FFmpeg
|
riff.c
Go to the documentation of this file.
160 { AV_CODEC_ID_JPEGLS, MKTAG('M', 'J', 'L', 'S') }, /* JPEG-LS custom FOURCC for avi - encoder */
390 { AV_CODEC_ID_VORBIS, ('V'<<8)+'o' }, //HACK/FIXME, does vorbis in WAV/AVI have an (in)official id?
395 {AV_CODEC_ID_AC3, {0x2C,0x80,0x6D,0xE0,0x46,0xDB,0xCF,0x11,0xB4,0xD1,0x00,0x80,0x5F,0x6C,0xBB,0xEA}},
396 {AV_CODEC_ID_ATRAC3P, {0xBF,0xAA,0x23,0xE9,0x58,0xCB,0x71,0x44,0xA1,0x19,0xFF,0xFA,0x01,0xE4,0xCE,0x62}},
397 {AV_CODEC_ID_EAC3, {0xAF,0x87,0xFB,0xA7,0x02,0x2D,0xFB,0x42,0xA4,0xD4,0x05,0xCD,0x93,0x84,0x3B,0xDD}},
398 {AV_CODEC_ID_MP2, {0x2B,0x80,0x6D,0xE0,0x46,0xDB,0xCF,0x11,0xB4,0xD1,0x00,0x80,0x5F,0x6C,0xBB,0xEA}},
487 av_log(enc, AV_LOG_WARNING, "requested bits_per_coded_sample (%d) and actually stored (%d) differ\n", enc->bits_per_coded_sample, bps);
552 avio_wl16(pb, riff_extradata - riff_extradata_start + 22); /* 22 is WAVEFORMATEXTENSIBLE size */
572 void ff_put_bmp_header(AVIOContext *pb, AVCodecContext *enc, const AVCodecTag *tags, int for_asf)
583 avio_wl32(pb, (enc->width * enc->height * (enc->bits_per_coded_sample ? enc->bits_per_coded_sample : 24)+7) / 8);
595 void ff_parse_specific_params(AVCodecContext *stream, int *au_rate, int *au_ssize, int *au_scale)
Definition: libavcodec/avcodec.h:162
Definition: libavcodec/avcodec.h:367
int ff_read_riff_info(AVFormatContext *s, int64_t size)
Definition: start.py:1
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
Definition: libavcodec/avcodec.h:279
Definition: libavcodec/avcodec.h:100
Definition: libavcodec/avcodec.h:134
Definition: libavcodec/avcodec.h:368
Definition: libavcodec/avcodec.h:210
void ff_end_tag(AVIOContext *pb, int64_t start)
int64_t ff_start_tag(AVIOContext *pb, const char *tag)
Definition: dict.h:80
Definition: libavcodec/avcodec.h:344
Definition: libavcodec/avcodec.h:170
enum AVCodecID ff_codec_get_id(const AVCodecTag *tags, unsigned int tag)
Definition: libavformat/utils.c:2614
Definition: libavcodec/avcodec.h:164
void ff_parse_specific_params(AVCodecContext *stream, int *au_rate, int *au_ssize, int *au_scale)
Definition: libavcodec/avcodec.h:259
Definition: libavcodec/avcodec.h:236
Definition: libavcodec/avcodec.h:173
Definition: libavcodec/avcodec.h:157
int64_t avio_seek(AVIOContext *s, int64_t offset, int whence)
fseek() equivalent for AVIOContext.
Definition: aviobuf.c:199
Definition: libavcodec/avcodec.h:184
Definition: libavcodec/avcodec.h:112
Definition: libavcodec/avcodec.h:177
Definition: libavcodec/avcodec.h:393
Definition: libavcodec/avcodec.h:255
Definition: libavcodec/avcodec.h:115
int64_t avio_skip(AVIOContext *s, int64_t offset)
Skip given number of bytes forward.
Definition: aviobuf.c:256
Definition: libavcodec/avcodec.h:123
Definition: libavcodec/avcodec.h:260
AVDictionaryEntry * av_dict_get(AVDictionary *m, const char *key, const AVDictionaryEntry *prev, int flags)
Get a dictionary entry with matching key.
Definition: dict.c:39
Definition: libavcodec/avcodec.h:130
int block_align
number of bytes per packet if constant and known or 0 Used by some WAV based audio codecs...
