FFmpeg
|
matroskaenc.c
Go to the documentation of this file.
229 static ebml_master start_ebml_master(AVIOContext *pb, unsigned int elementid, uint64_t expectedsize)
266 static mkv_seekhead * mkv_start_seekhead(AVIOContext *pb, int64_t segment_offset, int numelements)
286 static int mkv_add_seekhead_entry(mkv_seekhead *seekhead, unsigned int elementid, uint64_t filepos)
387 static int64_t mkv_write_cues(AVIOContext *pb, mkv_cues *cues, mkv_track *tracks, int num_tracks)
455 static void get_aac_sample_rates(AVFormatContext *s, AVCodecContext *codec, int *sample_rate, int *output_sample_rate)
469 static int mkv_write_codecprivate(AVFormatContext *s, AVIOContext *pb, AVCodecContext *codec, int native_id, int qt_id)
612 if(st->avg_frame_rate.num && st->avg_frame_rate.den && 1.0/av_q2d(st->avg_frame_rate) > av_q2d(codec->time_base))
659 int64_t d_width = av_rescale(codec->width, st->sample_aspect_ratio.num, st->sample_aspect_ratio.den);
1248 ebml_master blockgroup = start_ebml_master(pb, MATROSKA_ID_BLOCKGROUP, mkv_blockgroup_size(pkt->size));
void av_sha_final(AVSHA *ctx, uint8_t *digest)
Finish hashing and output digest value.
Definition: sha.c:320
Definition: libavcodec/avcodec.h:312
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
Definition: libavcodec/avcodec.h:271
Definition: libavcodec/avcodec.h:410
static void put_ebml_size_unknown(AVIOContext *pb, int bytes)
Write an EBML size meaning "unknown size".
Definition: matroskaenc.c:130
Definition: libavcodec/avcodec.h:100
void ff_metadata_conv_ctx(AVFormatContext *ctx, const AVMetadataConv *d_conv, const AVMetadataConv *s_conv)
Definition: libavformat/metadata.c:59
void av_sha_update(AVSHA *ctx, const uint8_t *data, unsigned int len)
Update hash value.
Definition: sha.c:293
Definition: dict.h:80
int avio_close_dyn_buf(AVIOContext *s, uint8_t **pbuffer)
Return the written size and a pointer to the buffer.
Definition: aviobuf.c:988
Definition: matroska.h:226
static void mkv_write_simpletag(AVIOContext *pb, AVDictionaryEntry *t)
Definition: matroskaenc.c:759
Definition: matroskaenc.c:67
void avpriv_set_pts_info(AVStream *s, int pts_wrap_bits, unsigned int pts_num, unsigned int pts_den)
Set the time base and wrapping info for a given stream.
Definition: libavformat/utils.c:3952
static int write_packet(AVFormatContext *s, AVPacket *pkt)
Definition: libavformat/assenc.c:63
static int mkv_write_attachments(AVFormatContext *s)
Definition: matroskaenc.c:855
int64_t avio_seek(AVIOContext *s, int64_t offset, int whence)
fseek() equivalent for AVIOContext.
Definition: aviobuf.c:199
#define MATROSKA_ID_TAGTARGETS_CHAPTERUID
Definition: matroska.h:173
Definition: libavcodec/avcodec.h:393
static av_always_inline uint64_t av_double2int(double f)
Reinterpret a double as a 64-bit integer.
Definition: intfloat.h:70
Definition: libavcodec/avcodec.h:115
unsigned int ff_codec_get_tag(const AVCodecTag *tags, enum AVCodecID id)
Definition: libavformat/utils.c:2604
void * av_realloc(void *ptr, size_t size)
Allocate or reallocate a block of memory.
Definition: mem.c:141
Definition: libavcodec/avcodec.h:251
Definition: matroskaenc.c:47
FFmpeg currently uses a custom build this text attempts to document some of its obscure features and options Makefile the full command issued by make and its output will be shown on the screen DESTDIR Destination directory for the install targets
Definition: build_system.txt:1
AVDictionaryEntry * av_dict_get(AVDictionary *m, const char *key, const AVDictionaryEntry *prev, int flags)
Get a dictionary entry with matching key.
