FFmpeg
|
multiple format streaming server based on the FFmpeg libraries More...
#include "config.h"
#include <string.h>
#include <stdlib.h>
#include <stdio.h>
#include "libavformat/avformat.h"
#include "libavformat/ffm.h"
#include "libavformat/network.h"
#include "libavformat/os_support.h"
#include "libavformat/rtpdec.h"
#include "libavformat/rtsp.h"
#include "libavformat/avio_internal.h"
#include "libavformat/internal.h"
#include "libavformat/url.h"
#include "libavutil/avassert.h"
#include "libavutil/avstring.h"
#include "libavutil/lfg.h"
#include "libavutil/dict.h"
#include "libavutil/intreadwrite.h"
#include "libavutil/mathematics.h"
#include "libavutil/random_seed.h"
#include "libavutil/parseutils.h"
#include "libavutil/opt.h"
#include "libavutil/time.h"
#include <stdarg.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <poll.h>
#include <errno.h>
#include <sys/wait.h>
#include <signal.h>
#include <dlfcn.h>
#include "cmdutils.h"
#include "cmdutils_common_opts.h"

Go to the source code of this file.
Data Structures | |
struct | RTSPActionServerSetup |
struct | DataRateData |
struct | HTTPContext |
struct | IPAddressACL |
struct | FFStream |
struct | FeedData |
Macros | |
#define | closesocket close |
#define | MAX_STREAMS 20 |
#define | IOBUFFER_INIT_SIZE 8192 |
#define | HTTP_REQUEST_TIMEOUT (15 * 1000) |
#define | RTSP_REQUEST_TIMEOUT (3600 * 24 * 1000) |
#define | SYNC_TIMEOUT (10 * 1000) |
#define | CHECK_CODEC(x) (ccf->x != ccs->x) |
#define | ERROR(...) report_config_error(filename, line_num, &errors, __VA_ARGS__) |
Typedefs | |
typedef struct RTSPActionServerSetup | RTSPActionServerSetup |
typedef struct HTTPContext | HTTPContext |
typedef struct IPAddressACL | IPAddressACL |
typedef struct FFStream | FFStream |
typedef struct FeedData | FeedData |
Functions | |
static void | new_connection (int server_fd, int is_rtsp) |
static void | close_connection (HTTPContext *c) |
static int | handle_connection (HTTPContext *c) |
static int | http_parse_request (HTTPContext *c) |
static int | http_send_data (HTTPContext *c) |
static void | compute_status (HTTPContext *c) |
static int | open_input_stream (HTTPContext *c, const char *info) |
static int | http_start_receive_data (HTTPContext *c) |
static int | http_receive_data (HTTPContext *c) |
static int | rtsp_parse_request (HTTPContext *c) |
static void | rtsp_cmd_describe (HTTPContext *c, const char *url) |
static void | rtsp_cmd_options (HTTPContext *c, const char *url) |
static void | rtsp_cmd_setup (HTTPContext *c, const char *url, RTSPMessageHeader *h) |
static void | rtsp_cmd_play (HTTPContext *c, const char *url, RTSPMessageHeader *h) |
static void | rtsp_cmd_pause (HTTPContext *c, const char *url, RTSPMessageHeader *h) |
static void | rtsp_cmd_teardown (HTTPContext *c, const char *url, RTSPMessageHeader *h) |
static int | prepare_sdp_description (FFStream *stream, uint8_t **pbuffer, struct in_addr my_ip) |
static HTTPContext * | rtp_new_connection (struct sockaddr_in *from_addr, FFStream *stream, const char *session_id, enum RTSPLowerTransport rtp_protocol) |
static int | rtp_new_av_stream (HTTPContext *c, int stream_index, struct sockaddr_in *dest_addr, HTTPContext *rtsp_c) |
static int64_t | ffm_read_write_index (int fd) |
static int | ffm_write_write_index (int fd, int64_t pos) |
static void | ffm_set_write_index (AVFormatContext *s, int64_t pos, int64_t file_size) |
static int | resolve_host (struct in_addr *sin_addr, const char *hostname) |
static char * | ctime1 (char *buf2, int buf_size) |
static void | http_vlog (const char *fmt, va_list vargs) |
static void | http_log (const char *fmt,...) |
static void | http_av_log (void *ptr, int level, const char *fmt, va_list vargs) |
static void | log_connection (HTTPContext *c) |
static void | update_datarate (DataRateData *drd, int64_t count) |
static int | compute_datarate (DataRateData *drd, int64_t count) |
static void | start_children (FFStream *feed) |
static int | socket_open_listen (struct sockaddr_in *my_addr) |
static void | start_multicast (void) |
static int | http_server (void) |
static void | start_wait_request (HTTPContext *c, int is_rtsp) |
static void | http_send_too_busy_reply (int fd) |
