FFmpeg
|
VP5 and VP6 compatible video decoder (common features) More...
#include "vp56data.h"
#include "dsputil.h"
#include "get_bits.h"
#include "hpeldsp.h"
#include "bytestream.h"
#include "h264chroma.h"
#include "videodsp.h"
#include "vp3dsp.h"
#include "vp56dsp.h"
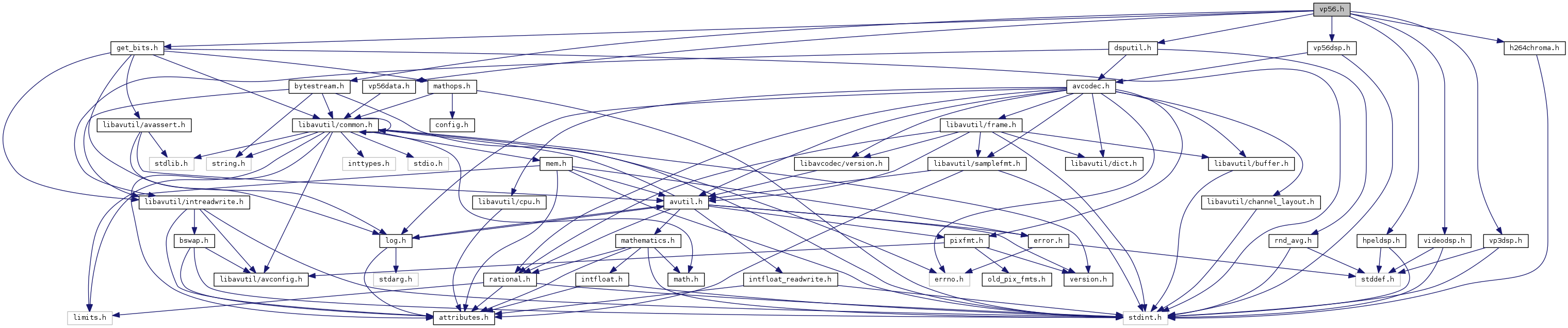
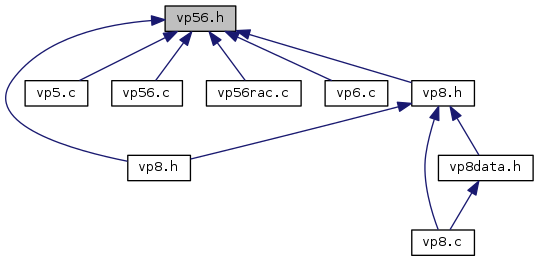
Go to the source code of this file.
Data Structures | |
struct | VP56mv |
struct | VP56RangeCoder |
struct | VP56RefDc |
struct | VP56Macroblock |
struct | VP56Model |
struct | vp56_context |
Macros | |
#define | VP56_SIZE_CHANGE 1 |
#define | vp56_rac_get_prob vp56_rac_get_prob |
Typedefs | |
typedef struct vp56_context | VP56Context |
typedef struct VP56mv | VP56mv |
typedef void(* | VP56ParseVectorAdjustment) (VP56Context *s, VP56mv *vect) |
typedef void(* | VP56Filter) (VP56Context *s, uint8_t *dst, uint8_t *src, int offset1, int offset2, int stride, VP56mv mv, int mask, int select, int luma) |
typedef void(* | VP56ParseCoeff) (VP56Context *s) |
typedef void(* | VP56DefaultModelsInit) (VP56Context *s) |
typedef void(* | VP56ParseVectorModels) (VP56Context *s) |
typedef int(* | VP56ParseCoeffModels) (VP56Context *s) |
typedef int(* | VP56ParseHeader) (VP56Context *s, const uint8_t *buf, int buf_size) |
typedef struct VP56RangeCoder | VP56RangeCoder |
typedef struct VP56RefDc | VP56RefDc |
typedef struct VP56Macroblock | VP56Macroblock |
typedef struct VP56Model | VP56Model |
Variables | |
const uint8_t | ff_vp56_norm_shift [256] |
vp56 specific range coder implementation More... | |
Detailed Description
VP5 and VP6 compatible video decoder (common features)
Definition in file vp56.h.
Macro Definition Documentation
#define vp56_rac_get_prob vp56_rac_get_prob |
Definition at line 226 of file vp56.h.
Referenced by decode_block_coeffs_internal(), decode_mb_mode(), decode_splitmvs(), read_mv_component(), vp56_parse_mb_type(), vp56_parse_mb_type_models(), vp56_rac_get_tree(), vp5_parse_coeff(), vp5_parse_coeff_models(), vp5_parse_vector_adjustment(), vp5_parse_vector_models(), vp6_parse_coeff(), vp6_parse_coeff_models(), vp6_parse_vector_adjustment(), vp6_parse_vector_models(), vp8_rac_get(), vp8_rac_get_coeff(), and vp8_rac_get_tree().
#define VP56_SIZE_CHANGE 1 |
Definition at line 46 of file vp56.h.