Definition: dict.c:39
Definition: libavcodec/avcodec.h:130
Definition: libavcodec/avcodec.h:397
#define AVFMT_TS_NONSTRICT
Format does not require strictly increasing timestamps, but they must still be monotonic.
Definition: avformat.h:363
int64_t pos
absolute offset in the file where the master's elements start
Definition: matroskaenc.c:43
Definition: libavcodec/avcodec.h:406
Definition: libavcodec/avcodec.h:401
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
Definition: matroskaenc.c:81
Public dictionary API.
static int mkv_write_tag(AVFormatContext *s, AVDictionary *m, unsigned int elementid, unsigned int uid, ebml_master *tags)
Definition: matroskaenc.c:789
Definition: libavcodec/avcodec.h:412
Definition: libavcodec/avcodec.h:383
Definition: libavcodec/avcodec.h:371
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
static int mkv_write_srt_blocks(AVFormatContext *s, AVIOContext *pb, AVPacket *pkt)
Definition: matroskaenc.c:1176
Definition: libavformat/internal.h:35
static void get_aac_sample_rates(AVFormatContext *s, AVCodecContext *codec, int *sample_rate, int *output_sample_rate)
Definition: matroskaenc.c:455
struct MatroskaMuxContext MatroskaMuxContext
#define CODEC_FLAG_BITEXACT
Use only bitexact stuff (except (I)DCT).
Definition: libavcodec/avcodec.h:715
static av_always_inline int64_t avio_tell(AVIOContext *s)
ftell() equivalent for AVIOContext.
Definition: avio.h:248
int bits_per_coded_sample
bits per sample/pixel from the demuxer (needed for huffyuv).
Definition: libavcodec/avcodec.h:2546
void avio_write(AVIOContext *s, const unsigned char *buf, int size)
Definition: aviobuf.c:173
int duration
Duration of this packet in AVStream->time_base units, 0 if unknown.
Definition: libavcodec/avcodec.h:1073
static void put_ebml_num(AVIOContext *pb, uint64_t num, int bytes)
Write a number in EBML variable length format.
Definition: matroskaenc.c:154
Definition: libavcodec/avcodec.h:400
static int mkv_add_seekhead_entry(mkv_seekhead *seekhead, unsigned int elementid, uint64_t filepos)
Definition: matroskaenc.c:286
int64_t av_rescale_q(int64_t a, AVRational bq, AVRational cq)
Rescale a 64-bit integer by 2 rational numbers.
Definition: mathematics.c:130
Definition: libavcodec/avcodec.h:426
Definition: libavcodec/avcodec.h:126
Definition: dict.c:28
int av_get_bits_per_sample(enum AVCodecID codec_id)
Return codec bits per sample.
Definition: libavcodec/utils.c:2690
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
struct mkv_seekhead mkv_seekhead
#define MAX_CUETRACKPOS_SIZE
per-cuepoint-track - 3 1-byte EBML IDs, 3 1-byte EBML sizes, 2 8-byte uint max
Definition: matroskaenc.c:107
const char * av_convert_lang_to(const char *lang, enum AVLangCodespace target_codespace)
Convert a language code to a target codespace.
Definition: avlanguage.c:736
static int put_xiph_codecpriv(AVFormatContext *s, AVIOContext *pb, AVCodecContext *codec)
Definition: matroskaenc.c:427
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
int64_t convergence_duration
Time difference in AVStream->time_base units from the pts of this packet to the point at which the ou...
Definition: libavcodec/avcodec.h:1099
AVBufferRef * buf
A reference to the reference-counted buffer where the packet data is stored.
Definition: libavcodec/avcodec.h:1034
static int ebml_num_size(uint64_t num)
Calculate how many bytes are needed to represent a given number in EBML.
Definition: matroskaenc.c:141
Definition: avutil.h:144
enum AVMediaType avcodec_get_type(enum AVCodecID codec_id)
Get the type of the given codec.
