FFmpeg
|
swscale_internal.h
Go to the documentation of this file.
284 int chrSrcHSubSample; ///< Binary logarithm of horizontal subsampling factor between luma/alpha and chroma planes in source image.
285 int chrSrcVSubSample; ///< Binary logarithm of vertical subsampling factor between luma/alpha and chroma planes in source image.
286 int chrDstHSubSample; ///< Binary logarithm of horizontal subsampling factor between luma/alpha and chroma planes in destination image.
287 int chrDstVSubSample; ///< Binary logarithm of vertical subsampling factor between luma/alpha and chroma planes in destination image.
288 int vChrDrop; ///< Binary logarithm of extra vertical subsampling factor in source image chroma planes specified by user.
289 int sliceDir; ///< Direction that slices are fed to the scaler (1 = top-to-bottom, -1 = bottom-to-top).
305 int16_t **lumPixBuf; ///< Ring buffer for scaled horizontal luma plane lines to be fed to the vertical scaler.
306 int16_t **chrUPixBuf; ///< Ring buffer for scaled horizontal chroma plane lines to be fed to the vertical scaler.
307 int16_t **chrVPixBuf; ///< Ring buffer for scaled horizontal chroma plane lines to be fed to the vertical scaler.
308 int16_t **alpPixBuf; ///< Ring buffer for scaled horizontal alpha plane lines to be fed to the vertical scaler.
313 int lumBufIndex; ///< Index in ring buffer of the last scaled horizontal luma/alpha line from source.
314 int chrBufIndex; ///< Index in ring buffer of the last scaled horizontal chroma line from source.
337 int32_t *hLumFilterPos; ///< Array of horizontal filter starting positions for each dst[i] for luma/alpha planes.
338 int32_t *hChrFilterPos; ///< Array of horizontal filter starting positions for each dst[i] for chroma planes.
339 int32_t *vLumFilterPos; ///< Array of vertical filter starting positions for each dst[i] for luma/alpha planes.
340 int32_t *vChrFilterPos; ///< Array of vertical filter starting positions for each dst[i] for chroma planes.
347 int lumMmxextFilterCodeSize; ///< Runtime-generated MMXEXT horizontal fast bilinear scaler code size for luma/alpha planes.
348 int chrMmxextFilterCodeSize; ///< Runtime-generated MMXEXT horizontal fast bilinear scaler code size for chroma planes.
349 uint8_t *lumMmxextFilterCode; ///< Runtime-generated MMXEXT horizontal fast bilinear scaler code for luma/alpha planes.
350 uint8_t *chrMmxextFilterCode; ///< Runtime-generated MMXEXT horizontal fast bilinear scaler code for chroma planes.
355 int flags; ///< Flags passed by the user to select scaler algorithm, optimizations, subsampling, etc...
361 DECLARE_ALIGNED(16, int32_t, input_rgb2yuv_table)[16+40*4]; // This table can contain both C and SIMD formatted values, teh C vales are always at the XY_IDX points
void ff_sws_init_output_funcs(SwsContext *c, yuv2planar1_fn *yuv2plane1, yuv2planarX_fn *yuv2planeX, yuv2interleavedX_fn *yuv2nv12cX, yuv2packed1_fn *yuv2packed1, yuv2packed2_fn *yuv2packed2, yuv2packedX_fn *yuv2packedX, yuv2anyX_fn *yuv2anyX)
Definition: output.c:1565
int16_t ** alpPixBuf
Ring buffer for scaled horizontal alpha plane lines to be fed to the vertical scaler.
Definition: swscale_internal.h:308
void(* hcScale)(struct SwsContext *c, int16_t *dst, int dstW, const uint8_t *src, const int16_t *filter, const int32_t *filterPos, int filterSize)
Definition: swscale_internal.h:568
const uint64_t ff_dither8[2]
int chrBufIndex
Index in ring buffer of the last scaled horizontal chroma line from source.
Definition: swscale_internal.h:314
const AVPixFmtDescriptor * av_pix_fmt_desc_get(enum AVPixelFormat pix_fmt)
Definition: pixdesc.c:1778
static av_always_inline int isPlanarRGB(enum AVPixelFormat pix_fmt)
Definition: swscale_internal.h:780
void(* chrConvertRange)(int16_t *dst1, int16_t *dst2, int width)
Color range conversion function for chroma planes if needed.
Definition: swscale_internal.h:576
uint8_t * chrMmxextFilterCode
Runtime-generated MMXEXT horizontal fast bilinear scaler code for chroma planes.
Definition: swscale_internal.h:350
const char * sws_format_name(enum AVPixelFormat format)
Definition: libswscale/utils.c:216
uint8_t * lumMmxextFilterCode
Runtime-generated MMXEXT horizontal fast bilinear scaler code for luma/alpha planes.
