodecContext *avctx) |
Variables | |
AVCodec | ff_tiff_decoder |
Detailed Description
TIFF image decoder.
Definition in file tiff.c.
Macro Definition Documentation
Referenced by tiff_decode_tag().
#define RET_GEOKEY | ( | TYPE, | |
array, | |||
element | |||
) |
Definition at line 109 of file tiff.c.
Referenced by get_geokey_name(), and get_geokey_type().
#define RET_GEOKEY_VAL | ( | TYPE, | |
array | |||
) |
Referenced by get_geokey_val().
Typedef Documentation
typedef struct TiffContext TiffContext |
Function Documentation
|
static |
Definition at line 268 of file tiff.c.
Referenced by add_metadata().
|
static |
|
static |
Definition at line 295 of file tiff.c.
Referenced by add_metadata().
|
static |
Definition at line 321 of file tiff.c.
Referenced by add_metadata().
Definition at line 134 of file tiff.c.
Referenced by search_keyval().
|
static |
|
static |
Definition at line 216 of file tiff.c.
Referenced by add_doubles_metadata(), and tiff_decode_tag().
|
static |
Definition at line 99 of file tiff.c.
Referenced by decode_frame(), and tiff_end().
|
static |
Definition at line 114 of file tiff.c.
Referenced by decode_frame().
|
static |
Definition at line 124 of file tiff.c.
Referenced by decode_frame().
|
static |
Definition at line 148 of file tiff.c.
Referenced by tiff_decode_tag().
|
static |
Definition at line 374 of file tiff.c.
Referenced by tiff_unpack_strip().
|
static |
Definition at line 599 of file tiff.c.
Referenced by decode_frame().
|
static |
Definition at line 139 of file tiff.c.
Referenced by get_geokey_val().
|
static |
Definition at line 242 of file tiff.c.
Referenced by add_shorts_metadata().
|
static |
Definition at line 89 of file tiff.c.
Referenced by decode_frame(), and tiff_decode_tag().
|
static |
Definition at line 83 of file tiff.c.
Referenced by add_doubles_metadata(), and tiff_decode_tag().
|
static |
Definition at line 77 of file tiff.c.
Referenced by decode_frame(), tget(), and tiff_decode_tag().
|
static |
Definition at line 71 of file tiff.c.
Referenced by add_shorts_metadata(), decode_frame(), tget(), and tiff_decode_tag().
|
static |
Definition at line 659 of file tiff.c.
Referenced by decode_frame().
|
static |
|
static |
|
static |
Definition at line 414 of file tiff.c.
Referenced by decode_frame().
Variable Documentation
AVCodec ff_tiff_decoder |
Generated on Wed May 8 2024 06:52:21 for FFmpeg by
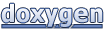