FFmpeg
|
libavresample/dither.c
Go to the documentation of this file.
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
int ff_audio_data_realloc(AudioData *a, int nb_samples)
Reallocate AudioData.
Definition: audio_data.c:153
struct DitherState DitherState
Audio buffer used for intermediate storage between conversion phases.
Definition: oss_audio.c:46
static int generate_dither_noise(DitherContext *c, DitherState *state, int min_samples)
Definition: libavresample/dither.c:141
memory handling functions
AudioData * ff_audio_data_alloc(int channels, int nb_samples, enum AVSampleFormat sample_fmt, const char *name)
Allocate AudioData.
Definition: audio_data.c:110
int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in)
Convert audio data from one sample format to another.
Definition: audio_convert.c:48
void(* quantize)(int16_t *dst, const float *src, float *dither, int len)
Definition: libavresample/dither.c:72
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
static void dither_int_to_float_rectangular_c(float *dst, int *src, int len)
Definition: libavresample/dither.c:104
void(* quantize)(int16_t *dst, const float *src, float *dither, int len)
Convert samples from flt to s16 with added dither noise.
Definition: dither.h:38
AudioConvert * ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map)
Allocate and initialize AudioConvert context for sample format conversion.
Definition: dither.h:29
static int convert_samples(DitherContext *c, int16_t **dst, float *const *src, int channels, int nb_samples)
Definition: libavresample/dither.c:210
static void quantize_c(int16_t *dst, const float *src, float *dither, int len)
Definition: libavresample/dither.c:123
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
Definition: libavresample/internal.h:43
Definition: libavresample/dither.c:53
void(* dither_int_to_float)(float *dst, int *src0, int len)
Convert dither noise from int to float with triangular distribution.
Definition: dither.h:54
DitherContext * ff_dither_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map)
Allocate and initialize a DitherContext.
Definition: libavresample/dither.c:344
int ff_convert_dither(DitherContext *c, AudioData *dst, AudioData *src)
Convert audio sample format with dithering.
Definition: libavresample/dither.c:241
Definition: libavresample/dither.c:42
enum AVSampleFormat av_get_packed_sample_fmt(enum AVSampleFormat sample_fmt)
Get the packed alternative form of the given sample format.
Definition: samplefmt.c:73
ChannelMapInfo ch_map_info
Definition: libavresample/internal.h:107
const char * av_get_sample_fmt_name(enum AVSampleFormat sample_fmt)
Return the name of sample_fmt, or NULL if sample_fmt is not recognized.
Definition: samplefmt.c:47
int av_get_bytes_per_sample(enum AVSampleFormat sample_fmt)
Return number of bytes per sample.
Definition: samplefmt.c:104
static void dither_highpass_filter(float *src, int len)
Definition: libavresample/dither.c:132
static unsigned int av_lfg_get(AVLFG *c)
Get the next random unsigned 32-bit number using an ALFG.
Definition: lfg.h:38
static void quantize_triangular_ns(DitherContext *c, DitherState *state, int16_t *dst, const float *src, int nb_samples)
Definition: libavresample/dither.c:171
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
static void dither_int_to_float_triangular_c(float *dst, int *src0, int len)
Definition: libavresample/dither.c:111
static void dither_init(DitherDSPContext *ddsp, enum AVResampleDitherMethod method)
Definition: libavresample/dither.c:328
int ff_audio_data_copy(AudioData *dst, AudioData *src, ChannelMapInfo *map)
Copy data from one AudioData to another.
Definition: audio_data.c:216
common internal and external API header
void ff_dither_init_x86(DitherDSPContext *ddsp, enum AVResampleDitherMethod method)
Definition: dither_init.c:34
Generated on Fri May 30 2025 06:53:54 for FFmpeg by
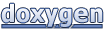