FFmpeg
|
mjpegenc.c
Go to the documentation of this file.
508 pic.data[i] += (pic.linesize[i] * (s->mjpeg_vsample[i] * (8 * s->mb_height -((s->height/V_MAX)&7)) - 1 ));
Definition: mjpeg.h:115
Definition: start.py:1
void ff_mjpeg_encode_mb(MpegEncContext *s, int16_t block[6][64])
Definition: mjpegenc.c:457
Definition: mjpegenc.h:40
Definition: libavcodec/avcodec.h:210
Definition: pixfmt.h:67
Definition: put_bits.h:41
planar YUV 4:4:4, 24bpp, (1 Cr & Cb sample per 1x1 Y samples)
Definition: pixfmt.h:73
const uint8_t avpriv_mjpeg_bits_ac_luminance[17]
Definition: mjpeg.c:73
MJPEG encoder.
AVRational sample_aspect_ratio
sample aspect ratio (0 if unknown) That is the width of a pixel divided by the height of the pixel...
Definition: libavcodec/avcodec.h:1505
Definition: libavcodec/avcodec.h:112
Definition: mjpeg.h:74
const uint8_t avpriv_mjpeg_bits_dc_chrominance[17]
Definition: mjpeg.c:70
mpegvideo header.
const uint8_t avpriv_mjpeg_bits_ac_chrominance[17]
Definition: mjpeg.c:99
MJPEG encoder and decoder.
uint16_t huff_code_dc_chrominance[12]
Definition: mjpegenc.h:44
static void encode_block(MpegEncContext *s, int16_t *block, int n)
Definition: mjpegenc.c:399
static int amv_encode_picture(AVCodecContext *avctx, AVPacket *pkt, const AVFrame *pic_arg, int *got_packet)
Definition: mjpegenc.c:494
void ff_mjpeg_build_huffman_codes(uint8_t *huff_size, uint16_t *huff_code, const uint8_t *bits_table, const uint8_t *val_table)
Definition: mjpeg.c:127
planar YUV 4:2:2, 16bpp, full scale (JPEG), deprecated in favor of PIX_FMT_YUV422P and setting color_...
Definition: pixfmt.h:81
#define CODEC_FLAG_BITEXACT
Use only bitexact stuff (except (I)DCT).
Definition: libavcodec/avcodec.h:715
Definition: libavcodec/avcodec.h:110
Definition: mjpeg.h:77
Definition: mjpeg.h:83
uint16_t huff_code_ac_chrominance[256]
Definition: mjpegenc.h:49
static uint8_t * put_bits_ptr(PutBitContext *s)
Return the pointer to the byte where the bitstream writer will put the next bit.
Definition: put_bits.h:199
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
Definition: mjpeg.h:43
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
int active_thread_type
Which multithreading methods are in use by the codec.
Definition: libavcodec/avcodec.h:2594
Definition: mjpeg.h:60
uint8_t huff_size_ac_chrominance[256]
Definition: mjpegenc.h:48
static void put_bits(J2kEncoderContext *s, int val, int n)
put n times val bit
Definition: j2kenc.c:160
external API header
planar YUV 4:2:2, 16bpp, (1 Cr & Cb sample per 2x1 Y samples)
Definition: pixfmt.h:72
static void skip_put_bytes(PutBitContext *s, int n)
Skip the given number of bytes.
Definition: put_bits.h:208
void ff_mjpeg_encode_picture_header(MpegEncContext *s)
Definition: mjpegenc.c:204
#define av_assert1(cond)
assert() equivalent, that does not lie in speed critical code.
Definition: avassert.h:53
Definition: mjpeg.h:76
planar YUV 4:2:0, 12bpp, full scale (JPEG), deprecated in favor of PIX_FMT_YUV420P and setting color_...
Definition: pixfmt.h:80
const uint8_t avpriv_mjpeg_bits_dc_luminance[17]
Definition: mjpeg.c:65
Definition: mjpeg.h:46
Definition: mjpeg.h:75
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
struct MpegEncContext * thread_context[MAX_THREADS]
Definition: mpegvideo.h:318
#define FF_THREAD_SLICE
Decode more than one part of a single frame at once.
Definition: libavcodec/avcodec.h:2587
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
FIXME Range Coding of cr are mx and my are Motion Vector top and top right vectors is used as motion vector prediction the used motion vector is the sum of the predictor and(mvx_diff, mvy_diff)*mv_scale Intra DC Predicton block[y][x] dc[1]
Definition: snow.txt:392
void ff_mjpeg_encode_picture_trailer(MpegEncContext *s)
Definition: mjpegenc.c:368
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
static int put_huffman_table(MpegEncContext *s, int table_class, int table_id, const uint8_t *bits_table, const uint8_t *value_table)
Definition: mjpegenc.c:87
planar YUV 4:2:0, 12bpp, (1 Cr & Cb sample per 2x2 Y samples)
Definition: pixfmt.h:68
#define CODEC_CAP_SLICE_THREADS
Codec supports slice-based (or partition-based) multithreading.
Definition: libavcodec/avcodec.h:814
static void flush_put_bits(PutBitContext *s)
Pad the end of the output stream with zeros.
Definition: put_bits.h:81
#define CODEC_CAP_FRAME_THREADS
Codec supports frame-level multithreading.
Definition: libavcodec/avcodec.h:810
Definition: mjpeg.h:65
planar YUV 4:4:4, 24bpp, full scale (JPEG), deprecated in favor of PIX_FMT_YUV444P and setting color_...
Definition: pixfmt.h:82
const uint8_t avpriv_mjpeg_val_ac_chrominance[]
Definition: mjpeg.c:102
Definition: mjpeg.h:79
void ff_mjpeg_encode_dc(MpegEncContext *s, int val, uint8_t *huff_size, uint16_t *huff_code)
Definition: mjpegenc.c:377
void avpriv_put_string(PutBitContext *pb, const char *string, int terminate_string)
Put the string string in the bitstream.
Definition: bitstream.c:51
Definition: avutil.h:143
int ff_MPV_encode_picture(AVCodecContext *avctx, AVPacket *pkt, AVFrame *frame, int *got_packet)
Definition: mpegvideo_enc.c:1443
Generated on Tue Jan 21 2025 06:52:24 for FFmpeg by
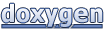