FFmpeg
|
latmenc.c
Go to the documentation of this file.
44 offsetof(LATMContext, mod), AV_OPT_TYPE_INT, {.i64 = 0x0014}, 0x0001, 0xffff, AV_OPT_FLAG_ENCODING_PARAM},
156 av_log(s, AV_LOG_ERROR, "ADTS header detected - ADTS will not be incorrectly muxed into LATM\n");
Definition: libavcodec/avcodec.h:100
static int latm_decode_extradata(LATMContext *ctx, uint8_t *buf, int size)
Definition: latmenc.c:55
Definition: put_bits.h:41
Definition: opt.h:222
static int write_packet(AVFormatContext *s, AVPacket *pkt)
Definition: libavformat/assenc.c:63
void avpriv_copy_bits(PutBitContext *pb, const uint8_t *src, int length)
Copy the content of src to the bitstream.
Definition: bitstream.c:61
void avpriv_align_put_bits(PutBitContext *s)
Pad the bitstream with zeros up to the next byte boundary.
Definition: bitstream.c:46
const char * class_name
The name of the class; usually it is the same name as the context structure type to which the AVClass...
Definition: log.h:55
AVOptions.
Definition: libavcodec/avcodec.h:383
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
bitstream reader API header.
void avio_write(AVIOContext *s, const unsigned char *buf, int size)
Definition: aviobuf.c:173
#define AV_OPT_FLAG_ENCODING_PARAM
a generic parameter which can be set by the user for muxing or encoding
Definition: opt.h:281
Definition: libavcodec/aacdec.c:2719
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
static void put_bits(J2kEncoderContext *s, int val, int n)
put n times val bit
Definition: j2kenc.c:160
external API header
Definition: mpeg4audio.h:29
int ff_raw_write_packet(AVFormatContext *s, AVPacket *pkt)
Definition: libavformat/rawenc.c:26
static int latm_write_packet(AVFormatContext *s, AVPacket *pkt)
Definition: latmenc.c:144
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
Definition: libavcodec/avcodec.h:431
Main libavformat public API header.
Definition: get_bits.h:54
static void flush_put_bits(PutBitContext *s)
Pad the end of the output stream with zeros.
Definition: put_bits.h:81
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
Definition: avformat.h:377
static void init_put_bits(PutBitContext *s, uint8_t *buffer, int buffer_size)
Initialize the PutBitContext s.
Definition: put_bits.h:54
int avpriv_mpeg4audio_get_config(MPEG4AudioConfig *c, const uint8_t *buf, int bit_size, int sync_extension)
Parse MPEG-4 systems extradata to retrieve audio configuration.
Definition: mpeg4audio.c:81
static void latm_write_frame_header(AVFormatContext *s, PutBitContext *bs)
Definition: latmenc.c:99
int avpriv_copy_pce_data(PutBitContext *pb, GetBitContext *gb)
Definition: mpeg4audio.c:161
bitstream writer API
Generated on Wed Apr 16 2025 06:53:16 for FFmpeg by
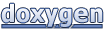