FFmpeg
|
libvpxenc.c
Go to the documentation of this file.
73 #define VP8F_ERROR_RESILIENT 0x00000001 ///< Enable measures appropriate for streaming over lossy links
628 { "cpu-used", "Quality/Speed ratio modifier", OFFSET(cpu_used), AV_OPT_TYPE_INT, {.i64 = INT_MIN}, INT_MIN, INT_MAX, VE},
632 "alternate reference frame selection", OFFSET(lag_in_frames), AV_OPT_TYPE_INT, {.i64 = -1}, -1, INT_MAX, VE},
633 { "arnr-maxframes", "altref noise reduction max frame count", OFFSET(arnr_max_frames), AV_OPT_TYPE_INT, {.i64 = -1}, -1, INT_MAX, VE},
634 { "arnr-strength", "altref noise reduction filter strength", OFFSET(arnr_strength), AV_OPT_TYPE_INT, {.i64 = -1}, -1, INT_MAX, VE},
635 { "arnr-type", "altref noise reduction filter type", OFFSET(arnr_type), AV_OPT_TYPE_INT, {.i64 = -1}, -1, INT_MAX, VE, "arnr_type"},
639 { "deadline", "Time to spend encoding, in microseconds.", OFFSET(deadline), AV_OPT_TYPE_INT, {.i64 = VPX_DL_GOOD_QUALITY}, INT_MIN, INT_MAX, VE, "quality"},
643 { "error-resilient", "Error resilience configuration", OFFSET(error_resilient), AV_OPT_TYPE_FLAGS, {.i64 = 0}, INT_MIN, INT_MAX, VE, "er"},
644 { "max-intra-rate", "Maximum I-frame bitrate (pct) 0=unlimited", OFFSET(max_intra_rate), AV_OPT_TYPE_INT, {.i64 = -1}, -1, INT_MAX, VE},
646 { "default", "Improve resiliency against losses of whole frames", 0, AV_OPT_TYPE_CONST, {.i64 = VPX_ERROR_RESILIENT_DEFAULT}, 0, 0, VE, "er"},
650 " is still done over the partition boundary.", 0, AV_OPT_TYPE_CONST, {.i64 = VPX_ERROR_RESILIENT_PARTITIONS}, 0, 0, VE, "er"},
653 {"quality", "", offsetof(VP8Context, deadline), AV_OPT_TYPE_INT, {.i64 = VPX_DL_GOOD_QUALITY}, INT_MIN, INT_MAX, VE, "quality"},
654 {"vp8flags", "", offsetof(VP8Context, flags), FF_OPT_TYPE_FLAGS, {.i64 = 0}, 0, UINT_MAX, VE, "flags"},
655 {"error_resilient", "enable error resilience", 0, FF_OPT_TYPE_CONST, {.dbl = VP8F_ERROR_RESILIENT}, INT_MIN, INT_MAX, VE, "flags"},
656 {"altref", "enable use of alternate reference frames (VP8/2-pass only)", 0, FF_OPT_TYPE_CONST, {.dbl = VP8F_AUTO_ALT_REF}, INT_MIN, INT_MAX, VE, "flags"},
657 {"arnr_max_frames", "altref noise reduction max frame count", offsetof(VP8Context, arnr_max_frames), AV_OPT_TYPE_INT, {.i64 = 0}, 0, 15, VE},
658 {"arnr_strength", "altref noise reduction filter strength", offsetof(VP8Context, arnr_strength), AV_OPT_TYPE_INT, {.i64 = 3}, 0, 6, VE},
659 {"arnr_type", "altref noise reduction filter type", offsetof(VP8Context, arnr_type), AV_OPT_TYPE_INT, {.i64 = 3}, 1, 3, VE},
660 {"rc_lookahead", "Number of frames to look ahead for alternate reference frame selection", offsetof(VP8Context, lag_in_frames), AV_OPT_TYPE_INT, {.i64 = 25}, 0, 25, VE},
661 { "crf", "Select the quality for constant quality mode", offsetof(VP8Context, crf), AV_OPT_TYPE_INT, {.i64 = 0}, 0, 63, VE },
Definition: libavcodec/avcodec.h:271
Definition: pixfmt.h:67
#define CODEC_FLAG_PASS2
Use internal 2pass ratecontrol in second pass mode.
Definition: libavcodec/avcodec.h:705
int64_t av_rescale_rnd(int64_t a, int64_t b, int64_t c, enum AVRounding rnd)
Rescale a 64-bit integer with specified rounding.
Definition: mathematics.c:60
void * av_realloc_f(void *ptr, size_t nelem, size_t elsize)
Allocate or reallocate a block of memory.
Definition: mem.c:168
#define CODEC_FLAG_PASS1
Use internal 2pass ratecontrol in first pass mode.
Definition: libavcodec/avcodec.h:704
Definition: opt.h:222
int rc_initial_buffer_occupancy
Number of bits which should be loaded into the rc buffer before decoding starts.
Definition: libavcodec/avcodec.h:2233
struct FrameListData * coded_frame_list
Definition: libvpxenc.c:66
char * stats_in
pass2 encoding statistics input buffer Concatenated stuff from stats_out of pass1 should be placed he...
Definition: libavcodec/avcodec.h:2367
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining list
Definition: filter_design.txt:23
Definition: opt.h:221
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
#define VP8F_AUTO_ALT_REF
Enable automatic alternate reference frame generation.
