FFmpeg
|
isom.h
Go to the documentation of this file.
struct MOVSbgp MOVSbgp
Buffered I/O operations.
Definition: isom.h:95
struct MOVStts MOVStts
Definition: isom.h:147
int ff_mp4_read_dec_config_descr(AVFormatContext *fc, AVStream *st, AVIOContext *pb)
Definition: isom.c:432
struct MOVTrackExt MOVTrackExt
Definition: libavformat/internal.h:35
struct MOVStsc MOVStsc
Definition: isom.h:82
struct MOVStreamContext MOVStreamContext
struct MOVDref MOVDref
void ff_mov_write_chan(AVIOContext *pb, int64_t channel_layout)
Definition: isom.c:552
int ff_mov_read_esds(AVFormatContext *fc, AVIOContext *pb, MOVAtom atom)
Definition: mov.c:607
Definition: mov.c:62
struct MOVContext MOVContext
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
struct MOVAtom MOVAtom
int ff_mov_read_stsd_entries(MOVContext *c, AVIOContext *pb, int entries)
Definition: mov.c:1201
int ff_mp4_read_descr(AVFormatContext *fc, AVIOContext *pb, int *tag)
Definition: isom.c:398
Definition: libavformat/dv.c:43
FFmpeg Automated Testing Environment ************************************Table of Contents *****************FFmpeg Automated Testing Environment Introduction Using FATE from your FFmpeg source directory Submitting the results to the FFmpeg result aggregation server FATE makefile targets and variables Makefile targets Makefile variables Examples Introduction **************FATE is an extended regression suite on the client side and a means for results aggregation and presentation on the server side The first part of this document explains how you can use FATE from your FFmpeg source directory to test your ffmpeg binary The second part describes how you can run FATE to submit the results to FFmpeg s FATE server In any way you can have a look at the publicly viewable FATE results by visiting this as it can be seen if some test on some platform broke with their recent contribution This usually happens on the platforms the developers could not test on The second part of this document describes how you can run FATE to submit your results to FFmpeg s FATE server If you want to submit your results be sure to check that your combination of OS and compiler is not already listed on the above mentioned website In the third part you can find a comprehensive listing of FATE makefile targets and variables Using FATE from your FFmpeg source directory **********************************************If you want to run FATE on your machine you need to have the samples in place You can get the samples via the build target fate rsync Use this command from the top level source this will cause FATE to fail NOTE To use a custom wrapper to run the pass target exec to configure or set the TARGET_EXEC Make variable Submitting the results to the FFmpeg result aggregation server ****************************************************************To submit your results to the server you should run fate through the shell script tests fate sh from the FFmpeg sources This script needs to be invoked with a configuration file as its first argument tests fate sh path to fate_config A configuration file template with comments describing the individual configuration variables can be found at doc fate_config sh template Create a configuration that suits your based on the configuration template The slot configuration variable can be any string that is not yet but it is suggested that you name it adhering to the following pattern< arch >< os >< compiler >< compiler version > The configuration file itself will be sourced in a shell therefore all shell features may be used This enables you to setup the environment as you need it for your build For your first test runs the fate_recv variable should be empty or commented out This will run everything as normal except that it will omit the submission of the results to the server The following files should be present in $workdir as specified in the configuration it may help to try out the ssh command with one or more v options You should get detailed output concerning your SSH configuration and the authentication process The only thing left is to automate the execution of the fate sh script and the synchronisation of the samples directory FATE makefile targets and variables *************************************Makefile can be set to
Definition: fate.txt:142
unsigned int sample_size
may contain value calculated from stsd or value from stsz atom
Definition: isom.h:112
Definition: isom.h:72
enum AVCodecID ff_mov_get_lpcm_codec_id(int bps, int flags)
Compute codec id for 'lpcm' tag.
Definition: mov.c:1191
struct MOVFragment MOVFragment
Generated on Mon Apr 21 2025 06:52:40 for FFmpeg by
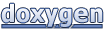