Definition: swscale_internal.h:349
void updateMMXDitherTables(SwsContext *c, int dstY, int lumBufIndex, int chrBufIndex, int lastInLumBuf, int lastInChrBuf)
int vChrDrop
Binary logarithm of extra vertical subsampling factor in source image chroma planes specified by user...
Definition: swscale_internal.h:288
int ff_yuv2rgb_c_init_tables(SwsContext *c, const int inv_table[4], int fullRange, int brightness, int contrast, int saturation)
Definition: yuv2rgb.c:706
void(* chrToYV12)(uint8_t *dstU, uint8_t *dstV, const uint8_t *src1, const uint8_t *src2, const uint8_t *src3, int width, uint32_t *pal)
Unscaled conversion of chroma planes to YV12 for horizontal scaler.
Definition: swscale_internal.h:492
uint8_t * table_bU[256+2 *YUVRGB_TABLE_HEADROOM]
Definition: swscale_internal.h:360
int dstFormatBpp
Number of bits per pixel of the destination pixel format.
Definition: swscale_internal.h:281
void(* alpToYV12)(uint8_t *dst, const uint8_t *src, const uint8_t *src2, const uint8_t *src3, int width, uint32_t *pal)
Unscaled conversion of alpha plane to YV12 for horizontal scaler.
Definition: swscale_internal.h:489
static av_always_inline int is16BPS(enum AVPixelFormat pix_fmt)
Definition: swscale_internal.h:606
external API header
void(* hyScale)(struct SwsContext *c, int16_t *dst, int dstW, const uint8_t *src, const int16_t *filter, const int32_t *filterPos, int filterSize)
Scale one horizontal line of input data using a filter over the input lines, to produce one (differen...
Definition: swscale_internal.h:565
void(* hyscale_fast)(struct SwsContext *c, int16_t *dst, int dstWidth, const uint8_t *src, int srcW, int xInc)
Scale one horizontal line of input data using a bilinear filter to produce one line of output data...
Definition: swscale_internal.h:525
SwsFunc swScale
Note that src, dst, srcStride, dstStride will be copied in the sws_scale() wrapper so they can be fre...
Definition: swscale_internal.h:269
int chrDstVSubSample
Binary logarithm of vertical subsampling factor between luma/alpha and chroma planes in destination i...
Definition: swscale_internal.h:287
AVComponentDescriptor comp[4]
Parameters that describe how pixels are packed.
Definition: pixdesc.h:86
int16_t ** lumPixBuf
Ring buffer for scaled horizontal luma plane lines to be fed to the vertical scaler.
Definition: swscale_internal.h:305
the mask is usually to keep the same permissions Filters should remove permissions on reference they give to output whenever necessary It can be automatically done by setting the rej_perms field on the output pad Here are a few guidelines corresponding to common then the filter should push the output frames on the output link immediately As an exception to the previous rule if the input frame is enough to produce several output frames then the filter needs output only at least one per link The additional frames can be left buffered in the filter
Definition: filter_design.txt:201
int lastInLumBuf
Last scaled horizontal luma/alpha line from source in the ring buffer.
Definition: swscale_internal.h:311
void(* yuv2planar1_fn)(const int16_t *src, uint8_t *dest, int dstW, const uint8_t *dither, int offset)
Write one line of horizontally scaled data to planar output without any additional vertical scaling (...
Definition: swscale_internal.h:80
uint8_t * table_gU[256+2 *YUVRGB_TABLE_HEADROOM]
Definition: swscale_internal.h:358
int chrSrcHSubSample
Binary logarithm of horizontal subsampling factor between luma/alpha and chroma planes in source imag...
Definition: swscale_internal.h:284
static av_always_inline int isYUV(enum AVPixelFormat pix_fmt)
Definition: swscale_internal.h:629
int32_t * vChrFilterPos
Array of vertical filter starting positions for each dst[i] for chroma planes.
Definition: swscale_internal.h:340
struct SwsContext SwsContext
void ff_yuv2rgb_init_tables_altivec(SwsContext *c, const int inv_table[4], int brightness, int contrast, int saturation)
Definition: yuv2rgb_altivec.c:595
const uint64_t ff_dither4[2]
int16_t ** chrVPixBuf
Ring buffer for scaled horizontal chroma plane lines to be fed to the vertical scaler.
Definition: swscale_internal.h:307
int32_t * hChrFilterPos
Array of horizontal filter starting positions for each dst[i] for chroma planes.
Definition: swscale_internal.h:338
simple assert() macros that are a bit more flexible than ISO C assert().
#define PIX_FMT_PAL
Pixel format has a palette in data[1], values are indexes in this palette.
