FFmpeg
|
cinepak.c
Go to the documentation of this file.
73 uint32_t pal[256];
Definition: cinepak.c:50
void void avpriv_request_sample(void *avc, const char *msg,...) av_printf_format(2
Log a generic warning message about a missing feature.
static int cinepak_decode_strip(CinepakContext *s, cvid_strip *strip, const uint8_t *data, int size)
Definition: cinepak.c:266
static int cinepak_decode_frame(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: cinepak.c:431
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
int bits_per_coded_sample
bits per sample/pixel from the demuxer (needed for huffyuv).
Definition: libavcodec/avcodec.h:2546
Definition: libavcodec/avcodec.h:918
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
external API header
int ff_reget_buffer(AVCodecContext *avctx, AVFrame *frame)
Identical in function to av_frame_make_writable(), except it uses ff_get_buffer() to allocate the buf...
Definition: libavcodec/utils.c:868
Definition: libavcodec/avcodec.h:146
static int cinepak_decode_vectors(CinepakContext *s, cvid_strip *strip, int chunk_id, int size, const uint8_t *data)
Definition: cinepak.c:132
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
static av_cold int cinepak_decode_init(AVCodecContext *avctx)
Definition: cinepak.c:405
static void cinepak_decode_codebook(cvid_codebook *codebook, int chunk_id, int size, const uint8_t *data)
Definition: cinepak.c:76
int palette_has_changed
Tell user application that palette has changed from previous frame.
Definition: frame.h:280
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
AVFrame * av_frame_alloc(void)
Allocate an AVFrame and set its fields to default values.
Definition: frame.c:95
int av_frame_ref(AVFrame *dst, AVFrame *src)
Setup a new reference to the data described by an given frame.
Definition: frame.c:228
common internal api header.
common internal and external API header
Definition: cinepak.c:58
void av_frame_free(AVFrame **frame)
Free the frame and any dynamically allocated objects in it, e.g.
Definition: frame.c:108
Definition: avutil.h:143
uint8_t * av_packet_get_side_data(AVPacket *pkt, enum AVPacketSideDataType type, int *size)
Get side information from packet.
Definition: avpacket.c:289
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
struct CinepakContext CinepakContext
static av_cold int cinepak_decode_end(AVCodecContext *avctx)
Definition: cinepak.c:469
Generated on Fri Dec 20 2024 06:55:59 for FFmpeg by
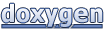