FFmpeg
|
swresample_internal.h
Go to the documentation of this file.
38 typedef void (mix_1_1_func_type)(void *out, const void *in, void *coeffp, integer index, integer len);
39 typedef void (mix_2_1_func_type)(void *out, const void *in1, const void *in2, void *coeffp, integer index1, integer index2, integer len);
74 enum AVSampleFormat int_sample_fmt; ///< internal sample format (AV_SAMPLE_FMT_FLTP or AV_SAMPLE_FMT_S16P)
87 int used_ch_count; ///< number of used input channels (mapped channel count if channel_map, otherwise in.ch_count)
92 int filter_size; /**< length of each FIR filter in the resampling filterbank relative to the cutoff frequency */
95 double cutoff; /**< resampling cutoff frequency (swr: 6dB point; soxr: 0dB point). 1.0 corresponds to half the output sample rate */
97 int kaiser_beta; /**< swr beta value for Kaiser window (only applicable if filter_type == AV_FILTER_TYPE_KAISER) */
99 int cheby; /**< soxr: if 1 then passband rolloff will be none (Chebyshev) & irrational ratio approximation precision will be higher */
102 float min_hard_compensation; ///< swr minimum below which no silence inject / sample drop will happen
104 float max_soft_compensation; ///< swr maximum soft compensation in seconds over soft_compensation_duration
109 int rematrix; ///< flag to indicate if rematrixing is needed (basically if input and output layouts mismatch)
122 int resample_in_constraint; ///< 1 if the input end was reach before the output end, 0 otherwise
130 struct AudioConvert *full_convert; ///< full conversion context (single conversion for input and output)
139 uint8_t matrix_ch[SWR_CH_MAX][SWR_CH_MAX+1]; ///< Lists of input channels per output channel that have non zero rematrixing coefficients
151 typedef struct ResampleContext * (* resample_init_func)(struct ResampleContext *c, int out_rate, int in_rate, int filter_size, int phase_shift, int linear,
152 double cutoff, enum AVSampleFormat format, enum SwrFilterType filter_type, int kaiser_beta, double precision, int cheby);
154 typedef int (* multiple_resample_func)(struct ResampleContext *c, AudioData *dst, int dst_size, AudioData *src, int src_size, int *consumed);
156 typedef int (* set_compensation_func)(struct ResampleContext *c, int sample_delta, int compensation_distance);
171 int swri_resample_int16(struct ResampleContext *c, int16_t *dst, const int16_t *src, int *consumed, int src_size, int dst_size, int update_ctx);
172 int swri_resample_int32(struct ResampleContext *c, int32_t *dst, const int32_t *src, int *consumed, int src_size, int dst_size, int update_ctx);
173 int swri_resample_float(struct ResampleContext *c, float *dst, const float *src, int *consumed, int src_size, int dst_size, int update_ctx);
174 int swri_resample_double(struct ResampleContext *c,double *dst, const double *src, int *consumed, int src_size, int dst_size, int update_ctx);
176 void swri_noise_shaping_int16 (SwrContext *s, AudioData *dsts, const AudioData *srcs, const AudioData *noises, int count);
177 void swri_noise_shaping_int32 (SwrContext *s, AudioData *dsts, const AudioData *srcs, const AudioData *noises, int count);
178 void swri_noise_shaping_float (SwrContext *s, AudioData *dsts, const AudioData *srcs, const AudioData *noises, int count);
179 void swri_noise_shaping_double(SwrContext *s, AudioData *dsts, const AudioData *srcs, const AudioData *noises, int count);
186 void swri_get_dither(SwrContext *s, void *dst, int len, unsigned seed, enum AVSampleFormat noise_fmt);
struct AudioData AudioData
struct AudioConvert * full_convert
full conversion context (single conversion for input and output)
Definition: swresample_internal.h:130
int64_t(* get_delay_func)(struct SwrContext *s, int64_t base)
Definition: swresample_internal.h:157
AudioData temp
temporary storage when writing into the input buffer isnt possible
Definition: swresample_internal.h:65
Audio buffer used for intermediate storage between conversion phases.