static int | extract_rates (char *rates, int ratelen, const char *request) |
static int | find_stream_in_feed (FFStream *feed, AVCodecContext *codec, int bit_rate) |
static int | modify_current_stream (HTTPContext *c, char *rates) |
static void | skip_spaces (const char **pp) |
static void | get_word (char *buf, int buf_size, const char **pp) |
static void | get_arg (char *buf, int buf_size, const char **pp) |
static void | parse_acl_row (FFStream *stream, FFStream *feed, IPAddressACL *ext_acl, const char *p, const char *filename, int line_num) |
static IPAddressACL * | parse_dynamic_acl (FFStream *stream, HTTPContext *c) |
static void | free_acl_list (IPAddressACL *in_acl) |
static int | validate_acl_list (IPAddressACL *in_acl, HTTPContext *c) |
static int | validate_acl (FFStream *stream, HTTPContext *c) |
static void | compute_real_filename (char *filename, int max_size) |
static void | fmt_bytecount (AVIOContext *pb, int64_t count) |
static int64_t | get_server_clock (HTTPContext *c) |
static int64_t | get_packet_send_clock (HTTPContext *c) |
static int | http_prepare_data (HTTPContext *c) |
static void | rtsp_reply_header (HTTPContext *c, enum RTSPStatusCode error_number) |
static void | rtsp_reply_error (HTTPContext *c, enum RTSPStatusCode error_number) |
static HTTPContext * | find_rtp_session (const char *session_id) |
static RTSPTransportField * | find_transport (RTSPMessageHeader *h, enum RTSPLowerTransport lower_transport) |
static HTTPContext * | find_rtp_session_with_url (const char *url, const char *session_id) |
static AVStream * | add_av_stream1 (FFStream *stream, AVCodecContext *codec, int copy) |
static int | add_av_stream (FFStream *feed, AVStream *st) |
static void | remove_stream (FFStream *stream) |
static void | extract_mpeg4_header (AVFormatContext *infile) |
static void | build_file_streams (void) |
static void | build_feed_streams (void) |
static void | compute_bandwidth (void) |
static void | add_codec (FFStream *stream, AVCodecContext *av) |
static enum AVCodecID | opt_audio_codec (const char *arg) |
static enum AVCodecID | opt_video_codec (const char *arg) |
static void | load_module (const char *filename) |
static int | ffserver_opt_default (const char *opt, const char *arg, AVCodecContext *avctx, int type) |
static int | ffserver_opt_preset (const char *arg, AVCodecContext *avctx, int type, enum AVCodecID *audio_id, enum AVCodecID *video_id) |
static AVOutputFormat * | ffserver_guess_format (const char *short_name, const char *filename, const char *mime_type) |
static void | report_config_error (const char *filename, int line_num, int *errors, const char *fmt,...) |
static int | parse_ffconfig (const char *filename) |
static void | handle_child_exit (int sig) |
static void | opt_debug (void) |
void | show_help_default (const char *opt, const char *arg) |
Per-fftool specific help handler. More... | |
int | main (int argc, char **argv) |
Variables | |
const char | program_name [] = "ffserver" |
program name, defined by the program for show_version(). More... | |
const int | program_birth_year = 2000 |
program birth year, defined by the program for show_banner() More... | |
static const OptionDef | options [] |
static const char * | http_state [] |
static struct sockaddr_in | my_http_addr |
static struct sockaddr_in | my_rtsp_addr |
static char | logfilename [1024] |
static HTTPContext * | first_http_ctx |
static FFStream * | first_feed |
static FFStream * | first_stream |
static const char * | my_program_name |
static const char * | config_filename |
static int | ffserver_debug |
static int | no_launch |
static int | need_to_start_children |
static unsigned int | nb_max_http_connections = 2000 |
static unsigned int | nb_max_connections = 5 |
static unsigned int | nb_connections |
static uint64_t | max_bandwidth = 1000 |
static uint64_t | current_bandwidth |
static int64_t | cur_time |
static AVLFG | random_state |
static FILE * | logfile = NULL |
Detailed Description
multiple format streaming server based on the FFmpeg libraries
Definition in file ffserver.c.
Macro Definition Documentation
Referenced by build_feed_streams().
#define closesocket close |
Definition at line 28 of file ffserver.c.
