FFmpeg
|
libavformat/rawdec.c
Go to the documentation of this file.
Definition: libavcodec/avcodec.h:410
#define AVFMT_NOBINSEARCH
Format does not allow to fallback to binary search via read_timestamp.
Definition: avformat.h:358
void av_shrink_packet(AVPacket *pkt, int size)
Reduce packet size, correctly zeroing padding.
Definition: avpacket.c:97
void avpriv_set_pts_info(AVStream *s, int pts_wrap_bits, unsigned int pts_num, unsigned int pts_den)
Set the time base and wrapping info for a given stream.
Definition: libavformat/utils.c:3952
#define FF_DEF_RAWVIDEO_DEMUXER(shortname, longname, probe, ext, id)
Definition: rawdec.h:52
Definition: libavcodec/avcodec.h:173
Definition: avformat.h:456
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
AVOptions.
AVStream * avformat_new_stream(AVFormatContext *s, const AVCodec *c)
Add a new stream to a media file.
Definition: libavformat/utils.c:3362
static av_always_inline int64_t avio_tell(AVIOContext *s)
ftell() equivalent for AVIOContext.
Definition: avio.h:248
Definition: libavcodec/avcodec.h:110
full parsing and repack with timestamp and position generation by parser for raw this assumes that ea...
Definition: avformat.h:586
Definition: libavcodec/avcodec.h:426
Definition: rawdec.h:29
int av_new_packet(AVPacket *pkt, int size)
Allocate the payload of a packet and initialize its fields with default values.
Definition: avpacket.c:73
int ff_raw_read_partial_packet(AVFormatContext *s, AVPacket *pkt)
Definition: libavformat/rawdec.c:34
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
int ffio_read_partial(AVIOContext *s, unsigned char *buf, int size)
Read size bytes from AVIOContext into buf.
Definition: aviobuf.c:526
int ff_raw_audio_read_header(AVFormatContext *s)
Definition: libavformat/rawdec.c:54
#define AVFMT_NOGENSEARCH
Format does not allow to fallback to generic search.
Definition: avformat.h:359
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
misc parsing utilities
Definition: libavcodec/avcodec.h:431
static av_always_inline AVRational av_inv_q(AVRational q)
Invert a rational.
Definition: rational.h:122
Definition: libavcodec/avcodec.h:396
Main libavformat public API header.
int64_t start_time
Decoding: pts of the first frame of the stream in presentation order, in stream time base...
Definition: avformat.h:689
struct AVInputFormat * iformat
Can only be iformat or oformat, not both at the same time.
Definition: avformat.h:957
AVRational framerate
AVRational describing framerate, set by a private option.
Definition: rawdec.h:33
Definition: avutil.h:143
int ff_raw_video_read_header(AVFormatContext *s)
Definition: libavformat/rawdec.c:69
Generated on Wed May 28 2025 06:53:11 for FFmpeg by
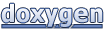