Definition: oss_audio.c:46
multiple_resample_func multiple_resample
Definition: swresample_internal.h:162
int swri_resample_float(struct ResampleContext *c, float *dst, const float *src, int *consumed, int src_size, int dst_size, int update_ctx)
float soft_compensation_duration
swr duration over which soft compensation is applied
Definition: swresample_internal.h:103
int rematrix_custom
flag to indicate that a custom matrix has been defined
Definition: swresample_internal.h:110
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip in
Definition: git-howto.txt:5
AudioData in_buffer
cached audio data (convert and resample purpose)
Definition: swresample_internal.h:117
int resample_in_constraint
1 if the input end was reach before the output end, 0 otherwise
Definition: swresample_internal.h:122
Definition: audio_convert.c:48
float async
swr simple 1 parameter async, similar to ffmpegs -async
Definition: swresample_internal.h:105
const int * channel_map
channel index (or -1 if muted channel) map
Definition: swresample_internal.h:86
Definition: af_resample.c:39
void( mix_2_1_func_type)(void *out, const void *in1, const void *in2, void *coeffp, integer index1, integer index2, integer len)
Definition: swresample_internal.h:39
struct Resampler const * resampler
resampler virtual function table
Definition: swresample_internal.h:132
Definition: swresample_internal.h:159
void(* resample_free_func)(struct ResampleContext **c)
Definition: swresample_internal.h:153
float max_soft_compensation
swr maximum soft compensation in seconds over soft_compensation_duration
Definition: swresample_internal.h:104
void swri_audio_convert_init_arm(struct AudioConvert *ac, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels)
Definition: libswresample/arm/audio_convert_init.c:48
int phase_shift
log2 of the number of entries in the resampling polyphase filterbank
Definition: swresample_internal.h:93
int swri_dither_init(SwrContext *s, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt)
Definition: libswresample/dither.c:75
int swri_resample_double(struct ResampleContext *c, double *dst, const double *src, int *consumed, int src_size, int dst_size, int update_ctx)
float min_hard_compensation
swr minimum below which no silence inject / sample drop will happen
Definition: swresample_internal.h:102
enum AVResampleFilterType filter_type
Definition: libavresample/resample.c:43
libswresample public header
AudioData postin
post-input audio data: used for rematrix/resample
Definition: swresample_internal.h:113
int output_sample_bits
the number of used output bits, needed to scale dither correctly
Definition: swresample_internal.h:66
Definition: swresample_internal.h:69
double cutoff
resampling cutoff frequency (swr: 6dB point; soxr: 0dB point).
Definition: swresample_internal.h:95
struct ResampleContext *(* resample_init_func)(struct ResampleContext *c, int out_rate, int in_rate, int filter_size, int phase_shift, int linear, double cutoff, enum AVSampleFormat format, enum SwrFilterType filter_type, int kaiser_beta, double precision, int cheby)
Definition: swresample_internal.h:151
Definition: libavresample/dither.c:53
void swri_audio_convert_init_x86(struct AudioConvert *ac, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels)
Definition: swresample_x86.c:33
void( mix_any_func_type)(uint8_t **out, const uint8_t **in1, void *coeffp, integer len)
Definition: swresample_internal.h:41
AudioData preout
pre-output audio data: used for rematrix/resample
Definition: swresample_internal.h:115
int(* multiple_resample_func)(struct ResampleContext *c, AudioData *dst, int dst_size, AudioData *src, int src_size, int *consumed)
Definition: swresample_internal.h:154
int(* resample_flush_func)(struct SwrContext *c)
Definition: swresample_internal.h:155
void swri_rematrix_init_x86(struct SwrContext *s)
audio channel layout utility functions
int linear_interp
if 1 then the resampling FIR filter will be linearly interpolated
Definition: swresample_internal.h:94
void swri_noise_shaping_int32(SwrContext *s, AudioData *dsts, const AudioData *srcs, const AudioData *noises, int count)
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample format(the sample packing is implied by the sample format) and sample rate.The lists are not just lists
int rematrix
flag to indicate if rematrixing is needed (basically if input and output layouts mismatch) ...
Definition: swresample_internal.h:109
set_compensation_func set_compensation
Definition: swresample_internal.h:164
void swri_noise_shaping_double(SwrContext *s, AudioData *dsts, const AudioData *srcs, const AudioData *noises, int count)
int filter_size
length of each FIR filter in the resampling filterbank relative to the cutoff frequency ...
Definition: swresample_internal.h:92
int swri_resample_int32(struct ResampleContext *c, int32_t *dst, const int32_t *src, int *consumed, int src_size, int dst_size, int update_ctx)
float min_compensation
swr minimum below which no compensation will happen
Definition: swresample_internal.h:101
void swri_noise_shaping_float(SwrContext *s, AudioData *dsts, const AudioData *srcs, const AudioData *noises, int count)
int swri_rematrix(SwrContext *s, AudioData *out, AudioData *in, int len, int mustcopy)
Definition: rematrix.c:393
int flushed
1 if data is to be flushed and no further input is expected
Definition: swresample_internal.h:123
void( mix_1_1_func_type)(void *out, const void *in, void *coeffp, integer index, integer len)
Definition: swresample_internal.h:38
void swri_get_dither(SwrContext *s, void *dst, int len, unsigned seed, enum AVSampleFormat noise_fmt)
Definition: libswresample/dither.c:26
int cheby
soxr: if 1 then passband rolloff will be none (Chebyshev) & irrational ratio approximation precision ...
Definition: swresample_internal.h:99
void swri_noise_shaping_int16(SwrContext *s, AudioData *dsts, const AudioData *srcs, const AudioData *noises, int count)
int kaiser_beta
swr beta value for Kaiser window (only applicable if filter_type == AV_FILTER_TYPE_KAISER) ...
Definition: swresample_internal.h:97
int(* set_compensation_func)(struct ResampleContext *c, int sample_delta, int compensation_distance)
Definition: swresample_internal.h:156
int compensation_distance
Definition: libavresample/resample.c:39
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> out
Definition: audio_convert.c:194
int swri_resample_int16(struct ResampleContext *c, int16_t *dst, const int16_t *src, int *consumed, int src_size, int dst_size, int update_ctx)
int used_ch_count
number of used input channels (mapped channel count if channel_map, otherwise in.ch_count) ...
Definition: swresample_internal.h:87
int resample_first
1 if resampling must come first, 0 if rematrixing
Definition: swresample_internal.h:108
Generated on Fri May 30 2025 06:54:02 for FFmpeg by
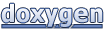