FFmpeg
|
cavs.c
Go to the documentation of this file.
void(* intra_pred_c[7])(uint8_t *d, uint8_t *top, uint8_t *left, int stride)
Definition: cavs.h:228
Definition: cavs.h:95
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
av_cold void ff_dsputil_init(DSPContext *c, AVCodecContext *avctx)
Definition: dsputil.c:2675
Definition: cavs.h:126
void(* cavs_filter_cv)(uint8_t *pix, int stride, int alpha, int beta, int tc, int bs1, int bs2)
Definition: cavsdsp.h:33
Definition: cavs.h:127
static void intra_pred_lp_top(uint8_t *d, uint8_t *top, uint8_t *left, int stride)
Definition: cavs.c:333
Definition: cavs.h:107
void ff_cavs_init_mb(AVSContext *h)
initialise predictors for motion vectors and intra prediction
Definition: cavs.c:592
Definition: cavs.h:113
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining list
Definition: filter_design.txt:23
void(* cavs_filter_lv)(uint8_t *pix, int stride, int alpha, int beta, int tc, int bs1, int bs2)
Definition: cavsdsp.h:31
void(* cavs_filter_lh)(uint8_t *pix, int stride, int alpha, int beta, int tc, int bs1, int bs2)
Definition: cavsdsp.h:32
Definition: cavs.h:85
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
Definition: cavs.h:98
Definition: cavs.h:100
Definition: cavs.h:90
Definition: cavs.h:94
Definition: cavs.h:122
Definition: cavs.h:125
uint8_t * top_border_y
intra prediction is done with un-deblocked samples they are saved here before deblocking the MB ...
Definition: cavs.h:222
av_cold void ff_cavsdsp_init(CAVSDSPContext *c, AVCodecContext *avctx)
Definition: cavsdsp.c:535
cavs_vector mv[2 *4 *3]
mv motion vector cache 0: D3 B2 B3 C2 4: A1 X0 X1 - 8: A3 X2 X3 -
Definition: cavs.h:203
static void intra_pred_plane(uint8_t *d, uint8_t *top, uint8_t *left, int stride)
Definition: cavs.c:274
Definition: cavs.h:133
bitstream reader API header.
void ff_cavs_filter(AVSContext *h, enum cavs_mb mb_type)
in-loop deblocking filter for a single macroblock
Definition: cavs.c:106
Definition: cavs.h:84
void(* cavs_filter_ch)(uint8_t *pix, int stride, int alpha, int beta, int tc, int bs1, int bs2)
Definition: cavsdsp.h:34
Definition: cavs.h:135
void ff_h264chroma_init(H264ChromaContext *c, int bit_depth)
Definition: h264chroma.c:38
void(* qpel_mc_func)(uint8_t *dst, uint8_t *src, ptrdiff_t stride)
Definition: dsputil.h:84
static void scale_mv(AVSContext *h, int *d_x, int *d_y, cavs_vector *src, int distp)
Definition: cavs.c:506
Definition: cavs.h:96
Definition: cavs.h:83
static void mv_pred_median(AVSContext *h, cavs_vector *mvP, cavs_vector *mvA, cavs_vector *mvB, cavs_vector *mvC)
Definition: cavs.c:513
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
int ff_cavs_next_mb(AVSContext *h)
save predictors for later macroblocks and increase macroblock address
Definition: cavs.c:632
static void intra_pred_dc_128(uint8_t *d, uint8_t *top, uint8_t *left, int stride)
Definition: cavs.c:266
void ff_cavs_load_intra_pred_luma(AVSContext *h, uint8_t *top, uint8_t **left, int block)
Definition: cavs.c:178
Definition: cavs.h:124
static void intra_pred_lp_left(uint8_t *d, uint8_t *top, uint8_t *left, int stride)
Definition: cavs.c:325
external API header
Definition: cavs.h:121
void ff_cavs_load_intra_pred_chroma(AVSContext *h)
Definition: cavs.c:229
static void intra_pred_horiz(uint8_t *d, uint8_t *top, uint8_t *left, int stride)
Definition: cavs.c:256
qpel_mc_func put_cavs_qpel_pixels_tab[2][16]
Definition: cavsdsp.h:29
Definition: cavs.h:138
Definition: cavs.h:97
void ff_cavs_init_top_lines(AVSContext *h)
some predictions require data from the top-neighbouring macroblock.