Definition: libavcodec/avcodec.h:1898
Definition: libavcodec/avcodec.h:413
Definition: libavcodec/avcodec.h:346
Definition: libavcodec/avcodec.h:194
Definition: libavcodec/avcodec.h:154
Definition: libavcodec/avcodec.h:275
Definition: libavcodec/avcodec.h:215
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
Definition: libavcodec/avcodec.h:417
Definition: libavcodec/avcodec.h:273
Definition: libavcodec/avcodec.h:360
Definition: libavcodec/avcodec.h:402
Definition: libavcodec/avcodec.h:419
Definition: libavcodec/avcodec.h:149
Definition: libavcodec/avcodec.h:144
Definition: libavcodec/avcodec.h:117
Definition: libavcodec/avcodec.h:219
Definition: libavcodec/avcodec.h:412
Definition: libavcodec/avcodec.h:383
Definition: libavcodec/avcodec.h:113
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
Definition: libavformat/internal.h:35
Definition: libavcodec/avcodec.h:124
Definition: libavcodec/avcodec.h:434
Definition: libavcodec/avcodec.h:274
Definition: libavcodec/avcodec.h:128
static av_always_inline int64_t avio_tell(AVIOContext *s)
ftell() equivalent for AVIOContext.
Definition: avio.h:248
int bits_per_coded_sample
bits per sample/pixel from the demuxer (needed for huffyuv).
Definition: libavcodec/avcodec.h:2546
Definition: libavcodec/avcodec.h:110
Definition: metadata.h:34
void avio_write(AVIOContext *s, const unsigned char *buf, int size)
Definition: aviobuf.c:173
static av_always_inline void ffio_wfourcc(AVIOContext *pb, const uint8_t *s)
Definition: avio_internal.h:50
Definition: libavcodec/avcodec.h:189
int avio_read(AVIOContext *s, unsigned char *buf, int size)
Read size bytes from AVIOContext into buf.
Definition: aviobuf.c:478
Definition: libavcodec/avcodec.h:127
Definition: libavcodec/avcodec.h:103
Definition: libavcodec/avcodec.h:126
Definition: libavcodec/avcodec.h:306
Definition: libavcodec/avcodec.h:106
Definition: libavcodec/avcodec.h:250
int ff_get_bmp_header(AVIOContext *pb, AVStream *st, unsigned *esize)
Read BITMAPINFOHEADER structure and set AVStream codec width, height and bits_per_encoded_sample fiel...
Definition: libavcodec/avcodec.h:179
int av_get_bits_per_sample(enum AVCodecID codec_id)
Return codec bits per sample.
Definition: libavcodec/utils.c:2690
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
Definition: libavcodec/avcodec.h:418
Definition: libavcodec/avcodec.h:421
Definition: libavcodec/avcodec.h:132
int ff_get_wav_header(AVIOContext *pb, AVCodecContext *codec, int size)
void ff_riff_write_info_tag(AVIOContext *pb, const char *tag, const char *str)
Write a single RIFF info tag.
Definition: libavcodec/avcodec.h:385
preferred ID for decoding MPEG audio layer 1, 2 or 3
Definition: libavcodec/avcodec.h:382
Definition: libavcodec/avcodec.h:118
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
int64_t av_gcd(int64_t a, int64_t b)
Return the greatest common divisor of a and b.
Definition: mathematics.c:55
Definition: libavcodec/avcodec.h:182
Definition: libavcodec/avcodec.h:129
external API header
Definition: libavcodec/avcodec.h:227
Definition: libavcodec/avcodec.h:176
Definition: libavcodec/avcodec.h:137
#define FF_INPUT_BUFFER_PADDING_SIZE
Required number of additionally allocated bytes at the end of the input bitstream for decoding...
Definition: libavcodec/avcodec.h:561
Definition: libavcodec/avcodec.h:188
Definition: libavcodec/avcodec.h:156
Definition: libavcodec/avcodec.h:411
Definition: libavcodec/avcodec.h:178
Definition: libavcodec/avcodec.h:192
#define AV_DICT_DONT_STRDUP_VAL
Take ownership of a value that's been allocated with av_malloc() and chilren.
Definition: dict.h:72
Definition: libavcodec/avcodec.h:268
Definition: libavcodec/avcodec.h:256
Definition: libavcodec/avcodec.h:146
Definition: libavcodec/avcodec.h:121
internal header for RIFF based (de)muxers do NOT include this in end user applications ...
Definition: libavcodec/avcodec.h:159
Definition: libavcodec/avcodec.h:107
Definition: libavcodec/avcodec.h:284
Definition: libavcodec/avcodec.h:248
Definition: libavcodec/avcodec.h:388
Definition: libavcodec/avcodec.h:241
Definition: libavcodec/avcodec.h:243
Definition: libavcodec/avcodec.h:266
Definition: libavcodec/avcodec.h:343
Definition: libavcodec/avcodec.h:125
Definition: libavcodec/avcodec.h:265
Definition: libavcodec/avcodec.h:216
Definition: libavcodec/avcodec.h:254
Definition: libavcodec/avcodec.h:193
int avio_put_str(AVIOContext *s, const char *str)
Write a NULL-terminated string.
