FFmpeg
|
mpegts.c
Go to the documentation of this file.
55 typedef int PESCallback(MpegTSFilter *f, const uint8_t *buf, int len, int is_start, int64_t pos);
135 {"compute_pcr", "Compute exact PCR for each transport stream packet.", offsetof(MpegTSContext, mpeg2ts_compute_pcr), AV_OPT_TYPE_INT,
330 crc_valid = !av_crc(av_crc_get_table(AV_CRC_32_IEEE), -1, tss->section_buf, tss->section_h_size);
1179 av_log(d->s, AV_LOG_ERROR, "Tag %x length violation new length %d bytes remaining %d\n", tag, len1, len);
1354 if (mp4_descr_count > 0 && (st->codec->codec_id == AV_CODEC_ID_AAC_LATM || st->request_probe>0) &&
1381 case 0x20: /* DVB subtitles (for the hard of hearing) with no monitor aspect ratio criticality */
1382 case 0x21: /* DVB subtitles (for the hard of hearing) for display on 4:3 aspect ratio monitor */
1383 case 0x22: /* DVB subtitles (for the hard of hearing) for display on 16:9 aspect ratio monitor */
1384 case 0x23: /* DVB subtitles (for the hard of hearing) for display on 2.21:1 aspect ratio monitor */
1385 case 0x24: /* DVB subtitles (for the hard of hearing) for display on a high definition monitor */
1386 case 0x25: /* DVB subtitles (for the hard of hearing) with plano-stereoscopic disparity for display on a high definition monitor */
1943 int dvhs_score= analyze(p->buf + TS_DVHS_PACKET_SIZE*i, TS_DVHS_PACKET_SIZE*left, TS_DVHS_PACKET_SIZE, NULL);
1944 int fec_score = analyze(p->buf + TS_FEC_PACKET_SIZE *i, TS_FEC_PACKET_SIZE *left, TS_FEC_PACKET_SIZE , NULL);
2184 pos = ((*ppos + ts->raw_packet_size - 1 - ts->pos47) / ts->raw_packet_size) * ts->raw_packet_size + ts->pos47;
2212 pos = ((*ppos + ts->raw_packet_size - 1 - ts->pos47) / ts->raw_packet_size) * ts->raw_packet_size + ts->pos47;
2226 av_add_index_entry(s->streams[pkt.stream_index], pkt.pos, pkt.dts, 0, 0, AVINDEX_KEYFRAME /* FIXME keyframe? */);
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
struct PESContext PESContext
Definition: libavcodec/avcodec.h:100
void av_buffer_unref(AVBufferRef **buf)
Free a given reference and automatically free the buffer if there are no more references to it...
Definition: libavutil/buffer.c:105
Definition: mpegts.h:86
Definition: mpegts.c:94
uint32_t av_crc(const AVCRC *ctx, uint32_t crc, const uint8_t *buffer, size_t length)
Calculate the CRC of a block.
Definition: crc.c:275
A dummy id pointing at the start of audio codecs.
Definition: libavcodec/avcodec.h:298
int av_add_index_entry(AVStream *st, int64_t pos, int64_t timestamp, int size, int distance, int flags)
Add an index entry into a sorted list.
Definition: libavformat/utils.c:1768
Definition: mpegts.c:151
Definition: libavcodec/avcodec.h:463
static int parse_MP4ODescrTag(MP4DescrParseContext *d, int64_t off, int len)
Definition: mpegts.c:1095
static int handle_packet(MpegTSContext *ts, const uint8_t *packet)
Definition: mpegts.c:1730
static void clear_program(MpegTSContext *ts, unsigned int programid)
Definition: mpegts.c:200
void avpriv_set_pts_info(AVStream *s, int pts_wrap_bits, unsigned int pts_num, unsigned int pts_den)
Set the time base and wrapping info for a given stream.
Definition: libavformat/utils.c:3952
Definition: opt.h:222
Definition: libavcodec/avcodec.h:173
int64_t avio_seek(AVIOContext *s, int64_t offset, int whence)
fseek() equivalent for AVIOContext.
Definition: aviobuf.c:199
#define AV_DISPOSITION_HEARING_IMPAIRED
stream for hearing impaired audiences
Definition: avformat.h:618
static void sdt_cb(MpegTSFilter *filter, const uint8_t *section, int section_len)
Definition: mpegts.c:1652
A dummy ID pointing at the start of various fake codecs.
