FFmpeg
|
opencl.c
Go to the documentation of this file.
81 { "platform_idx", "set platform index value", OFFSET(platform_idx), AV_OPT_TYPE_INT, {.i64=-1}, -1, INT_MAX},
82 { "device_idx", "set device index value", OFFSET(device_idx), AV_OPT_TYPE_INT, {.i64=-1}, -1, INT_MAX},
83 { "build_options", "build options of opencl", OFFSET(build_options), AV_OPT_TYPE_STRING, {.str="-I."}, CHAR_MIN, CHAR_MAX},
97 static const cl_device_type device_type[] = {CL_DEVICE_TYPE_GPU, CL_DEVICE_TYPE_CPU, CL_DEVICE_TYPE_DEFAULT};
217 device_list->platform_node = av_mallocz(device_list->platform_num * sizeof(AVOpenCLPlatformNode *));
243 device_list->platform_node[i]->device_node = av_mallocz(total_devices_num * sizeof(AVOpenCLDeviceNode *));
265 device_list->platform_node[i]->device_node[device_num] = av_mallocz(sizeof(AVOpenCLDeviceNode));
371 "Could not register kernel code, maximum number of registered kernel code %d already reached\n",
419 av_log(&opencl_ctx, AV_LOG_ERROR, "Could not create OpenCL kernel: %s\n", opencl_errstr(status));
485 opencl_ctx->platform_id = opencl_ctx->device_list.platform_node[opencl_ctx->platform_idx]->platform_id;
502 if (opencl_ctx->device_list.platform_node[opencl_ctx->platform_idx]->device_num < opencl_ctx->device_idx + 1) {
511 device_node = opencl_ctx->device_list.platform_node[opencl_ctx->platform_idx]->device_node[opencl_ctx->device_idx];
572 opencl_ctx->programs[opencl_ctx->program_count] = clCreateProgramWithSource(opencl_ctx->context,
586 status = clBuildProgram(opencl_ctx->programs[opencl_ctx->program_count], 1, &(opencl_ctx->device_id),
677 av_log(&opencl_ctx, AV_LOG_ERROR, "Could not create OpenCL buffer: %s\n", opencl_errstr(status));
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
static int compile_kernel_file(OpenclContext *opencl_ctx)
Definition: opencl.c:544
char platform_name[AV_OPENCL_MAX_PLATFORM_NAME_SIZE]
Definition: opencl.h:54
Definition: opencl.h:52
char kernel_name[AV_OPENCL_MAX_KERNEL_NAME_SIZE]
Definition: opencl.h:67
Definition: opt.h:222
void av_opt_set_defaults(void *s)
Set the values of all AVOption fields to their default values.
Definition: opt.c:942
int av_opencl_buffer_read(uint8_t *dst_buf, cl_mem src_cl_buf, size_t buf_size)
Read data from OpenCL buffer to memory buffer.
Definition: opencl.c:719
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
static int init_opencl_env(OpenclContext *opencl_ctx, AVOpenCLExternalEnv *ext_opencl_env)
Definition: opencl.c:451
const char * class_name
The name of the class; usually it is the same name as the context structure type to which the AVClass...
Definition: log.h:55
AVOptions.
Definition: opt.h:226
int av_opencl_get_device_list(AVOpenCLDeviceList **device_list)
Get OpenCL device list.
Definition: opencl.c:296
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
simple assert() macros that are a bit more flexible than ISO C assert().
size_t av_strlcpy(char *dst, const char *src, size_t size)
Copy the string src to dst, but no more than size - 1 bytes, and null-terminate dst.
Definition: avstring.c:82
static void free_device_list(AVOpenCLDeviceList *device_list)
Definition: opencl.c:175
int av_opencl_set_option(const char *key, const char *val)
Set option in the global OpenCL context.
Definition: opencl.c:320
int av_opencl_buffer_create(cl_mem *cl_buf, size_t cl_buf_size, int flags, void *host_ptr)
Create OpenCL buffer.
Definition: opencl.c:672
int av_opencl_buffer_write_image(cl_mem dst_cl_buf, size_t cl_buffer_size, int dst_cl_offset, uint8_t **src_data, int *plane_size, int plane_num)
Write image data from memory to OpenCL buffer.
Definition: opencl.c:742
Definition: opencl.c:51
void av_opencl_release_kernel(AVOpenCLKernelEnv *env)
Release kernel object.
Definition: opencl.c:432
int av_opencl_register_kernel_code(const char *kernel_code)
Register kernel code.
Definition: opencl.c:365
void av_opencl_free_external_env(AVOpenCLExternalEnv **ext_opencl_env)
Free OpenCL external environment.
Definition: opencl.c:360
Definition: opencl.c:46
int av_opencl_create_kernel(AVOpenCLKernelEnv *env, const char *kernel_name)
Create kernel object in the specified kernel environment.
Definition: opencl.c:390
Definition: opencl.h:64
void av_opencl_free_option(void)
Free option values of the global OpenCL context.
Definition: opencl.c:342
AVOpenCLExternalEnv * av_opencl_alloc_external_env(void)
Allocate OpenCL external environment.
Definition: opencl.c:350
Definition: opencl.h:46
Definition: opencl.c:99
int is_user_created
if set to 1, the OpenCL environment was created by the user and passed as AVOpenCLExternalEnv when in...
Definition: opencl.c:61
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
char device_name[AV_OPENCL_MAX_DEVICE_NAME_SIZE]
Definition: opencl.h:48
int av_opencl_buffer_write(cl_mem dst_cl_buf, uint8_t *src_buf, size_t buf_size)
Write OpenCL buffer with data from src_buf.
Definition: opencl.c:696
void av_opencl_free_device_list(AVOpenCLDeviceList **device_list)
Free OpenCL device list.
Definition: opencl.c:314
int av_opt_get(void *obj, const char *name, int search_flags, uint8_t **out_val)
Definition: opt.c:566
int av_opencl_init(AVOpenCLExternalEnv *ext_opencl_env)
Initialize the run time OpenCL environment and compile the kernel code registered with av_opencl_regi...
Definition: opencl.c:600
Definition: opencl.h:59
OpenCL wrapper.
int av_opencl_get_option(const char *key, uint8_t **out_val)
Get option value from the global OpenCL context.
Definition: opencl.c:333
Definition: opencl.h:70
int av_opt_set(void *obj, const char *name, const char *val, int search_flags)
Definition: opt.c:252
int av_opencl_buffer_read_image(uint8_t **dst_data, int *plane_size, int plane_num, cl_mem src_cl_buf, size_t cl_buffer_size)
Read image data from OpenCL buffer.
Definition: opencl.c:783
Generated on Thu May 29 2025 06:52:51 for FFmpeg by
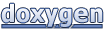