Definition: pixdesc.h:90
int vChrBufSize
Number of vertical chroma lines allocated in the ring buffer.
Definition: swscale_internal.h:310
void ff_bfin_get_unscaled_swscale(SwsContext *c)
Definition: swscale_bfin.c:74
void(* hcscale_fast)(struct SwsContext *c, int16_t *dst1, int16_t *dst2, int dstWidth, const uint8_t *src1, const uint8_t *src2, int srcW, int xInc)
Definition: swscale_internal.h:528
int32_t * hLumFilterPos
Array of horizontal filter starting positions for each dst[i] for luma/alpha planes.
Definition: swscale_internal.h:337
int dstRange
0 = MPG YUV range, 1 = JPG YUV range (destination image).
Definition: swscale_internal.h:380
static av_always_inline int is9_OR_10BPS(enum AVPixelFormat pix_fmt)
Definition: swscale_internal.h:613
int vLumBufSize
Number of vertical luma/alpha lines allocated in the ring buffer.
Definition: swscale_internal.h:309
void(* readChrPlanar)(uint8_t *dstU, uint8_t *dstV, const uint8_t *src[4], int width, int32_t *rgb2yuv)
Definition: swscale_internal.h:502
int16_t ** chrUPixBuf
Ring buffer for scaled horizontal chroma plane lines to be fed to the vertical scaler.
Definition: swscale_internal.h:306
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample format(the sample packing is implied by the sample format) and sample rate.The lists are not just lists
void(* lumConvertRange)(int16_t *dst, int width)
Color range conversion function for luma plane if needed.
Definition: swscale_internal.h:574
uint8_t * table_rV[256+2 *YUVRGB_TABLE_HEADROOM]
Definition: swscale_internal.h:357
void ff_swscale_get_unscaled_altivec(SwsContext *c)
Definition: yuv2yuv_altivec.c:182
int sliceDir
Direction that slices are fed to the scaler (1 = top-to-bottom, -1 = bottom-to-top).
Definition: swscale_internal.h:289
int(* SwsFunc)(struct SwsContext *context, const uint8_t *src[], int srcStride[], int srcSliceY, int srcSliceH, uint8_t *dst[], int dstStride[])
Definition: swscale_internal.h:64
int32_t * vLumFilterPos
Array of vertical filter starting positions for each dst[i] for luma/alpha planes.
Definition: swscale_internal.h:339
SwsFunc ff_getSwsFunc(SwsContext *c)
Return function pointer to fastest main scaler path function depending on architecture and available ...
Definition: swscale.c:755
#define PIX_FMT_PLANAR
At least one pixel component is not in the first data plane.
Definition: pixdesc.h:93
void(* yuv2packedX_fn)(struct SwsContext *c, const int16_t *lumFilter, const int16_t **lumSrc, int lumFilterSize, const int16_t *chrFilter, const int16_t **chrUSrc, const int16_t **chrVSrc, int chrFilterSize, const int16_t **alpSrc, uint8_t *dest, int dstW, int y)
Write one line of horizontally scaled Y/U/V/A to packed-pixel YUV/RGB output by doing multi-point ver...
Definition: swscale_internal.h:216
static av_always_inline int isPlanar(enum AVPixelFormat pix_fmt)
Definition: swscale_internal.h:766
Descriptor that unambiguously describes how the bits of a pixel are stored in the up to 4 data planes...
Definition: pixdesc.h:55
void(* yuv2packed1_fn)(struct SwsContext *c, const int16_t *lumSrc, const int16_t *chrUSrc[2], const int16_t *chrVSrc[2], const int16_t *alpSrc, uint8_t *dest, int dstW, int uvalpha, int y)
Write one line of horizontally scaled Y/U/V/A to packed-pixel YUV/RGB output without any additional v...
Definition: swscale_internal.h:151
static av_always_inline int isRGB(enum AVPixelFormat pix_fmt)
Definition: swscale_internal.h:643
SwsFunc ff_yuv2rgb_init_altivec(SwsContext *c)
Definition: yuv2rgb_altivec.c:535
int lumMmxextFilterCodeSize
Runtime-generated MMXEXT horizontal fast bilinear scaler code size for luma/alpha planes...
Definition: swscale_internal.h:347
void(* yuv2planarX_fn)(const int16_t *filter, int filterSize, const int16_t **src, uint8_t *dest, int dstW, const uint8_t *dither, int offset)
Write one line of horizontally scaled data to planar output with multi-point vertical scaling between...
Definition: swscale_internal.h:96
SwsFunc ff_yuv2rgb_get_func_ptr_bfin(SwsContext *c)
Definition: yuv2rgb_bfin.c:171
int chrMmxextFilterCodeSize
Runtime-generated MMXEXT horizontal fast bilinear scaler code size for chroma planes.