Definition: libavcodec/avcodec.h:480
Definition: libavcodec/avcodec.h:115
int64_t avio_skip(AVIOContext *s, int64_t offset)
Skip given number of bytes forward.
Definition: aviobuf.c:256
void * av_realloc(void *ptr, size_t size)
Allocate or reallocate a block of memory.
Definition: mem.c:141
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
static int parse_section_header(SectionHeader *h, const uint8_t **pp, const uint8_t *p_end)
Definition: mpegts.c:521
Definition: avformat.h:456
static int mpegts_read_packet(AVFormatContext *s, AVPacket *pkt)
Definition: mpegts.c:2130
Definition: libavcodec/avcodec.h:130
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
const char * class_name
The name of the class; usually it is the same name as the context structure type to which the AVClass...
Definition: log.h:55
Definition: mpegts.c:88
void ff_read_frame_flush(AVFormatContext *s)
Flush the frame reader.
Definition: libavformat/utils.c:1663
void ff_reduce_index(AVFormatContext *s, int stream_index)
Ensure the index uses less memory than the maximum specified in AVFormatContext.max_index_size by dis...
Definition: libavformat/utils.c:1703
static void write_section_data(AVFormatContext *s, MpegTSFilter *tss1, const uint8_t *buf, int buf_size, int is_start)
Assemble PES packets out of TS packets, and then call the "section_cb" function when they are complet...
Definition: mpegts.c:295
Public dictionary API.
static int read_sl_header(PESContext *pes, SLConfigDescr *sl, const uint8_t *buf, int buf_size)
Definition: mpegts.c:742
void void avpriv_request_sample(void *avc, const char *msg,...) av_printf_format(2
Log a generic warning message about a missing feature.
MpegTSFilter * pids[NB_PID_MAX]
filters for various streams specified by PMT + for the PAT and PMT
Definition: mpegts.c:130
Definition: ffprobe.c:78
int stream_identifier
Stream Identifier This is the MPEG-TS stream identifier +1 0 means unknown.
Definition: avformat.h:805
static int64_t mpegts_get_dts(AVFormatContext *s, int stream_index, int64_t *ppos, int64_t pos_limit)
Definition: mpegts.c:2207
#define AVFMTCTX_NOHEADER
signal that no header is present (streams are added dynamically)
Definition: avformat.h:914
AVOptions.
const AVCRC * av_crc_get_table(AVCRCId crc_id)
Get an initialized standard CRC table.
Definition: crc.c:261
Definition: libavcodec/avcodec.h:219
static int parse_MP4ESDescrTag(MP4DescrParseContext *d, int64_t off, int len)
Definition: mpegts.c:1109
static int discard_pid(MpegTSContext *ts, unsigned int pid)
discard_pid() decides if the pid is to be discarded according to caller's programs selection ...
Definition: mpegts.c:266
static void add_pat_entry(MpegTSContext *ts, unsigned int programid)
Definition: mpegts.c:216
static int mpegts_push_data(MpegTSFilter *filter, const uint8_t *buf, int buf_size, int is_start, int64_t pos)
Definition: mpegts.c:809
Definition: libavcodec/avcodec.h:383
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
AVStream * avformat_new_stream(AVFormatContext *s, const AVCodec *c)
Add a new stream to a media file.
Definition: libavformat/utils.c:3362
the mask is usually to keep the same permissions Filters should remove permissions on reference they give to output whenever necessary It can be automatically done by setting the rej_perms field on the output pad Here are a few guidelines corresponding to common then the filter should push the output frames on the output link immediately As an exception to the previous rule if the input frame is enough to produce several output frames then the filter needs output only at least one per link The additional frames can be left buffered in the filter
Definition: filter_design.txt:201
Definition: libavcodec/avcodec.h:325
static void update_offsets(AVIOContext *pb, int64_t *off, int *len)
Definition: mpegts.c:1064
AVProgram * av_new_program(AVFormatContext *s, int id)
Definition: libavformat/utils.c:3421
bitstream reader API header.
Definition: mpegts.c:162
static av_always_inline int64_t avio_tell(AVIOContext *s)
ftell() equivalent for AVIOContext.