Referenced by close_connection(), new_connection(), sctp_close(), sctp_open(), socket_open_listen(), tcp_close(), tcp_open(), udp_close(), udp_open(), and udp_socket_create().
#define ERROR | ( | ... | ) | report_config_error(filename, line_num, &errors, __VA_ARGS__) |
Referenced by main(), and parse_ffconfig().
#define HTTP_REQUEST_TIMEOUT (15 * 1000) |
Definition at line 113 of file ffserver.c.
Referenced by start_wait_request().
#define IOBUFFER_INIT_SIZE 8192 |
Definition at line 110 of file ffserver.c.
Referenced by new_connection(), and rtp_new_connection().
#define MAX_STREAMS 20 |
Definition at line 108 of file ffserver.c.
#define RTSP_REQUEST_TIMEOUT (3600 * 24 * 1000) |
Definition at line 114 of file ffserver.c.
Referenced by start_wait_request().
#define SYNC_TIMEOUT (10 * 1000) |
Definition at line 116 of file ffserver.c.
Typedef Documentation
typedef struct HTTPContext HTTPContext |
typedef struct IPAddressACL IPAddressACL |
typedef struct RTSPActionServerSetup RTSPActionServerSetup |
Enumeration Type Documentation
enum HTTPState |
Definition at line 77 of file ffserver.c.
enum IPAddressAction |
Enumerator | |
---|---|
IP_ALLOW | |
IP_DENY |
Definition at line 200 of file ffserver.c.
enum RedirType |
Enumerator | |
---|---|
REDIR_NONE | |
REDIR_ASX | |
REDIR_RAM | |
REDIR_ASF | |
REDIR_RTSP | |
REDIR_SDP |
Definition at line 1481 of file ffserver.c.
enum StreamType |
Enumerator | |
---|---|
STREAM_TYPE_LIVE | |
STREAM_TYPE_STATUS | |
STREAM_TYPE_REDIRECT |
Definition at line 194 of file ffserver.c.
Function Documentation
Definition at line 3540 of file ffserver.c.
Referenced by build_feed_streams().
|
static |
Definition at line 3507 of file ffserver.c.
Referenced by add_av_stream(), and build_file_streams().
|
static |
Definition at line 3859 of file ffserver.c.
Referenced by parse_ffconfig().
Definition at line 3691 of file ffserver.c.
Referenced by main().
Definition at line 3644 of file ffserver.c.
Referenced by main().
|
static |
Definition at line 854 of file ffserver.c.
Referenced by http_server(), and rtsp_cmd_teardown().
Definition at line 3835 of file ffserver.c.
Referenced by main().
|
static |
Definition at line 475 of file ffserver.c.
Referenced by compute_status().
|
static |
Definition at line 1457 of file ffserver.c.
Referenced by http_parse_request().
|
static |
Definition at line 1922 of file ffserver.c.
Referenced by http_parse_request().
|
static |
Definition at line 399 of file ffserver.c.
Referenced by http_vlog().
|
static |
Definition at line 3593 of file ffserver.c.
Referenced by build_file_streams().
|
static |
Definition at line 1120 of file ffserver.c.
Referenced by http_parse_request().
|
static |
Definition at line 331 of file ffserver.c.
Referenced by build_feed_streams(), and http_start_receive_data().
|
static |
Definition at line 356 of file ffserver.c.
Referenced by http_prepare_data().
|
static |
Definition at line 342 of file ffserver.c.
Referenced by http_receive_data(), and http_start_receive_data().
|
static |
Definition at line 4036 of file ffserver.c.
Referenced by parse_ffconfig().
|
static |
Definition at line 3983 of file ffserver.c.
Referenced by ffserver_opt_preset(), and parse_ffconfig().
|
static |
Definition at line 3993 of file ffserver.c.
Referenced by parse_ffconfig().
|
static |
Definition at line 3062 of file ffserver.c.
Referenced by find_rtp_session_with_url(), and rtsp_cmd_setup().
|
static |
Definition at line 3235 of file ffserver.c.
Referenced by rtsp_cmd_pause(), rtsp_cmd_play(), and rtsp_cmd_teardown().
|
static |
Definition at line 1167 of file ffserver.c.
Referenced by modify_current_stream().
|
static |
Definition at line 3076 of file ffserver.c.
Referenced by rtsp_cmd_setup().
|
static |
Definition at line 1912 of file ffserver.c.
Referenced by compute_status().
|
static |
Definition at line 1406 of file ffserver.c.
