FFmpeg
|
vp56.c
Go to the documentation of this file.
120 model->mb_type[ctx][type][0] = 255 - (255 * model->mb_types_stats[ctx][type][0]) / (1 + model->mb_types_stats[ctx][type][0] + model->mb_types_stats[ctx][type][1]);
Definition: vp56data.h:32
void(* edge_filter_hor)(uint8_t *yuv, int stride, int t)
Definition: vp56dsp.h:28
VP5 and VP6 compatible video decoder (common features)
static int vp56_get_vectors_predictors(VP56Context *s, int row, int col, VP56Frame ref_frame)
Definition: vp56.c:41
int coded_width
Bitstream width / height, may be different from width/height e.g.
Definition: libavcodec/avcodec.h:1312
void(* put_no_rnd_pixels_l2)(uint8_t *dst, const uint8_t *a, const uint8_t *b, ptrdiff_t stride, int h)
Copy 8xH pixels from source to destination buffer using a bilinear filter with no rounding (i...
Definition: vp3dsp.h:36
void avcodec_set_dimensions(AVCodecContext *s, int width, int height)
Definition: libavcodec/utils.c:177
static VP56mb vp56_parse_mb_type(VP56Context *s, VP56mb prev_type, int ctx)
Definition: vp56.c:150
void * av_realloc(void *ptr, size_t size)
Allocate or reallocate a block of memory.
Definition: mem.c:141
void ff_vp56dsp_init(VP56DSPContext *s, enum AVCodecID codec)
Definition: vp56dsp.c:78
av_cold int ff_vp56_init(AVCodecContext *avctx, int flip, int has_alpha)
Definition: vp56.c:678
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
Definition: vp56data.h:35
void(* idct_add)(uint8_t *dest, int line_size, int16_t *block)
Definition: vp3dsp.h:42
planar YUV 4:2:0, 20bpp, (1 Cr & Cb sample per 2x2 Y & A samples)
Definition: pixfmt.h:105
Definition: vp56data.h:33
av_cold int ff_vp56_init_context(AVCodecContext *avctx, VP56Context *s, int flip, int has_alpha)
Definition: vp56.c:684
static av_always_inline int vp56_rac_get_tree(VP56RangeCoder *c, const VP56Tree *tree, const uint8_t *probs)
Definition: vp56.h:337
void ff_h264chroma_init(H264ChromaContext *c, int bit_depth)
Definition: h264chroma.c:38
VP56ParseVectorAdjustment parse_vector_adjustment
Definition: vp56.h:161
static const uint8_t vp56_pre_def_mb_type_stats[16][3][10][2]
Definition: vp56data.h:101
Definition: vp56.h:97
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
static void vp56_mc(VP56Context *s, int b, int plane, uint8_t *src, int stride, int x, int y)
Definition: vp56.c:313
external API header
void(* edge_filter_ver)(uint8_t *yuv, int stride, int t)
Definition: vp56dsp.h:29
void(* idct_put)(uint8_t *dest, int line_size, int16_t *block)
Definition: vp3dsp.h:41
VP5 and VP6 compatible video decoder (common data)
struct VP56mv VP56mv
int ff_vp56_decode_frame(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: vp56.c:496
Definition: libavcodec/avcodec.h:193
int(* execute2)(struct AVCodecContext *c, int(*func)(struct AVCodecContext *c2, void *arg, int jobnr, int threadnr), void *arg2, int *ret, int count)
The codec may call this to execute several independent things.
Definition: libavcodec/avcodec.h:2635
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
FIXME Range Coding of cr are mx and my are Motion Vector top and top right vectors is used as motion vector prediction the used motion vector is the sum of the predictor and(mvx_diff, mvy_diff)*mv_scale Intra DC Predicton block[y][x] dc[1]
Definition: snow.txt:392
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
op_pixels_func put_pixels_tab[4][4]
Halfpel motion compensation with rounding (a+b+1)>>1.
Definition: hpeldsp.h:56
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
static int ff_vp56_decode_mbs(AVCodecContext *avctx, void *, int, int)
Definition: vp56.c:577
Inter MB, above/left vector + delta, from golden frame.
Definition: vp56data.h:46
static void vp56_decode_mb(VP56Context *s, int row, int col, int is_alpha)
Definition: vp56.c:383
void av_frame_unref(AVFrame *frame)
Unreference all the buffers referenced by frame and reset the frame fields.
Definition: frame.c:330
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
#define type
AVFrame * av_frame_alloc(void)
Allocate an AVFrame and set its fields to default values.
Definition: frame.c:95
int av_frame_ref(AVFrame *dst, AVFrame *src)
Setup a new reference to the data described by an given frame.
Definition: frame.c:228
planar YUV 4:2:0, 12bpp, (1 Cr & Cb sample per 2x2 Y samples)
Definition: pixfmt.h:68
common internal api header.
static int ref_frame(Vp3DecodeContext *s, ThreadFrame *dst, ThreadFrame *src)
Definition: vp3.c:1877
void av_frame_free(AVFrame **frame)
Free the frame and any dynamically allocated objects in it, e.g.
Definition: frame.c:108
Definition: vp56.h:60
enum AVDiscard skip_loop_filter
Skip loop filtering for selected frames.
Definition: libavcodec/avcodec.h:2735
#define T(x)
static void vp56_add_predictors_dc(VP56Context *s, VP56Frame ref_frame)
Definition: vp56.c:264
void(* emulated_edge_mc)(uint8_t *buf, const uint8_t *src, ptrdiff_t linesize, int block_w, int block_h, int src_x, int src_y, int w, int h)
Copy a rectangular area of samples to a temporary buffer and replicate the border samples...
Definition: videodsp.h:58
Inter MB, above/left vector + delta, from previous frame.
Definition: vp56data.h:42
static void vp56_deblock_filter(VP56Context *s, uint8_t *yuv, int stride, int dx, int dy)
Definition: vp56.c:305
static const int8_t vp56_candidate_predictor_pos[12][2]
Definition: vp56data.h:237
#define AV_GET_BUFFER_FLAG_REF
The decoder will keep a reference to the frame and may reuse it later.
Definition: libavcodec/avcodec.h:909
Definition: vp56data.h:34
Generated on Fri Aug 1 2025 06:53:49 for FFmpeg by
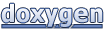