Definition: cavs.c:711
void(* intra_pred_l[8])(uint8_t *d, uint8_t *top, uint8_t *left, int stride)
Definition: cavs.h:227
const cavs_vector ff_cavs_dir_mv
mark block as "no prediction from this direction" e.g.
Definition: cavsdata.c:59
void(* h264_chroma_mc_func)(uint8_t *dst, uint8_t *src, int srcStride, int h, int x, int y)
Definition: h264chroma.h:24
h264_chroma_mc_func avg_h264_chroma_pixels_tab[3]
Definition: h264chroma.h:28
Definition: cavs.h:128
void ff_cavs_mv(AVSContext *h, enum cavs_mv_loc nP, enum cavs_mv_loc nC, enum cavs_mv_pred mode, enum cavs_block size, int ref)
Definition: cavs.c:539
Definition: cavs.h:87
Definition: cavs.h:88
Definition: cavs.h:132
static void intra_pred_lp(uint8_t *d, uint8_t *top, uint8_t *left, int stride)
Definition: cavs.c:296
Definition: cavs.h:99
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
Definition: cavs.h:105
static void mc_dir_part(AVSContext *h, AVFrame *pic, int chroma_height, int delta, int list, uint8_t *dest_y, uint8_t *dest_cb, uint8_t *dest_cr, int src_x_offset, int src_y_offset, qpel_mc_func *qpix_op, h264_chroma_mc_func chroma_op, cavs_vector *mv)
Definition: cavs.c:379
Definition: cavs.h:131
Definition: cavs.h:162
static void intra_pred_down_left(uint8_t *d, uint8_t *top, uint8_t *left, int stride)
Definition: cavs.c:304
qpel_mc_func avg_cavs_qpel_pixels_tab[2][16]
Definition: cavsdsp.h:30
void ff_init_scantable_permutation(uint8_t *idct_permutation, int idct_permutation_type)
Definition: dsputil.c:131
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
AVFrame * av_frame_alloc(void)
Allocate an AVFrame and set its fields to default values.
Definition: frame.c:95
planar YUV 4:2:0, 12bpp, (1 Cr & Cb sample per 2x2 Y samples)
Definition: pixfmt.h:68
void av_frame_free(AVFrame **frame)
Free the frame and any dynamically allocated objects in it, e.g.
Definition: frame.c:108
Definition: cavs.h:129
Definition: cavs.h:89
static void intra_pred_vert(uint8_t *d, uint8_t *top, uint8_t *left, int stride)
Definition: cavs.c:247
void(* emulated_edge_mc)(uint8_t *buf, const uint8_t *src, ptrdiff_t linesize, int block_w, int block_h, int src_x, int src_y, int w, int h)
Copy a rectangular area of samples to a temporary buffer and replicate the border samples...
Definition: videodsp.h:58
h264_chroma_mc_func put_h264_chroma_pixels_tab[3]
Definition: h264chroma.h:27
Definition: cavs.h:108
static void intra_pred_down_right(uint8_t *d, uint8_t *top, uint8_t *left, int stride)
Definition: cavs.c:312
void ff_init_scantable(uint8_t *permutation, ScanTable *st, const uint8_t *src_scantable)
Definition: dsputil.c:110
Definition: cavs.h:120
Definition: cavs.h:86
Definition: cavs.h:130
Definition: cavs.h:139
exp golomb vlc stuff
Definition: cavs.h:106
Definition: cavs.h:123
static void mc_part_std(AVSContext *h, int chroma_height, int delta, uint8_t *dest_y, uint8_t *dest_cb, uint8_t *dest_cr, int x_offset, int y_offset, qpel_mc_func *qpix_put, h264_chroma_mc_func chroma_put, qpel_mc_func *qpix_avg, h264_chroma_mc_func chroma_avg, cavs_vector *mv)
Definition: cavs.c:431
Generated on Tue Jul 8 2025 06:56:43 for FFmpeg by
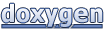