Referenced by validate_acl().
|
static |
Definition at line 1271 of file ffserver.c.
Referenced by parse_acl_row(), parse_dynamic_acl(), and parse_ffconfig().
|
static |
Definition at line 2242 of file ffserver.c.
Referenced by http_send_data().
|
static |
Definition at line 2234 of file ffserver.c.
Referenced by http_send_data().
|
static |
Definition at line 1253 of file ffserver.c.
Referenced by http_parse_request(), and rtsp_parse_request().
|
static |
Definition at line 4658 of file ffserver.c.
Referenced by main().
|
static |
Definition at line 943 of file ffserver.c.
Referenced by http_server().
Definition at line 439 of file ffserver.c.
Referenced by main().
|
static |
Definition at line 431 of file ffserver.c.
Referenced by build_feed_streams(), build_file_streams(), http_av_log(), http_parse_request(), http_prepare_data(), http_receive_data(), http_server(), http_start_receive_data(), log_connection(), main(), new_connection(), open_input_stream(), rtp_new_av_stream(), start_children(), and start_multicast().
|
static |
Definition at line 1491 of file ffserver.c.
Referenced by handle_connection().
|
static |
Definition at line 2257 of file ffserver.c.
Referenced by http_send_data().
|
static |
Definition at line 2652 of file ffserver.c.
Referenced by handle_connection().
|
static |
Definition at line 2487 of file ffserver.c.
Referenced by handle_connection().
|
static |
Definition at line 787 of file ffserver.c.
Referenced by new_connection().
|
static |
Definition at line 633 of file ffserver.c.
Referenced by main().
|
static |
Definition at line 2606 of file ffserver.c.
Referenced by http_parse_request().
|
static |
Definition at line 413 of file ffserver.c.
Referenced by http_av_log(), and http_log().
|
static |
Definition at line 3960 of file ffserver.c.
Referenced by parse_ffconfig().
|
static |
Definition at line 451 of file ffserver.c.
Referenced by http_server().
int main | ( | int | argc, |
char ** | argv | ||
) |
Definition at line 4705 of file ffserver.c.
|
static |
Definition at line 1204 of file ffserver.c.
Referenced by http_parse_request().
|
static |
Definition at line 804 of file ffserver.c.
Referenced by http_server().
|
static |
Definition at line 2164 of file ffserver.c.
Referenced by http_parse_request(), http_prepare_data(), rtsp_cmd_setup(), and start_multicast().
|
static |
Definition at line 3937 of file ffserver.c.
Referenced by ffserver_opt_preset(), and parse_ffconfig().
Definition at line 4683 of file ffserver.c.
|
static |
Definition at line 3947 of file ffserver.c.
Referenced by ffserver_opt_preset(), and parse_ffconfig().
|
static |
Definition at line 1303 of file ffserver.c.
Referenced by parse_dynamic_acl(), and parse_ffconfig().
|
static |
Definition at line 1369 of file ffserver.c.
Referenced by validate_acl().
|
static |
Definition at line 4066 of file ffserver.c.
Referenced by main().
|
static |
Definition at line 2959 of file ffserver.c.
Referenced by http_parse_request(), and rtsp_cmd_describe().
Definition at line 3580 of file ffserver.c.
Referenced by build_file_streams().
|
static |
Definition at line 4055 of file ffserver.c.
|
static |
Definition at line 366 of file ffserver.c.
Referenced by parse_acl_row(), and parse_ffconfig().
|
static |
Definition at line 3410 of file ffserver.c.
Referenced by rtsp_cmd_setup(), and start_multicast().
|
static |
Definition at line 3344 of file ffserver.c.
Referenced by rtsp_cmd_setup(), and start_multicast().
|
static |
Definition at line 3015 of file ffserver.c.
Referenced by rtsp_parse_request().
|
static |
Definition at line 3006 of file ffserver.c.
Referenced by rtsp_parse_request().
|
static |
Definition at line 3295 of file ffserver.c.
Referenced by rtsp_parse_request().
|
static |
Definition at line 3269 of file ffserver.c.
Referenced by rtsp_parse_request().
|
static |
Definition at line 3089 of file ffserver.c.
Referenced by rtsp_parse_request().
|
static |
Definition at line 3320 of file ffserver.c.
Referenced by rtsp_parse_request().
|
static |
Definition at line 2870 of file ffserver.c.
Referenced by handle_connection().
|
static |
Definition at line 2864 of file ffserver.c.
