FFmpeg
|
avidec.c
Go to the documentation of this file.
81 { "use_odml", "use odml index", offsetof(AVIContext, use_odml), AV_OPT_TYPE_INT, {.i64 = 1}, -1, 1, AV_OPT_FLAG_DECODING_PARAM},
529 av_log(s, AV_LOG_WARNING, "scale/rate is %u/%u which is invalid. (This file has been generated by broken software.)\n", ast->scale, ast->rate);
647 st->need_parsing = AVSTREAM_PARSE_HEADERS; // This is needed to get the pict type which is necessary for generating correct pts.
651 st->codec->extradata= av_realloc_f(st->codec->extradata, 1, st->codec->extradata_size + FF_INPUT_BUFFER_PADDING_SIZE);
665 av_log(s, AV_LOG_WARNING, "sample size (%d) != block align (%d)\n", ast->sample_size, st->codec->block_align);
689 if(st->codec->codec_id == AV_CODEC_ID_AAC && ast->dshow_block_align <= 4 && ast->dshow_block_align) {
690 av_log(s, AV_LOG_DEBUG, "overriding invalid dshow_block_align of %d\n", ast->dshow_block_align);
693 if(st->codec->codec_id == AV_CODEC_ID_AAC && ast->dshow_block_align == 1024 && ast->sample_size == 1024 ||
694 st->codec->codec_id == AV_CODEC_ID_AAC && ast->dshow_block_align == 4096 && ast->sample_size == 4096 ||
695 st->codec->codec_id == AV_CODEC_ID_MP3 && ast->dshow_block_align == 1152 && ast->sample_size == 1152) {
715 if (stream_index >= (unsigned)s->nb_streams || s->streams[stream_index]->codec->extradata_size) {
922 * @param exit_early set to 1 to just gather packet position without making the changes needed to actually read & return the packet
1008 /*|| (st->discard >= AVDISCARD_NONKEY && !(pkt->flags & AV_PKT_FLAG_KEY))*/ //FIXME needs a little reordering
1047 if(!st->index_entries || !st->nb_index_entries || st->index_entries[st->nb_index_entries - 1].pos < pos){
1391 min_dts = FFMIN(min_dts, av_rescale_q(st->index_entries[idx[i]].timestamp, st->time_base, AV_TIME_BASE_Q));
1395 max_dts = FFMAX(max_dts, av_rescale_q(st->index_entries[idx[i]-1].timestamp, st->time_base, AV_TIME_BASE_Q));
1482 av_log(s, AV_LOG_DEBUG, "Failed to find timestamp %"PRId64 " in index %"PRId64 " .. %"PRId64 "\n",
1534 flags | AVSEEK_FLAG_BACKWARD | (st2->codec->codec_type != AVMEDIA_TYPE_VIDEO ? AVSEEK_FLAG_ANY : 0));
1550 flags | AVSEEK_FLAG_BACKWARD | (st2->codec->codec_type != AVMEDIA_TYPE_VIDEO ? AVSEEK_FLAG_ANY : 0));
int ff_read_riff_info(AVFormatContext *s, int64_t size)
static AVStream * get_subtitle_pkt(AVFormatContext *s, AVStream *next_st, AVPacket *pkt)
Definition: avidec.c:878
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
full parsing and interpolation of timestamps for frames not starting on a packet boundary ...
Definition: avformat.h:584
unsigned int stream_codec_tag
fourcc from the AVI stream header (LSB first, so "ABCD" -> ('D'<<24) + ('C'<<16) + ('B'<<8) + 'A')...
Definition: libavcodec/avcodec.h:1168
Definition: libavcodec/avcodec.h:100
void ff_metadata_conv_ctx(AVFormatContext *ctx, const AVMetadataConv *d_conv, const AVMetadataConv *s_conv)
Definition: libavformat/metadata.c:59
Definition: libavcodec/avcodec.h:210
int av_add_index_entry(AVStream *st, int64_t pos, int64_t timestamp, int size, int distance, int flags)
Add an index entry into a sorted list.
Definition: libavformat/utils.c:1768
enum AVCodecID ff_codec_get_id(const AVCodecTag *tags, unsigned int tag)
Definition: libavformat/utils.c:2614
void * av_realloc_f(void *ptr, size_t nelem, size_t elsize)
Allocate or reallocate a block of memory.
Definition: mem.c:168
unsigned char * buf_end
End of the data, may be less than buffer+buffer_size if the read function returned less data than req...
Definition: avio.h:85
int avformat_open_input(AVFormatContext **ps, const char *filename, AVInputFormat *fmt, AVDictionary **options)
Open an input stream and read the header.
Definition: libavformat/utils.c:614
void avpriv_set_pts_info(AVStream *s, int pts_wrap_bits, unsigned int pts_num, unsigned int pts_den)
Set the time base and wrapping info for a given stream.