Definition: avio.h:248
enum AVDiscard discard
selects which program to discard and which to feed to the caller
Definition: avformat.h:891
static PESContext * add_pes_stream(MpegTSContext *ts, int pid, int pcr_pid)
Definition: mpegts.c:1007
int duration
Duration of this packet in AVStream->time_base units, 0 if unknown.
Definition: libavcodec/avcodec.h:1073
Definition: libavcodec/avcodec.h:486
int avio_read(AVIOContext *s, unsigned char *buf, int size)
Read size bytes from AVIOContext into buf.
Definition: aviobuf.c:478
static MpegTSFilter * mpegts_open_pes_filter(MpegTSContext *ts, unsigned int pid, PESCallback *pes_cb, void *opaque)
Definition: mpegts.c:375
static int init_MP4DescrParseContext(MP4DescrParseContext *d, AVFormatContext *s, const uint8_t *buf, unsigned size, Mp4Descr *descr, int max_descr_count)
Definition: mpegts.c:1042
Definition: libavcodec/avcodec.h:426
int av_new_packet(AVPacket *pkt, int size)
Allocate the payload of a packet and initialize its fields with default values.
Definition: avpacket.c:73
int ff_mp4_read_dec_config_descr(AVFormatContext *fc, AVStream *st, AVIOContext *pb)
Definition: isom.c:432
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
static void clear_avprogram(MpegTSContext *ts, unsigned int programid)
Definition: mpegts.c:186
static int mpegts_raw_read_packet(AVFormatContext *s, AVPacket *pkt)
Definition: mpegts.c:2086
union MpegTSFilter::@150 u
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: mpegts.c:150
Definition: libavcodec/avcodec.h:385
preferred ID for decoding MPEG audio layer 1, 2 or 3
Definition: libavcodec/avcodec.h:382
AVBufferRef * buf
A reference to the reference-counted buffer where the packet data is stored.
Definition: libavcodec/avcodec.h:1034
Definition: avutil.h:144
void ff_program_add_stream_index(AVFormatContext *ac, int progid, unsigned int idx)
Definition: libavformat/utils.c:3473
simple assert() macros that are a bit more flexible than ISO C assert().
FFmpeg Automated Testing Environment ************************************Table of Contents *****************FFmpeg Automated Testing Environment Introduction Using FATE from your FFmpeg source directory Submitting the results to the FFmpeg result aggregation server FATE makefile targets and variables Makefile targets Makefile variables Examples Introduction **************FATE is an extended regression suite on the client side and a means for results aggregation and presentation on the server side The first part of this document explains how you can use FATE from your FFmpeg source directory to test your ffmpeg binary The second part describes how you can run FATE to submit the results to FFmpeg s FATE server In any way you can have a look at the publicly viewable FATE results by visiting this as it can be seen if some test on some platform broke with their recent contribution This usually happens on the platforms the developers could not test on The second part of this document describes how you can run FATE to submit your results to FFmpeg s FATE server If you want to submit your results be sure to check that your combination of OS and compiler is not already listed on the above mentioned website In the third part you can find a comprehensive listing of FATE makefile targets and variables Using FATE from your FFmpeg source directory **********************************************If you want to run FATE on your machine you need to have the samples in place You can get the samples via the build target fate rsync Use this command from the top level source this will cause FATE to fail NOTE To use a custom wrapper to run the pass target exec to configure or set the TARGET_EXEC Make variable Submitting the results to the FFmpeg result aggregation server ****************************************************************To submit your results to the server you should run fate through the shell script tests fate sh from the FFmpeg sources This script needs to be invoked with a configuration file as its first argument tests fate sh path to fate_config A configuration file template with comments describing the individual configuration variables can be found at doc fate_config sh template Create a configuration that suits your based on the configuration template The slot configuration variable can be any string that is not yet used
Definition: fate.txt:34
static int64_t ff_parse_pes_pts(const uint8_t *buf)
Parse MPEG-PES five-byte timestamp.
Definition: mpeg.h:67
static int parse_MP4DecConfigDescrTag(MP4DescrParseContext *d, int64_t off, int len)
Definition: mpegts.c:1127
struct MpegTSPESFilter MpegTSPESFilter
MpegTSContext * ff_mpegts_parse_open(AVFormatContext *s)
Definition: mpegts.c:2241
unsigned char * buf
Buffer must have AVPROBE_PADDING_SIZE of extra allocated bytes filled with zero.
