FFmpeg
|
libavformat/nuv.c
Go to the documentation of this file.
Definition: libavcodec/avcodec.h:100
Definition: libavformat/nuv.c:46
int av_add_index_entry(AVStream *st, int64_t pos, int64_t timestamp, int size, int distance, int flags)
Add an index entry into a sorted list.
Definition: libavformat/utils.c:1768
Definition: libavformat/nuv.c:35
enum AVCodecID ff_codec_get_id(const AVCodecTag *tags, unsigned int tag)
Definition: libavformat/utils.c:2614
Definition: libavformat/nuv.c:43
void av_shrink_packet(AVPacket *pkt, int size)
Reduce packet size, correctly zeroing padding.
Definition: avpacket.c:97
void avpriv_set_pts_info(AVStream *s, int pts_wrap_bits, unsigned int pts_num, unsigned int pts_den)
Set the time base and wrapping info for a given stream.
Definition: libavformat/utils.c:3952
int64_t avio_seek(AVIOContext *s, int64_t offset, int whence)
fseek() equivalent for AVIOContext.
Definition: aviobuf.c:199
int64_t avio_skip(AVIOContext *s, int64_t offset)
Skip given number of bytes forward.
Definition: aviobuf.c:256
Definition: avformat.h:456
Definition: libavcodec/avcodec.h:187
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
static av_always_inline double av_int2double(uint64_t i)
Reinterpret a 64-bit integer as a double.
Definition: intfloat.h:60
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
AVStream * avformat_new_stream(AVFormatContext *s, const AVCodec *c)
Add a new stream to a media file.
Definition: libavformat/utils.c:3362
Definition: libavformat/internal.h:35
int av_get_packet(AVIOContext *s, AVPacket *pkt, int size)
Allocate and read the payload of a packet and initialize its fields with default values.
Definition: libavformat/utils.c:328
static int get_codec_data(AVIOContext *pb, AVStream *vst, AVStream *ast, int myth)
read until we found all data needed for decoding
Definition: libavformat/nuv.c:68
static av_always_inline int64_t avio_tell(AVIOContext *s)
ftell() equivalent for AVIOContext.
Definition: avio.h:248
int bits_per_coded_sample
bits per sample/pixel from the demuxer (needed for huffyuv).
Definition: libavcodec/avcodec.h:2546
int avio_read(AVIOContext *s, unsigned char *buf, int size)
Read size bytes from AVIOContext into buf.
Definition: aviobuf.c:478
int av_new_packet(AVPacket *pkt, int size)
Allocate the payload of a packet and initialize its fields with default values.
Definition: avpacket.c:73
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
preferred ID for decoding MPEG audio layer 1, 2 or 3
Definition: libavcodec/avcodec.h:382
static int64_t nuv_read_dts(AVFormatContext *s, int stream_index, int64_t *ppos, int64_t pos_limit)
attempts to read a timestamp from stream at the given stream position
Definition: libavformat/nuv.c:329
Definition: avutil.h:144
unsigned char * buf
Buffer must have AVPROBE_PADDING_SIZE of extra allocated bytes filled with zero.
Definition: avformat.h:336
audio channel layout utility functions
AVRational av_d2q(double d, int max)
Convert a double precision floating point number to a rational.
Definition: rational.c:106
internal header for RIFF based (de)muxers do NOT include this in end user applications ...
unsigned int codec_tag
fourcc (LSB first, so "ABCD" -> ('D'<<24) + ('C'<<16) + ('B'<<8) + 'A').
Definition: libavcodec/avcodec.h:1160
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
enum AVCodecID ff_get_pcm_codec_id(int bps, int flt, int be, int sflags)
Select a PCM codec based on the given parameters.
Definition: libavformat/utils.c:2628
This structure contains the data a format has to probe a file.
Definition: avformat.h:334
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
static int nuv_resync(AVFormatContext *s, int64_t pos_limit)
looks for the string RTjjjjjjjjjj in the stream too resync reading
Definition: libavformat/nuv.c:312
Definition: libavformat/nuv.c:45
#define AVPROBE_SCORE_MAX
maximum score, half of that is used for file-extension-based detection
Definition: avformat.h:340
Main libavformat public API header.
enum AVCodecID ff_wav_codec_get_id(unsigned int tag, int bps)
Definition: libavcodec/avcodec.h:299
Definition: libavformat/nuv.c:44
Definition: libavformat/nuv.c:42
Definition: avutil.h:143
int64_t pts
Presentation timestamp in AVStream->time_base units; the time at which the decompressed packet will b...
Definition: libavcodec/avcodec.h:1044
Generated on Fri May 30 2025 06:53:59 for FFmpeg by
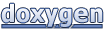