Definition: libavformat/utils.c:3952
if max(w)>1 w=0.9 *w/max(w)
Definition: opt.h:222
static int read_seek(AVFormatContext *ctx, int stream_index, int64_t timestamp, int flags)
Definition: libcdio.c:153
int dshow_block_align
block align variable used to emulate bugs in the MS dshow demuxer
Definition: avidec.c:51
int64_t avio_seek(AVIOContext *s, int64_t offset, int whence)
fseek() equivalent for AVIOContext.
Definition: aviobuf.c:199
static int sync(AVFormatContext *s, uint8_t *header)
Read input until we find the next ident.
Definition: lxfdec.c:85
AVIndexEntry * index_entries
Only used if the format does not support seeking natively.
Definition: avformat.h:822
Definition: libavcodec/avcodec.h:115
int64_t avio_skip(AVIOContext *s, int64_t offset)
Skip given number of bytes forward.
Definition: aviobuf.c:256
struct AVStream::@125 * info
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
Definition: avidec.c:60
Definition: avformat.h:456
int avio_get_str16le(AVIOContext *pb, int maxlen, char *buf, int buflen)
Read a UTF-16 string from pb and convert it to UTF-8.
int block_align
number of bytes per packet if constant and known or 0 Used by some WAV based audio codecs...
Definition: libavcodec/avcodec.h:1898
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
const char * class_name
The name of the class; usually it is the same name as the context structure type to which the AVClass...
Definition: log.h:55
Public dictionary API.
AVOptions.
Definition: libavcodec/avcodec.h:383
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
AVStream * avformat_new_stream(AVFormatContext *s, const AVCodec *c)
Add a new stream to a media file.
Definition: libavformat/utils.c:3362
AVFormatContext * avformat_alloc_context(void)
Allocate an AVFormatContext.
Definition: libavformat/options.c:106
DVDemuxContext * avpriv_dv_init_demux(AVFormatContext *s)
Definition: libavformat/dv.c:317
int av_get_packet(AVIOContext *s, AVPacket *pkt, int size)
Allocate and read the payload of a packet and initialize its fields with default values.
Definition: libavformat/utils.c:328
static av_always_inline int64_t avio_tell(AVIOContext *s)
ftell() equivalent for AVIOContext.
Definition: avio.h:248
int bits_per_coded_sample
bits per sample/pixel from the demuxer (needed for huffyuv).
Definition: libavcodec/avcodec.h:2546
Definition: metadata.h:34
int avio_read(AVIOContext *s, unsigned char *buf, int size)
Read size bytes from AVIOContext into buf.
Definition: aviobuf.c:478
Definition: avformat.h:581
int64_t av_rescale_q(int64_t a, AVRational bq, AVRational cq)
Rescale a 64-bit integer by 2 rational numbers.
Definition: mathematics.c:130
Definition: log.h:33
AVIOContext * avio_alloc_context(unsigned char *buffer, int buffer_size, int write_flag, void *opaque, int(*read_packet)(void *opaque, uint8_t *buf, int buf_size), int(*write_packet)(void *opaque, uint8_t *buf, int buf_size), int64_t(*seek)(void *opaque, int64_t offset, int whence))
Allocate and initialize an AVIOContext for buffered I/O.
Definition: aviobuf.c:109
Definition: dict.c:28
int ff_get_bmp_header(AVIOContext *pb, AVStream *st, unsigned *esize)
Read BITMAPINFOHEADER structure and set AVStream codec width, height and bits_per_encoded_sample fiel...
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
Definition: libavcodec/avcodec.h:918
int av_index_search_timestamp(AVStream *st, int64_t timestamp, int flags)
Get the index for a specific timestamp.
Definition: libavformat/utils.c:1811
int ff_get_wav_header(AVIOContext *pb, AVCodecContext *codec, int size)
int64_t timestamp
Timestamp in AVStream.time_base units, preferably the time from which on correctly decoded frames are...
Definition: avformat.h:593
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
AVRational av_div_q(AVRational b, AVRational c)
Divide one rational by another.
Definition: rational.c:88
static int read_braindead_odml_indx(AVFormatContext *s, int frame_num)
Definition: avidec.c:152
preferred ID for decoding MPEG audio layer 1, 2 or 3
Definition: libavcodec/avcodec.h:382
AVBufferRef * buf
A reference to the reference-counted buffer where the packet data is stored.
Definition: libavcodec/avcodec.h:1034
Definition: avutil.h:144
static int avi_read_tag(AVFormatContext *s, AVStream *st, uint32_t tag, uint32_t size)
Definition: avidec.c:275
simple assert() macros that are a bit more flexible than ISO C assert().
AVInputFormat * av_probe_input_format2(AVProbeData *pd, int is_opened, int *score_max)
Guess the file format.
Definition: libavformat/utils.c:398
unsigned char * buf
Buffer must have AVPROBE_PADDING_SIZE of extra allocated bytes filled with zero.
