FFmpeg
|
seek.c
Go to the documentation of this file.
35 int64_t pos_lo; ///< position of the frame with low timestamp in file or INT64_MAX if not found (yet)
38 int64_t pos_hi; ///< position of the frame with high timestamp in file or INT64_MAX if not found (yet)
AVParserState * ff_store_parser_state(AVFormatContext *s)
Store current parser state and file position.
Definition: seek.c:394
memory handling functions
int64_t avio_seek(AVIOContext *s, int64_t offset, int whence)
fseek() equivalent for AVIOContext.
Definition: aviobuf.c:199
static int sync(AVFormatContext *s, uint8_t *header)
Read input until we find the next ident.
Definition: lxfdec.c:85
static void search_hi_lo_keyframes(AVFormatContext *s, int64_t timestamp, AVRational timebase, int flags, AVSyncPoint *sync, int keyframes_to_find, int *found_lo, int *found_hi, int first_iter)
Partial search for keyframes in multiple streams.
Definition: seek.c:97
struct AVPacketList * packet_buffer
This buffer is only needed when packets were already buffered but not decoded, for example to get the...
Definition: avformat.h:1238
int64_t pos_lo
position of the frame with low timestamp in file or INT64_MAX if not found (yet)
Definition: seek.c:35
void ff_read_frame_flush(AVFormatContext *s)
Flush the frame reader.
Definition: libavformat/utils.c:1663
Definition: avformat.h:1279
static av_always_inline int64_t avio_tell(AVIOContext *s)
ftell() equivalent for AVIOContext.
Definition: avio.h:248
struct AVPacketList * raw_packet_buffer
Raw packets from the demuxer, prior to parsing and decoding.
Definition: avformat.h:1250
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
void ff_restore_parser_state(AVFormatContext *s, AVParserState *state)
Restore previously saved parser state and file position.
Definition: seek.c:444
int av_compare_ts(int64_t ts_a, AVRational tb_a, int64_t ts_b, AVRational tb_b)
Compare 2 timestamps each in its own timebases.
Definition: mathematics.c:135
int64_t first_ts
first packet timestamp in this iteration (to fill term_ts later)
Definition: seek.c:45
int raw_packet_buffer_remaining_size
Definition: avformat.h:1261
#define RAW_PACKET_BUFFER_SIZE
Remaining size available for raw_packet_buffer, in bytes.
Definition: avformat.h:1260
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
int av_read_frame(AVFormatContext *s, AVPacket *pkt)
Return the next frame of a stream.
Definition: libavformat/utils.c:1530
struct AVPacketList * parse_queue
Packets split by the parser get queued here.
Definition: avformat.h:1255
int64_t ff_gen_syncpoint_search(AVFormatContext *s, int stream_index, int64_t pos, int64_t ts_min, int64_t ts, int64_t ts_max, int flags)
Search for the sync point of all active streams.
Definition: seek.c:244
void ff_free_parser_state(AVFormatContext *s, AVParserState *state)
Free previously saved parser state.
Definition: seek.c:489
static int64_t ts_distance(int64_t ts_hi, AVRational tb_hi, int64_t ts_lo, AVRational tb_lo)
Compute a distance between timestamps.
Definition: seek.c:63
int64_t dts
Decompression timestamp in AVStream->time_base units; the time at which the packet is decompressed...
Definition: libavcodec/avcodec.h:1050
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: avformat.h:679
enum AVDiscard discard
Selects which packets can be discarded at will and do not need to be demuxed.
Definition: avformat.h:702
int64_t pts
Presentation timestamp in AVStream->time_base units; the time at which the decompressed packet will b...
Definition: libavcodec/avcodec.h:1044
trying all byte sequences megabyte in length and selecting the best looking sequence will yield cases to try But a word about which is also called distortion Distortion can be quantified by almost any quality measurement one chooses the sum of squared differences is used but more complex methods that consider psychovisual effects can be used as well It makes no difference in this discussion First step
Definition: rate_distortion.txt:12
int64_t pos_hi
position of the frame with high timestamp in file or INT64_MAX if not found (yet) ...
Definition: seek.c:38
Generated on Wed May 8 2024 06:52:11 for FFmpeg by
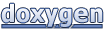