Definition: libvpxenc.c:74
#define CODEC_FLAG_PSNR
error[?] variables will be set during encoding.
Definition: libavcodec/avcodec.h:708
const char * class_name
The name of the class; usually it is the same name as the context structure type to which the AVClass...
Definition: log.h:55
Definition: opt.h:229
static av_cold int codecctl_int(AVCodecContext *avctx, enum vp8e_enc_control_id id, int val)
Definition: libvpxenc.c:203
AVOptions.
static void coded_frame_add(void *list, struct FrameListData *cx_frame)
Definition: libvpxenc.c:176
int64_t pts
Presentation timestamp in time_base units (time when frame should be shown to user).
Definition: frame.h:159
int mb_threshold
Macroblock threshold below which the user specified macroblock types will be used.
Definition: libavcodec/avcodec.h:1701
Definition: opt.h:236
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
#define CODEC_CAP_DELAY
Encoder or decoder requires flushing with NULL input at the end in order to give the complete and cor...
Definition: libavcodec/avcodec.h:770
Definition: libavcodec/internal.h:112
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
simple assert() macros that are a bit more flexible than ISO C assert().
char * av_base64_encode(char *out, int out_size, const uint8_t *in, int in_size)
Encode data to base64 and null-terminate.
Definition: libavutil/base64.c:137
external API header
AVFrame * avcodec_alloc_frame(void)
Allocate an AVFrame and set its fields to default values.
Definition: libavcodec/utils.c:951
static av_cold void dump_enc_cfg(AVCodecContext *avctx, const struct vpx_codec_enc_cfg *cfg)
Definition: libvpxenc.c:121
#define AV_BASE64_SIZE(x)
Calculate the output size needed to base64-encode x bytes to a null-terminated string.
Definition: base64.h:61
#define CODEC_CAP_AUTO_THREADS
Codec supports avctx->thread_count == 0 (auto).
Definition: libavcodec/avcodec.h:822
int ticks_per_frame
For some codecs, the time base is closer to the field rate than the frame rate.
Definition: libavcodec/avcodec.h:1262
static av_cold void log_encoder_error(AVCodecContext *avctx, const char *desc)
Definition: libvpxenc.c:110
Definition: libavcodec/avcodec.h:243
int ff_alloc_packet2(AVCodecContext *avctx, AVPacket *avpkt, int size)
Check AVPacket size and/or allocate data.
Definition: libavcodec/utils.c:1377
int thread_count
thread count is used to decide how many independent tasks should be passed to execute() ...
Definition: libavcodec/avcodec.h:2575
#define VP8F_ERROR_RESILIENT
Enable measures appropriate for streaming over lossy links.
Definition: libvpxenc.c:73
static av_cold int vpx_init(AVCodecContext *avctx, const struct vpx_codec_iface *iface)
Definition: libvpxenc.c:236
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
static int storeframe(AVCodecContext *avctx, struct FrameListData *cx_frame, AVPacket *pkt, AVFrame *coded_frame)
Store coded frame information in format suitable for return from encode2().
Definition: libvpxenc.c:450
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
static void cx_pktcpy(struct FrameListData *dst, const struct vpx_codec_cx_pkt *src, VP8Context *ctx)
Definition: libvpxenc.c:416
float rc_buffer_aggressivity
Definition: libavcodec/avcodec.h:2205
static av_cold void free_coded_frame(struct FrameListData *cx_frame)
Definition: libvpxenc.c:186
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
float qcompress
amount of qscale change between easy & hard scenes (0.0-1.0)
Definition: libavcodec/avcodec.h:2134
static int vp8_encode(AVCodecContext *avctx, AVPacket *pkt, const AVFrame *frame, int *got_packet)
Definition: libvpxenc.c:578
int gop_size
the number of pictures in a group of pictures, or 0 for intra_only
Definition: libavcodec/avcodec.h:1321
static const char *const ctlidstr[]
String mappings for enum vp8e_enc_control_id.
Definition: libvpxenc.c:89
planar YUV 4:2:0, 12bpp, (1 Cr & Cb sample per 2x2 Y samples)
Definition: pixfmt.h:68
common internal api header.
Definition: libvpxenc.c:57
common internal and external API header
#define CODEC_CAP_EXPERIMENTAL
Codec is experimental and is thus avoided in favor of non experimental encoders.
Definition: libavcodec/avcodec.h:796
static av_cold void free_frame_list(struct FrameListData *list)
Definition: libvpxenc.c:192
struct VP8EncoderContext VP8Context
int av_base64_decode(uint8_t *out, const char *in_str, int out_size)
Decode a base64-encoded string.
Definition: libavutil/base64.c:78
Definition: vp8.h:136
int64_t dts
Decompression timestamp in AVStream->time_base units; the time at which the packet is decompressed...
Definition: libavcodec/avcodec.h:1050
static int queue_frames(AVCodecContext *avctx, AVPacket *pkt_out, AVFrame *coded_frame)
Queue multiple output frames from the encoder, returning the front-most.
Definition: libvpxenc.c:492
Definition: avutil.h:143
Definition: opt.h:244
int64_t pts
Presentation timestamp in AVStream->time_base units; the time at which the decompressed packet will b...
Definition: libavcodec/avcodec.h:1044
Generated on Wed Jun 18 2025 06:53:03 for FFmpeg by
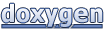