FFmpeg
|
avio_internal.h
Go to the documentation of this file.
56 * Rewind the AVIOContext using the specified buffer containing the first buf_size bytes of the file.
Buffered I/O operations.
int ffio_rewind_with_probe_data(AVIOContext *s, unsigned char **buf, int buf_size)
Rewind the AVIOContext using the specified buffer containing the first buf_size bytes of the file...
Definition: aviobuf.c:755
static int write_packet(AVFormatContext *s, AVPacket *pkt)
Definition: libavformat/assenc.c:63
static av_always_inline void ffio_wfourcc(AVIOContext *pb, const uint8_t *s)
Definition: avio_internal.h:50
int ffio_read_partial(AVIOContext *s, unsigned char *buf, int size)
Read size bytes from AVIOContext into buf.
Definition: aviobuf.c:526
void ffio_init_checksum(AVIOContext *s, unsigned long(*update_checksum)(unsigned long c, const uint8_t *p, unsigned int len), unsigned long checksum)
Definition: aviobuf.c:457
int ffio_open_dyn_packet_buf(AVIOContext **s, int max_packet_size)
Open a write only packetized memory stream with a maximum packet size of 'max_packet_size'.
Definition: aviobuf.c:981
unsigned long ff_crc04C11DB7_update(unsigned long checksum, const uint8_t *buf, unsigned int len)
Definition: aviobuf.c:443
Definition: url.h:41
int ffio_init_context(AVIOContext *s, unsigned char *buffer, int buffer_size, int write_flag, void *opaque, int(*read_packet)(void *opaque, uint8_t *buf, int buf_size), int(*write_packet)(void *opaque, uint8_t *buf, int buf_size), int64_t(*seek)(void *opaque, int64_t offset, int whence))
Definition: aviobuf.c:71
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
int ffio_fdopen(AVIOContext **s, URLContext *h)
Create and initialize a AVIOContext for accessing the resource referenced by the URLContext h...
Definition: aviobuf.c:694
unbuffered private I/O API
Generated on Mon Nov 18 2024 06:51:52 for FFmpeg by
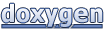