FFmpeg
|
vp8.h
Go to the documentation of this file.
168 int8_t base_quant[4];
190 int16_t luma_qmul[2];
192 int16_t chroma_qmul[2];
Definition: videodsp.h:40
VP5 and VP6 compatible video decoder (common features)
struct VP8Macroblock VP8Macroblock
struct VP8Frame VP8Frame
os2threads to pthreads wrapper
Definition: vp8.h:69
int update_probabilities
If this flag is not set, all the probability updates are discarded after this frame is decoded...
Definition: vp8.h:252
struct VP8FilterStrength VP8FilterStrength
int update_golden
VP56_FRAME_NONE if not updated, or which frame to copy if so.
Definition: vp8.h:245
Definition: vp8.h:67
VP8 compatible video decoder.
the mask is usually to keep the same permissions Filters should remove permissions on reference they give to output whenever necessary It can be automatically done by setting the rej_perms field on the output pad Here are a few guidelines corresponding to common then the filter should push the output frames on the output link immediately As an exception to the previous rule if the input frame is enough to produce several output frames then the filter needs output only at least one per link The additional frames can be left buffered in the filter
Definition: filter_design.txt:201
Definition: os2threads.h:41
int num_coeff_partitions
All coefficients are contained in separate arith coding contexts.
Definition: vp8.h:258
struct VP8ThreadData VP8ThreadData
Multithreading support functions.
VP5 and VP6 compatible video decoder (common data)
Definition: vp8.h:101
Definition: thread.h:35
void(* vp8_mc_func)(uint8_t *dst, ptrdiff_t dstStride, uint8_t *src, ptrdiff_t srcStride, int h, int x, int y)
Definition: vp8dsp.h:33
Definition: vp8.h:80
uint8_t enabled
whether each mb can have a different strength based on mode/ref
Definition: vp8.h:165
struct VP8Context VP8Context
refcounted data buffer API
Definition: vp8.h:86
H.264 / AVC / MPEG4 prediction functions.
Definition: vp8dsp.h:37
Definition: vp56.h:60
Definition: vp8.h:60
Definition: vp8.h:68
w32threads to pthreads wrapper
Definition: vp8.h:136
Generated on Mon Nov 18 2024 06:52:03 for FFmpeg by
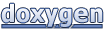