Definition: libavcodec/utils.c:3082
static mkv_seekhead * mkv_start_seekhead(AVIOContext *pb, int64_t segment_offset, int numelements)
Initialize a mkv_seekhead element to be ready to index level 1 Matroska elements. ...
Definition: matroskaenc.c:266
int ff_avc_parse_nal_units_buf(const uint8_t *buf_in, uint8_t **buf, int *size)
Definition: avc.c:92
Definition: mpeg4audio.h:29
Definition: libavcodec/avcodec.h:464
static void mkv_write_block(AVFormatContext *s, AVIOContext *pb, unsigned int blockid, AVPacket *pkt, int flags)
Definition: matroskaenc.c:1122
#define AV_DISPOSITION_FORCED
Track should be used during playback by default.
Definition: avformat.h:617
int seekable
A combination of AVIO_SEEKABLE_ flags or 0 when the stream is not seekable.
Definition: avio.h:117
int void avio_flush(AVIOContext *s)
Force flushing of buffered data to the output s.
Definition: aviobuf.c:193
Definition: avlanguage.h:28
int64_t av_rescale(int64_t a, int64_t b, int64_t c)
Rescale a 64-bit integer with rounding to nearest.
Definition: mathematics.c:118
int av_strcasecmp(const char *a, const char *b)
Locale-independent case-insensitive compare.
Definition: avstring.c:212
const char *const ff_matroska_video_stereo_mode[MATROSKA_VIDEO_STEREO_MODE_COUNT]
Definition: matroska.c:120
int64_t ff_iso8601_to_unix_time(const char *datestr)
Convert a date string in ISO8601 format to Unix timestamp.
Definition: libavformat/utils.c:4170
Definition: libavcodec/avcodec.h:146
internal header for RIFF based (de)muxers do NOT include this in end user applications ...
Definition: libavcodec/avcodec.h:108
Definition: libavcodec/avcodec.h:109
Definition: libavcodec/avcodec.h:449
Definition: matroska.h:230
Definition: libavcodec/avcodec.h:243
static int mkv_add_cuepoint(mkv_cues *cues, int stream, int64_t ts, int64_t cluster_pos)
Definition: matroskaenc.c:368
Definition: matroskaenc.c:52
Definition: libavcodec/avcodec.h:300
Definition: libavcodec/avcodec.h:125
const char * avcodec_get_name(enum AVCodecID id)
Get the name of a codec.
Definition: libavcodec/utils.c:2416
int av_get_bytes_per_sample(enum AVSampleFormat sample_fmt)
Return number of bytes per sample.
Definition: samplefmt.c:104
static void put_ebml_float(AVIOContext *pb, unsigned int elementid, double val)
Definition: matroskaenc.c:185
static void put_ebml_string(AVIOContext *pb, unsigned int elementid, const char *str)
Definition: matroskaenc.c:200
Definition: libavcodec/avcodec.h:133
static int64_t mkv_write_cues(AVIOContext *pb, mkv_cues *cues, mkv_track *tracks, int num_tracks)
Definition: matroskaenc.c:387
struct mkv_seekhead_entry mkv_seekhead_entry
Definition: libavcodec/avcodec.h:384
unsigned int codec_tag
fourcc (LSB first, so "ABCD" -> ('D'<<24) + ('C'<<16) + ('B'<<8) + 'A').
Definition: libavcodec/avcodec.h:1160
Definition: libavcodec/avcodec.h:171
struct ebml_master ebml_master
static unsigned int av_lfg_get(AVLFG *c)
Get the next random unsigned 32-bit number using an ALFG.
Definition: lfg.h:38
Definition: libavcodec/avcodec.h:422
Definition: libavcodec/avcodec.h:403
#define MAX_CUEPOINT_SIZE(num_tracks)
per-cuepoint - 2 1-byte EBML IDs, 2 1-byte EBML sizes, 8-byte uint max
Definition: matroskaenc.c:110
static void put_ebml_uint(AVIOContext *pb, unsigned int elementid, uint64_t val)
Definition: matroskaenc.c:173
static int64_t mkv_write_seekhead(AVIOContext *pb, mkv_seekhead *seekhead)
Write the seek head to the file and free it.