Referenced by ff_vp56_decode_frame(), vp5_parse_header(), and vp6_parse_header().
Typedef Documentation
typedef struct vp56_context VP56Context |
typedef void(* VP56DefaultModelsInit) (VP56Context *s) |
typedef struct VP56Macroblock VP56Macroblock |
typedef void(* VP56ParseCoeff) (VP56Context *s) |
typedef int(* VP56ParseCoeffModels) (VP56Context *s) |
typedef int(* VP56ParseHeader) (VP56Context *s, const uint8_t *buf, int buf_size) |
typedef void(* VP56ParseVectorAdjustment) (VP56Context *s, VP56mv *vect) |
typedef void(* VP56ParseVectorModels) (VP56Context *s) |
typedef struct VP56RangeCoder VP56RangeCoder |
Function Documentation
int ff_vp56_decode_frame | ( | AVCodecContext * | avctx, |
void * | data, | ||
int * | got_frame, | ||
AVPacket * | avpkt | ||
) |
int ff_vp56_free | ( | AVCodecContext * | avctx | ) |
Definition at line 737 of file vp56.c.
Referenced by ff_vp56_init_context(), and vp6_decode_free().
int ff_vp56_free_context | ( | VP56Context * | s | ) |
Definition at line 743 of file vp56.c.
Referenced by ff_vp56_free(), and vp6_decode_free().
int ff_vp56_init | ( | AVCodecContext * | avctx, |
int | flip, | ||
int | has_alpha | ||
) |
Definition at line 678 of file vp56.c.
Referenced by vp5_decode_init(), and vp6_decode_init().
int ff_vp56_init_context | ( | AVCodecContext * | avctx, |
VP56Context * | s, | ||
int | flip, | ||
int | has_alpha | ||
) |
Definition at line 684 of file vp56.c.
Referenced by ff_vp56_init(), and vp6_decode_init().
void ff_vp56_init_dequant | ( | VP56Context * | s, |
int | quantizer | ||
) |
Definition at line 34 of file vp56.c.
Referenced by vp5_parse_header(), and vp6_parse_header().
void ff_vp56_init_range_decoder | ( | VP56RangeCoder * | c, |
const uint8_t * | buf, | ||
int | buf_size | ||
) |
Definition at line 40 of file vp56rac.c.
Referenced by decode_frame_header(), setup_partitions(), vp5_parse_header(), and vp6_parse_header().
|
static |
Definition at line 261 of file vp56.h.
Referenced by vp56_parse_mb_type_models(), vp56_rac_gets(), vp5_parse_coeff(), vp5_parse_header(), vp6_parse_coeff(), vp6_parse_coeff_models(), and vp6_parse_header().
|
static |
|
static |
Definition at line 243 of file vp56.h.
Referenced by decode_block_coeffs(), decode_block_coeffs_internal(), decode_frame_header(), decode_mb_mode(), decode_mvs(), decode_splitmvs(), and read_mv_component().
|
static |
Definition at line 337 of file vp56.h.
Referenced by vp56_parse_mb_type(), vp56_parse_mb_type_models(), vp5_parse_coeff(), vp5_parse_vector_adjustment(), vp6_parse_coeff(), and vp6_parse_vector_adjustment().
|
static |
Definition at line 285 of file vp56.h.
Referenced by vp56_decode_4mv(), vp56_parse_mb_type_models(), vp56_rac_gets_nn(), vp5_parse_header(), vp6_parse_coeff_models(), and vp6_parse_header().
|
static |
Definition at line 324 of file vp56.h.
Referenced by vp5_parse_coeff_models(), vp5_parse_vector_models(), vp6_parse_coeff_models(), and vp6_parse_vector_models().
|
static |
Definition at line 202 of file vp56.h.
Referenced by vp56_rac_get(), vp56_rac_get_prob(), and vp56_rac_get_prob_branchy().
|
static |
Definition at line 280 of file vp56.h.
Referenced by decode_block_coeffs_internal(), decode_frame_header(), parse_segment_info(), update_lf_deltas(), update_refs(), vp8_rac_get_sint(), and vp8_rac_get_uint().
|
static |
Definition at line 365 of file vp56.h.
Referenced by decode_block_coeffs_internal().
|
static |
Definition at line 330 of file vp56.h.
Referenced by decode_frame_header().
|
static |
Definition at line 308 of file vp56.h.
Referenced by get_quants(), and parse_segment_info().
|
static |
Definition at line 352 of file vp56.h.
Referenced by decode_intra4x4_modes(), and decode_mb_mode().
|
static |
Definition at line 296 of file vp56.h.
Referenced by decode_frame_header(), get_quants(), parse_segment_info(), ref_to_update(), setup_partitions(), update_lf_deltas(), vp8_rac_get_nn(), and vp8_rac_get_sint().
Variable Documentation
const uint8_t ff_vp56_norm_shift[256] |
vp56 specific range coder implementation
Definition at line 25 of file vp56rac.c.
Referenced by vp56_rac_renorm().
Generated on Mon Nov 18 2024 06:52:10 for FFmpeg by
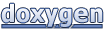