Definition: aviobuf.c:307
Definition: libavcodec/avcodec.h:145
Definition: libavcodec/avcodec.h:304
int frame_size
Number of samples per channel in an audio frame.
Definition: libavcodec/avcodec.h:1881
Definition: libavcodec/avcodec.h:139
Definition: libavcodec/avcodec.h:133
Definition: libavcodec/avcodec.h:120
Definition: libavcodec/avcodec.h:384
Definition: libavcodec/avcodec.h:294
Definition: libavcodec/avcodec.h:447
Definition: libavcodec/avcodec.h:305
unsigned int codec_tag
fourcc (LSB first, so "ABCD" -> ('D'<<24) + ('C'<<16) + ('B'<<8) + 'A').
Definition: libavcodec/avcodec.h:1160
Definition: libavcodec/avcodec.h:278
Definition: libavcodec/avcodec.h:422
int av_dict_set(AVDictionary **pm, const char *key, const char *value, int flags)
Set the given entry in *pm, overwriting an existing entry.
Definition: dict.c:62
void ff_riff_write_info(AVFormatContext *s)
Write all recognized RIFF tags from s->metadata.
Definition: libavcodec/avcodec.h:163
Definition: libavcodec/avcodec.h:196
enum AVCodecID ff_get_pcm_codec_id(int bps, int flt, int be, int sflags)
Select a PCM codec based on the given parameters.
Definition: libavformat/utils.c:2628
Definition: libavcodec/avcodec.h:258
Definition: libavcodec/avcodec.h:267
Definition: libavcodec/avcodec.h:270
Definition: libavcodec/avcodec.h:311
Definition: libavcodec/avcodec.h:381
Definition: libavcodec/avcodec.h:420
Definition: libavcodec/avcodec.h:122
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
int av_get_audio_frame_duration(AVCodecContext *avctx, int frame_bytes)
Return audio frame duration.
Definition: libavcodec/utils.c:2708
Definition: libavcodec/avcodec.h:322
Definition: riff.h:75
Definition: libavcodec/avcodec.h:281
void ff_put_bmp_header(AVIOContext *pb, AVCodecContext *enc, const AVCodecTag *tags, int for_asf)
Definition: libavcodec/avcodec.h:231
Definition: libavcodec/avcodec.h:230
Definition: libavcodec/avcodec.h:431
Definition: libavcodec/avcodec.h:190
Definition: libavcodec/avcodec.h:191
Definition: libavcodec/avcodec.h:288
Definition: libavcodec/avcodec.h:320
Definition: libavcodec/avcodec.h:136
Definition: libavcodec/avcodec.h:408
Main libavformat public API header.
Definition: libavcodec/avcodec.h:174
Definition: libavcodec/avcodec.h:283
enum AVCodecID ff_codec_guid_get_id(const AVCodecGuid *guids, ff_asf_guid guid)
enum AVCodecID ff_wav_codec_get_id(unsigned int tag, int bps)
Definition: libavcodec/avcodec.h:264
int ff_put_wav_header(AVIOContext *pb, AVCodecContext *enc)
Definition: libavcodec/avcodec.h:299
Definition: libavcodec/avcodec.h:233
void ff_get_guid(AVIOContext *s, ff_asf_guid *g)
void ff_metadata_conv(AVDictionary **pm, const AVMetadataConv *d_conv, const AVMetadataConv *s_conv)
Definition: libavformat/metadata.c:26
Definition: libavcodec/avcodec.h:114
Definition: libavcodec/avcodec.h:119
Definition: libavcodec/avcodec.h:138
Definition: libavcodec/avcodec.h:160
Definition: libavcodec/avcodec.h:338
Definition: libavcodec/avcodec.h:285
Definition: libavcodec/avcodec.h:214
Definition: libavcodec/avcodec.h:153
Definition: libavcodec/avcodec.h:440
Definition: libavcodec/avcodec.h:389
Definition: libavcodec/avcodec.h:446
Definition: libavcodec/avcodec.h:316
Definition: libavcodec/avcodec.h:175
Definition: avutil.h:143
Definition: libavcodec/avcodec.h:307
Definition: libavcodec/avcodec.h:116
Definition: libavcodec/avcodec.h:226
Definition: avutil.h:146
Definition: libavcodec/avcodec.h:195
Definition: libavcodec/avcodec.h:423
Definition: libavcodec/avcodec.h:131
Definition: libavcodec/avcodec.h:345
Definition: libavcodec/avcodec.h:180
Definition: libavcodec/avcodec.h:135
static av_always_inline int ff_guidcmp(const void *g1, const void *g2)
Definition: riff.h:68
Definition: libavcodec/avcodec.h:148
Definition: libavcodec/avcodec.h:386
Definition: libavcodec/avcodec.h:269
Generated on Fri Aug 1 2025 06:53:45 for FFmpeg by
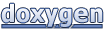