Definition: avformat.h:336
#define FF_INPUT_BUFFER_PADDING_SIZE
Required number of additionally allocated bytes at the end of the input bitstream for decoding...
Definition: libavcodec/avcodec.h:561
int seekable
A combination of AVIO_SEEKABLE_ flags or 0 when the stream is not seekable.
Definition: avio.h:117
int64_t av_rescale(int64_t a, int64_t b, int64_t c)
Rescale a 64-bit integer with rounding to nearest.
Definition: mathematics.c:118
void ff_dv_offset_reset(DVDemuxContext *c, int64_t frame_offset)
Definition: libavformat/dv.c:426
int av_strcasecmp(const char *a, const char *b)
Locale-independent case-insensitive compare.
Definition: avstring.c:212
#define AV_DICT_DONT_STRDUP_VAL
Take ownership of a value that's been allocated with av_malloc() and chilren.
Definition: dict.h:72
Definition: avformat.h:591
internal header for RIFF based (de)muxers do NOT include this in end user applications ...
static void seek_subtitle(AVStream *st, AVStream *st2, int64_t timestamp)
Definition: avidec.c:1452
#define AVFMT_FLAG_SORT_DTS
try to interleave outputted packets by dts (using this flag can slow demuxing down) ...
Definition: avformat.h:1032
Definition: libavcodec/avcodec.h:459
int ff_read_packet(AVFormatContext *s, AVPacket *pkt)
Read a transport packet from a media file.
Definition: libavformat/utils.c:769
static int avi_read_packet(AVFormatContext *s, AVPacket *pkt)
Definition: avidec.c:1061
int prefix
normally 'd'<<8 + 'c' or 'w'<<8 + 'b'
Definition: avidec.c:47
struct AVIStream AVIStream
int avpriv_dv_produce_packet(DVDemuxContext *c, AVPacket *pkt, uint8_t *buf, int buf_size, int64_t pos)
Definition: libavformat/dv.c:357
unsigned int codec_tag
fourcc (LSB first, so "ABCD" -> ('D'<<24) + ('C'<<16) + ('B'<<8) + 'A').
Definition: libavcodec/avcodec.h:1160
int av_dict_set(AVDictionary **pm, const char *key, const char *value, int flags)
Set the given entry in *pm, overwriting an existing entry.
Definition: dict.c:62
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
#define AV_OPT_FLAG_DECODING_PARAM
a generic parameter which can be set by the user for demuxing or decoding
Definition: opt.h:282
byte swapping routines
discard useless packets like 0 size packets in avi
Definition: libavcodec/avcodec.h:595
This structure contains the data a format has to probe a file.
Definition: avformat.h:334
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
int avformat_seek_file(AVFormatContext *s, int stream_index, int64_t min_ts, int64_t ts, int64_t max_ts, int flags)
Seek to timestamp ts.
Definition: libavformat/utils.c:2146
Definition: libavformat/dv.c:43
#define AVPROBE_SCORE_MAX
maximum score, half of that is used for file-extension-based detection
Definition: avformat.h:340
Main libavformat public API header.
static void avi_metadata_creation_time(AVDictionary **metadata, char *date)
Definition: avidec.c:299
int64_t start_time
Decoding: pts of the first frame of the stream in presentation order, in stream time base...
Definition: avformat.h:689
int error_recognition
Error recognition; higher values will detect more errors but may misdetect some more or less valid pa...
Definition: avformat.h:1114
Definition: avidec.c:35
struct AVProbeData AVProbeData
This structure contains the data a format has to probe a file.
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
int avpriv_dv_get_packet(DVDemuxContext *c, AVPacket *pkt)
Definition: libavformat/dv.c:340
void avformat_close_input(AVFormatContext **s)
Close an opened input AVFormatContext.
Definition: libavformat/utils.c:3329
int64_t dts
Decompression timestamp in AVStream->time_base units; the time at which the packet is decompressed...
Definition: libavcodec/avcodec.h:1050
static int avi_read_seek(AVFormatContext *s, int stream_index, int64_t timestamp, int flags)
Definition: avidec.c:1462
int64_t duration
Decoding: duration of the stream, in AV_TIME_BASE fractional seconds.
Definition: avformat.h:1009
Definition: avutil.h:143
uint8_t * av_packet_new_side_data(AVPacket *pkt, enum AVPacketSideDataType type, int size)
Allocate new information of a packet.
Definition: avpacket.c:264
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: avformat.h:679
Definition: avutil.h:146
enum AVDiscard discard
Selects which packets can be discarded at will and do not need to be demuxed.
Definition: avformat.h:702
int request_probe
stream probing state -1 -> probing finished 0 -> no probing requested rest -> perform probing with re...
Definition: avformat.h:834
Definition: libavcodec/avcodec.h:377
Generated on Wed May 7 2025 06:52:38 for FFmpeg by
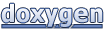