Definition: avformat.h:336
#define FF_INPUT_BUFFER_PADDING_SIZE
Required number of additionally allocated bytes at the end of the input bitstream for decoding...
Definition: libavcodec/avcodec.h:561
int ff_find_stream_index(AVFormatContext *s, int id)
Find stream index based on format-specific stream ID.
Definition: libavformat/utils.c:4091
int seekable
A combination of AVIO_SEEKABLE_ flags or 0 when the stream is not seekable.
Definition: avio.h:117
int PESCallback(MpegTSFilter *f, const uint8_t *buf, int len, int is_start, int64_t pos)
Definition: mpegts.c:55
static void set_pcr_pid(AVFormatContext *s, unsigned int programid, unsigned int pid)
Definition: mpegts.c:247
static int mp4_read_od(AVFormatContext *s, const uint8_t *buf, unsigned size, Mp4Descr *descr, int *descr_count, int max_descr_count)
Definition: mpegts.c:1230
Definition: mpegts.h:69
Definition: mpegts.c:57
struct SectionHeader SectionHeader
Definition: mpegts.c:50
Definition: mpegts.c:76
static int mpegts_set_stream_info(AVStream *st, PESContext *pes, uint32_t stream_type, uint32_t prog_reg_desc)
Definition: mpegts.c:636
static int parse_mp4_descr(MP4DescrParseContext *d, int64_t off, int len, int target_tag)
Definition: mpegts.c:1173
#define CHECK_COUNT
static int parse_MP4SLDescrTag(MP4DescrParseContext *d, int64_t off, int len)
Definition: mpegts.c:1140
#define CHECK_BLOCK
unsigned int probesize
decoding: size of data to probe; encoding: unused.
Definition: avformat.h:1039
int ff_mpegts_parse_packet(MpegTSContext *ts, AVPacket *pkt, const uint8_t *buf, int len)
Definition: mpegts.c:2260
Definition: mpegts.c:49
#define AV_DISPOSITION_VISUAL_IMPAIRED
stream for visual impaired audiences
Definition: avformat.h:619
FAKE codec to indicate a MPEG-4 Systems stream (only used by libavformat)
Definition: libavcodec/avcodec.h:494
static av_unused int64_t mpegts_get_pcr(AVFormatContext *s, int stream_index, int64_t *ppos, int64_t pos_limit)
Definition: mpegts.c:2177
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
AVBufferRef * av_buffer_alloc(int size)
Allocate an AVBuffer of the given size using av_malloc().
Definition: libavutil/buffer.c:65
static int parse_mp4_descr_arr(MP4DescrParseContext *d, int64_t off, int len)
Definition: mpegts.c:1073
Definition: libavcodec/avcodec.h:384
Definition: crc.h:34
Definition: libavcodec/avcodec.h:457
unsigned int codec_tag
fourcc (LSB first, so "ABCD" -> ('D'<<24) + ('C'<<16) + ('B'<<8) + 'A').
Definition: libavcodec/avcodec.h:1160
Definition: libavcodec/avcodec.h:422
int av_dict_set(AVDictionary **pm, const char *key, const char *value, int flags)
Set the given entry in *pm, overwriting an existing entry.
Definition: dict.c:62
static int parse_pcr(int64_t *ppcr_high, int *ppcr_low, const uint8_t *packet)
Definition: mpegts.c:1962
Definition: mpegts.c:66
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
static void pmt_cb(MpegTSFilter *filter, const uint8_t *section, int section_len)
Definition: mpegts.c:1436
#define AV_OPT_FLAG_DECODING_PARAM
a generic parameter which can be set by the user for demuxing or decoding
Definition: opt.h:282
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
refcounted data buffer API
static void mpegts_close_filter(MpegTSContext *ts, MpegTSFilter *filter)
Definition: mpegts.c:398
Definition: mpegts.c:154
This structure contains the data a format has to probe a file.
Definition: avformat.h:334
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
#define type
int av_read_frame(AVFormatContext *s, AVPacket *pkt)
Return the next frame of a stream.
Definition: libavformat/utils.c:1530
Definition: mpegts.c:463
void avpriv_report_missing_feature(void *avc, const char *msg,...) av_printf_format(2
Log a generic warning message about a missing feature.
