FFmpeg
|
rtsp.h
Go to the documentation of this file.
int interleaved_min
interleave ids, if TCP transport; each TCP/RTSP data packet starts with a '$', stream length and stre...
Definition: rtsp.h:92
Definition: mpegts.c:94
RTSPLowerTransport
Network layer over which RTP/etc packet data will be transported.
Definition: rtsp.h:37
int ff_rtsp_open_transport_ctx(AVFormatContext *s, RTSPStream *rtsp_st)
Open RTSP transport context.
Definition: rtsp.c:629
Definition: rtpdec.h:119
struct RTSPTransportField RTSPTransportField
This describes a single item in the "Transport:" line of one stream as negotiated by the SETUP RTSP c...
Definition: rtsp.h:41
int ff_rtsp_make_setup_request(AVFormatContext *s, const char *host, int port, int lower_transport, const char *real_challenge)
Do the SETUP requests for each stream for the chosen lower transport mode.
FFmpeg currently uses a custom build this text attempts to document some of its obscure features and options Makefile the full command issued by make and its output will be shown on the screen DESTDIR Destination directory for the install useful to prepare packages or install FFmpeg in cross environments Makefile builds all the libraries and the executables fate Run the fate test note you must have installed it fate list Will list all fate regression test targets install Install headers
Definition: build_system.txt:1
enum RTSPLowerTransport lower_transport
network layer transport protocol; e.g.
Definition: rtsp.h:120
Definition: rtsp.h:60
int ff_rtsp_connect(AVFormatContext *s)
Connect to the RTSP server and set up the individual media streams.
int get_parameter_supported
Whether the server supports the GET_PARAMETER method.
Definition: rtsp.h:358
int ttl
time-to-live value (required for multicast); the amount of HOPs that packets will be allowed to make ...
Definition: rtsp.h:108
AVOptions.
void ff_rtsp_parse_line(RTSPMessageHeader *reply, const char *buf, RTSPState *rt, const char *method)
URLContext * rtsp_hd_out
Additional output handle, used when input and output are done separately, eg for HTTP tunneling...
Definition: rtsp.h:327
Describe a single stream, as identified by a single m= line block in the SDP content.
Definition: rtsp.h:418
Custom IO - not a public option for lower_transport_mask, but set in the SDP demuxer based on a flag...
Definition: rtsp.h:45
RTSPServerType
Identify particular servers that require special handling, such as standards-incompliant "Transport:"...
Definition: rtsp.h:205
int ff_rtsp_parse_streaming_commands(AVFormatContext *s)
Parse RTSP commands (OPTIONS, PAUSE and TEARDOWN) during streaming in listen mode.
Definition: rtspdec.c:451
int ff_rtsp_send_cmd(AVFormatContext *s, const char *method, const char *url, const char *headers, RTSPMessageHeader *reply, unsigned char **content_ptr)
Send a command to the RTSP server and wait for the reply.
int ff_sdp_parse(AVFormatContext *s, const char *content)
Parse an SDP description of streams by populating an RTSPState struct within the AVFormatContext; als...
int64_t last_cmd_time
timestamp of the last RTSP command that we sent to the RTSP server.
Definition: rtsp.h:254
uint64_t asf_pb_pos
cache for position of the asf demuxer, since we load a new data packet in the bytecontext for each in...
Definition: rtsp.h:310
void * cur_transport_priv
RTSPStream->transport_priv of the last stream that we read a packet from.
Definition: rtsp.h:282
int timeout
The "timeout" comes as part of the server response to the "SETUP" command, in the "Session: <xyz>[;ti...
Definition: rtsp.h:171
HTTP tunneled - not a proper transport mode as such, only for use via AVOptions.
Definition: rtsp.h:42
This describes a single item in the "Transport:" line of one stream as negotiated by the SETUP RTSP c...
Definition: rtsp.h:87
struct MpegTSContext * ts
The following are used for parsing raw mpegts in udp.
Definition: rtsp.h:320
Definition: network.h:100
void ff_rtsp_undo_setup(AVFormatContext *s)
Undo the effect of ff_rtsp_make_setup_request, close the transport_priv and rtp_handle fields...
Definition: rtsp.c:569
struct RTSPMessageHeader RTSPMessageHeader
This describes the server response to each RTSP command.
Definition: url.h:41
int ff_rtsp_setup_output_streams(AVFormatContext *s, const char *addr)
Announce the stream to the server and set up the RTSPStream child objects for each media stream...
Definition: rtspenc.c:46
int ff_rtsp_tcp_read_packet(AVFormatContext *s, RTSPStream **prtsp_st, uint8_t *buf, int buf_size)
Receive one RTP packet from an TCP interleaved RTSP stream.
Definition: rtspdec.c:713
Definition: rtsp.h:209
int ff_rtsp_fetch_packet(AVFormatContext *s, AVPacket *pkt)
Receive one packet from the RTSPStreams set up in the AVFormatContext (which should contain a RTSPSta...
int ff_rtsp_send_cmd_with_content(AVFormatContext *s, const char *method, const char *url, const char *headers, RTSPMessageHeader *reply, unsigned char **content_ptr, const unsigned char *send_content, int send_content_length)
Send a command to the RTSP server and wait for the reply.
Main libavformat public API header.
int ff_rtsp_read_reply(AVFormatContext *s, RTSPMessageHeader *reply, unsigned char **content_ptr, int return_on_interleaved_data, const char *method)
Read a RTSP message from the server, or prepare to read data packets if we're reading data interleave...
void ff_rtsp_close_streams(AVFormatContext *s)
Close and free all streams within the RTSP (de)muxer.
Definition: rtsp.c:603
int ff_rtsp_send_cmd_async(AVFormatContext *s, const char *method, const char *url, const char *headers)
Send a command to the RTSP server without waiting for the reply.
struct RTSPStream RTSPStream
Describe a single stream, as identified by a single m= line block in the SDP content.
int ff_rtsp_setup_input_streams(AVFormatContext *s, RTSPMessageHeader *reply)
Get the description of the stream and set up the RTSPStream child objects.
Definition: rtspdec.c:567
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source unless expressly licensed for other it is provided solely for reference purposes pursuant to the terms of this License Source code may not be redistributed unless expressly provided for in this License INTELLECTUAL AND INDUSTRIAL PROPERTY RIGHTS Title to Software and all associated intellectual and industrial property rights is retained by UPF and or its licensors UPF warrants that the copyright in the Software is owned by it or licensed to it and that it has the power and authority to grant the Licence You shall keep intact all patent or trademark notices and all notices that refer to the Software and the Licence and to the disclaimer of warranties DISCLAIMER OF WARRANTY This Software is a work in progress It is not a finished work and may therefore contain defects or bugs inherent to this type of software development For this reason
Definition: MELODIA - License.txt:24
int server_port_min
UDP unicast server port range; the ports to which we should connect to receive unicast UDP RTP/RTCP d...
Definition: rtsp.h:104
void ff_rtsp_close_connections(AVFormatContext *s)
Close all connection handles within the RTSP (de)muxer.
int port_min
UDP multicast port range; the ports to which we should connect to receive multicast UDP data...
Definition: rtsp.h:96
int client_port_min
UDP client ports; these should be the local ports of the UDP RTP (and RTCP) sockets over which we rec...
Definition: rtsp.h:100
Generated on Mon Nov 18 2024 06:52:00 for FFmpeg by
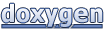