Referenced by rtsp_cmd_describe(), rtsp_cmd_pause(), rtsp_cmd_play(), rtsp_cmd_setup(), rtsp_cmd_teardown(), and rtsp_parse_request().
|
static |
Definition at line 2808 of file ffserver.c.
Referenced by rtsp_cmd_describe(), rtsp_cmd_pause(), rtsp_cmd_play(), rtsp_cmd_setup(), rtsp_cmd_teardown(), and rtsp_reply_error().
void show_help_default | ( | const char * | opt, |
const char * | arg | ||
) |
Per-fftool specific help handler.
Implemented in each fftool, called by show_help().
Definition at line 4689 of file ffserver.c.
Referenced by show_help().
|
static |
Definition at line 1244 of file ffserver.c.
Referenced by get_word().
|
static |
Definition at line 543 of file ffserver.c.
Referenced by http_server().
Definition at line 484 of file ffserver.c.
Referenced by http_server().
Definition at line 576 of file ffserver.c.
Referenced by http_server().
|
static |
Definition at line 773 of file ffserver.c.
Referenced by handle_connection(), and new_connection().
|
static |
Definition at line 461 of file ffserver.c.
Referenced by http_receive_data(), and http_send_data().
|
static |
Definition at line 1435 of file ffserver.c.
Referenced by http_parse_request().
|
static |
Definition at line 1418 of file ffserver.c.
Referenced by validate_acl().
Variable Documentation
|
static |
Definition at line 311 of file ffserver.c.
Referenced by main().
|
static |
Definition at line 325 of file ffserver.c.
Referenced by audio_read_packet(), check_keyboard_interaction(), compute_datarate(), get_server_clock(), handle_connection(), http_prepare_data(), http_server(), open_input_stream(), print_report(), start_wait_request(), transcode(), update_datarate(), and video_refresh().
|
static |
Definition at line 323 of file ffserver.c.
Referenced by close_connection(), compute_status(), http_parse_request(), and rtp_new_connection().
|
static |
Definition at line 313 of file ffserver.c.
Referenced by http_parse_request(), opt_debug(), parse_ffconfig(), and start_children().
|
static |
Definition at line 273 of file ffserver.c.
Referenced by parse_ffconfig().
|
static |
Definition at line 272 of file ffserver.c.
Referenced by close_connection(), compute_status(), http_server(), new_connection(), and rtp_new_connection().
|
static |
Definition at line 274 of file ffserver.c.
Referenced by compute_status(), http_parse_request(), parse_ffconfig(), and remove_stream().
|
static |
Definition at line 92 of file ffserver.c.
Referenced by compute_status().
|
static |
Definition at line 329 of file ffserver.c.
Referenced by http_vlog(), and main().
|
static |
Definition at line 271 of file ffserver.c.
Referenced by main(), opt_debug(), parse_ffconfig(), and transcode_init().
|
static |
Definition at line 322 of file ffserver.c.
Referenced by compute_status(), hls_open(), http_parse_request(), and parse_ffconfig().
|
static |
Definition at line 268 of file ffserver.c.
Referenced by http_server(), and parse_ffconfig().
|
static |
Definition at line 309 of file ffserver.c.
Referenced by main(), and start_children().
|
static |
Definition at line 269 of file ffserver.c.
Referenced by http_parse_request(), http_server(), and parse_ffconfig().
|
static |
Definition at line 320 of file ffserver.c.
Referenced by close_connection(), compute_status(), http_send_too_busy_reply(), new_connection(), and rtp_new_connection().
|
static |
Definition at line 319 of file ffserver.c.
Referenced by compute_status(), http_send_too_busy_reply(), new_connection(), parse_ffconfig(), and rtp_new_connection().
|
static |
Definition at line 318 of file ffserver.c.
Referenced by http_server(), and parse_ffconfig().
|
static |
Definition at line 315 of file ffserver.c.
Referenced by handle_child_exit(), and http_server().
|
static |
Definition at line 314 of file ffserver.c.
Referenced by start_children().
|
static |
Definition at line 75 of file ffserver.c.
const int program_birth_year = 2000 |
program birth year, defined by the program for show_banner()
Definition at line 73 of file ffserver.c.
Referenced by print_program_info().
const char program_name[] = "ffserver" |
program name, defined by the program for show_version().
Definition at line 72 of file ffserver.c.
Referenced by compute_status(), expand_filename_template(), init_report(), print_program_info(), show_help_default(), show_license(), and show_usage().
|
static |
Definition at line 327 of file ffserver.c.
Generated on Wed Apr 16 2025 06:53:26 for FFmpeg by
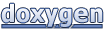