Definition: libavcodec/avcodec.h:431
Definition: libavcodec/avcodec.h:190
Definition: libavcodec/avcodec.h:323
unsigned int end_of_section_reached
Definition: mpegts.c:71
int ff_parse_mpeg2_descriptor(AVFormatContext *fc, AVStream *st, int stream_type, const uint8_t **pp, const uint8_t *desc_list_end, Mp4Descr *mp4_descr, int mp4_descr_count, int pid, MpegTSContext *ts)
Parse an MPEG-2 descriptor.
Definition: mpegts.c:1307
#define AVPROBE_SCORE_MAX
maximum score, half of that is used for file-extension-based detection
Definition: avformat.h:340
Main libavformat public API header.
static void pat_cb(MpegTSFilter *filter, const uint8_t *section, int section_len)
Definition: mpegts.c:1590
int ff_mp4_read_descr(AVFormatContext *fc, AVIOContext *pb, int *tag)
Definition: isom.c:398
Definition: get_bits.h:54
Definition: mpegts.c:153
int ffio_init_context(AVIOContext *s, unsigned char *buffer, int buffer_size, int write_flag, void *opaque, int(*read_packet)(void *opaque, uint8_t *buf, int buf_size), int(*write_packet)(void *opaque, uint8_t *buf, int buf_size), int64_t(*seek)(void *opaque, int64_t offset, int whence))
Definition: aviobuf.c:71
int64_t start_time
Decoding: pts of the first frame of the stream in presentation order, in stream time base...
Definition: avformat.h:689
static int read_packet(AVFormatContext *s, uint8_t *buf, int raw_packet_size)
Definition: mpegts.c:1853
void av_init_packet(AVPacket *pkt)
Initialize optional fields of a packet with default values.
Definition: avpacket.c:56
struct AVInputFormat * iformat
Can only be iformat or oformat, not both at the same time.
Definition: avformat.h:957
struct MpegTSSectionFilter MpegTSSectionFilter
static MpegTSFilter * mpegts_open_section_filter(MpegTSContext *ts, unsigned int pid, SectionCallback *section_cb, void *opaque, int check_crc)
Definition: mpegts.c:343
FAKE codec to indicate a raw MPEG-2 TS stream (only used by libavformat)
Definition: libavcodec/avcodec.h:492
Definition: mpegts.c:1032
Definition: mpegts.c:152
static int analyze(const uint8_t *buf, int size, int packet_size, int *index)
Definition: mpegts.c:419
static void add_pid_to_pmt(MpegTSContext *ts, unsigned int programid, unsigned int pid)
Definition: mpegts.c:229
int64_t dts
Decompression timestamp in AVStream->time_base units; the time at which the packet is decompressed...
Definition: libavcodec/avcodec.h:1050
Definition: mpegts.c:550
int bit_rate
Decoding: total stream bitrate in bit/s, 0 if not available.
Definition: avformat.h:1016
Definition: avutil.h:143
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> out
Definition: audio_convert.c:194
static int parse_MP4IODescrTag(MP4DescrParseContext *d, int64_t off, int len)
Definition: mpegts.c:1083
static void m4sl_cb(MpegTSFilter *filter, const uint8_t *section, int section_len)
Definition: mpegts.c:1243
Definition: avutil.h:146
enum AVDiscard discard
Selects which packets can be discarded at will and do not need to be demuxed.
Definition: avformat.h:702
static void mpegts_find_stream_type(AVStream *st, uint32_t stream_type, const StreamType *types)
Definition: mpegts.c:618
int request_probe
stream probing state -1 -> probing finished 0 -> no probing requested rest -> perform probing with re...
Definition: avformat.h:834
int64_t pts
Presentation timestamp in AVStream->time_base units; the time at which the decompressed packet will b...
Definition: libavcodec/avcodec.h:1044
A dummy ID pointing at the start of subtitle codecs.
Definition: libavcodec/avcodec.h:455
void SectionCallback(MpegTSFilter *f, const uint8_t *buf, int len)
Definition: mpegts.c:62
static int mp4_read_iods(AVFormatContext *s, const uint8_t *buf, unsigned size, Mp4Descr *descr, int *descr_count, int max_descr_count)
Definition: mpegts.c:1217
Generated on Mon Nov 18 2024 06:51:58 for FFmpeg by
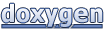