Definition: matroskaenc.c:314
Definition: matroska.h:227
Definition: libavcodec/avcodec.h:172
int avpriv_split_xiph_headers(uint8_t *extradata, int extradata_size, int first_header_size, uint8_t *header_start[3], int header_len[3])
Split a single extradata buffer into the three headers that most Xiph codecs use. ...
Definition: xiph.c:24
static int mkv_write_packet_internal(AVFormatContext *s, AVPacket *pkt)
Definition: matroskaenc.c:1207
Definition: libavcodec/avcodec.h:372
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
Definition: matroskaenc.c:73
#define type
int ff_flac_write_header(AVIOContext *pb, AVCodecContext *codec, int last_block)
Definition: flacenc_header.c:27
void ff_put_bmp_header(AVIOContext *pb, AVCodecContext *enc, const AVCodecTag *tags, int for_asf)
Definition: matroskaenc.c:42
Definition: libavcodec/avcodec.h:460
Main libavformat public API header.
Definition: avformat.h:918
static void put_ebml_binary(AVIOContext *pb, unsigned int elementid, const void *buf, int size)
Definition: matroskaenc.c:192
static int mkv_write_codecprivate(AVFormatContext *s, AVIOContext *pb, AVCodecContext *codec, int native_id, int qt_id)
Definition: matroskaenc.c:469
AVBufferRef * av_buffer_ref(AVBufferRef *buf)
Create a new reference to an AVBuffer.
Definition: libavutil/buffer.c:91
int ff_put_wav_header(AVIOContext *pb, AVCodecContext *enc)
static void put_ebml_void(AVIOContext *pb, uint64_t size)
Write a void element of a given size.
Definition: matroskaenc.c:211
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
AVRational time_base
time base in which the start/end timestamps are specified
Definition: avformat.h:920
Definition: avformat.h:377
void av_init_packet(AVPacket *pkt)
Initialize optional fields of a packet with default values.
Definition: avpacket.c:56
int avpriv_mpeg4audio_get_config(MPEG4AudioConfig *c, const uint8_t *buf, int bit_size, int sync_extension)
Parse MPEG-4 systems extradata to retrieve audio configuration.
Definition: mpeg4audio.c:81
int64_t dts
Decompression timestamp in AVStream->time_base units; the time at which the packet is decompressed...
Definition: libavcodec/avcodec.h:1050
Definition: avutil.h:143
uint32_t av_get_random_seed(void)
Get a seed to use in conjunction with random functions.
Definition: random_seed.c:105
Definition: matroskaenc.c:61
Definition: libavcodec/avcodec.h:116
static int mkv_write_packet(AVFormatContext *s, AVPacket *pkt)
Definition: matroskaenc.c:1267
#define MAX_SEEKENTRY_SIZE
2 bytes * 3 for EBML IDs, 3 1-byte EBML lengths, 8 bytes for 64 bit offset, 4 bytes for target EBML I...
Definition: matroskaenc.c:103
static void end_ebml_master(AVIOContext *pb, ebml_master master)
Definition: matroskaenc.c:237
Definition: avutil.h:146
Definition: libavcodec/avcodec.h:423
static ebml_master start_ebml_master(AVIOContext *pb, unsigned int elementid, uint64_t expectedsize)
Definition: matroskaenc.c:229
static int mkv_query_codec(enum AVCodecID codec_id, int std_compliance)
Definition: matroskaenc.c:1362
int64_t pts
Presentation timestamp in AVStream->time_base units; the time at which the decompressed packet will b...
Definition: libavcodec/avcodec.h:1044
Definition: libavcodec/avcodec.h:386
int ff_isom_write_avcc(AVIOContext *pb, const uint8_t *data, int len)
Definition: avc.c:106
Definition: libavcodec/avcodec.h:308
Generated on Mon Aug 4 2025 06:54:22 for FFmpeg by
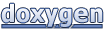