Definition: swscale_internal.h:348
static av_always_inline int isPlanarYUV(enum AVPixelFormat pix_fmt)
Definition: swscale_internal.h:636
static av_always_inline int isALPHA(enum AVPixelFormat pix_fmt)
Definition: swscale_internal.h:740
int16_t * vChrFilter
Array of vertical filter coefficients for chroma planes.
Definition: swscale_internal.h:336
int16_t * hLumFilter
Array of horizontal filter coefficients for luma/alpha planes.
Definition: swscale_internal.h:333
static void fillPlane16(uint8_t *plane, int stride, int width, int height, int y, int alpha, int bits, const int big_endian)
Definition: swscale_internal.h:829
#define PIX_FMT_RGB
The pixel format contains RGB-like data (as opposed to YUV/grayscale)
Definition: pixdesc.h:94
int lumBufIndex
Index in ring buffer of the last scaled horizontal luma/alpha line from source.
Definition: swscale_internal.h:313
const uint8_t dithers[8][8][8]
int lastInChrBuf
Last scaled horizontal chroma line from source in the ring buffer.
Definition: swscale_internal.h:312
double param[2]
Input parameters for scaling algorithms that need them.
Definition: swscale_internal.h:290
common internal and external API header
void(* yuv2packed2_fn)(struct SwsContext *c, const int16_t *lumSrc[2], const int16_t *chrUSrc[2], const int16_t *chrVSrc[2], const int16_t *alpSrc[2], uint8_t *dest, int dstW, int yalpha, int uvalpha, int y)
Write one line of horizontally scaled Y/U/V/A to packed-pixel YUV/RGB output by doing bilinear scalin...
Definition: swscale_internal.h:184
void(* readLumPlanar)(uint8_t *dst, const uint8_t *src[4], int width, int32_t *rgb2yuv)
Functions to read planar input, such as planar RGB, and convert internally to Y/UV.
Definition: swscale_internal.h:501
pixel format definitions
void(* yuv2interleavedX_fn)(struct SwsContext *c, const int16_t *chrFilter, int chrFilterSize, const int16_t **chrUSrc, const int16_t **chrVSrc, uint8_t *dest, int dstW)
Write one line of horizontally scaled chroma to interleaved output with multi-point vertical scaling ...
Definition: swscale_internal.h:115
int srcFormatBpp
Number of bits per pixel of the source pixel format.
Definition: swscale_internal.h:282
void(* lumToYV12)(uint8_t *dst, const uint8_t *src, const uint8_t *src2, const uint8_t *src3, int width, uint32_t *pal)
Unscaled conversion of luma plane to YV12 for horizontal scaler.
Definition: swscale_internal.h:486
void(* yuv2anyX_fn)(struct SwsContext *c, const int16_t *lumFilter, const int16_t **lumSrc, int lumFilterSize, const int16_t *chrFilter, const int16_t **chrUSrc, const int16_t **chrVSrc, int chrFilterSize, const int16_t **alpSrc, uint8_t **dest, int dstW, int y)
Write one line of horizontally scaled Y/U/V/A to YUV/RGB output by doing multi-point vertical scaling...
Definition: swscale_internal.h:250
static av_always_inline int isPackedRGB(enum AVPixelFormat pix_fmt)
Definition: swscale_internal.h:773
int16_t * vLumFilter
Array of vertical filter coefficients for luma/alpha planes.
Definition: swscale_internal.h:335
void ff_sws_init_swScale_altivec(SwsContext *c)
Definition: swscale_altivec.c:288
DECLARE_ALIGNED(16, int32_t, input_rgb2yuv_table)[16+40 *4]
static av_always_inline int usePal(enum AVPixelFormat pix_fmt)
Definition: swscale_internal.h:788
int16_t * hChrFilter
Array of horizontal filter coefficients for chroma planes.
Definition: swscale_internal.h:334
int chrDstHSubSample
Binary logarithm of horizontal subsampling factor between luma/alpha and chroma planes in destination...
Definition: swscale_internal.h:286
Definition: swscale_internal.h:259
void ff_get_unscaled_swscale(SwsContext *c)
Set c->swScale to an unscaled converter if one exists for the specific source and destination formats...
Definition: swscale_unscaled.c:997
int chrSrcVSubSample
Binary logarithm of vertical subsampling factor between luma/alpha and chroma planes in source image...
Definition: swscale_internal.h:285
int flags
Flags passed by the user to select scaler algorithm, optimizations, subsampling, etc...
Definition: swscale_internal.h:355
#define FILL(wfunc)
Generated on Thu Jun 19 2025 06:53:14 for FFmpeg by
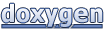