FFmpeg
|
flashsv2enc.c
Go to the documentation of this file.
39 * Make better heuristics for deciding stream parameters (optimum_* functions). Currently these return constants.
40 * Figure out how to encode palette information in the stream, choose an optimum palette at each keyframe.
64 // Disables experimental "smart" parameter-choosing code, as well as the statistics that it depends on.
65 // At the moment, the "smart" code is a great example of how the parameters *shouldn't* be chosen.
649 data = s->current_frame + s->image_width * 3 * s->block_height * row + s->block_width * col * 3;
650 res = encode_block(s, &s->palette, b, prev, data, s->image_width * 3, s->comp, s->dist, keyframe);
740 double save = (1-pow(s->diff_lines/s->diff_blocks/s->block_height, 0.5)) * s->comp_size/s->tot_blocks;
752 double save = (1-pow(s->diff_lines/s->diff_blocks/s->block_height, 0.5)) * s->comp_size/s->tot_blocks;
766 double ideal = ((double)(s->avctx->bit_rate * s->avctx->time_base.den * s->avctx->ticks_per_frame)) /
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
static int compare_sl(FlashSV2Context *s, Block *b, const uint8_t *src, uint8_t *frame, uint8_t *key, int y, int keyframe)
Definition: flashsv2enc.c:589
static void recommend_keyframe(FlashSV2Context *s, int *keyframe)
Definition: flashsv2enc.c:715
Definition: pixfmt.h:67
Definition: put_bits.h:41
Definition: flashsv2enc.c:81
misc image utilities
static int encode_zlibprime(Block *b, Block *prime, uint8_t *buf, int *buf_size, int comp)
Definition: flashsv2enc.c:359
static const unsigned int default_screen_video_v2_palette[128]
Definition: flashsv2enc.c:485
static int generate_optimum_palette(Palette *palette, const uint8_t *image, int width, int height, int stride)
Definition: flashsv2enc.c:518
void * av_realloc(void *ptr, size_t size)
Allocate or reallocate a block of memory.
Definition: mem.c:141
Definition: libavcodec/avcodec.h:234
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
static av_cold int flashsv2_encode_end(AVCodecContext *avctx)
Definition: flashsv2enc.c:912
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
void av_fast_malloc(void *ptr, unsigned int *size, size_t min_size)
Allocate a buffer, reusing the given one if large enough.
Definition: libavcodec/utils.c:96
static int generate_default_palette(Palette *palette)
Definition: flashsv2enc.c:510
struct Block Block
static int encode_block(FlashSV2Context *s, Palette *palette, Block *b, Block *prev, const uint8_t *src, int stride, int comp, int dist, int keyframe)
Definition: flashsv2enc.c:552
static unsigned int chroma_diff(unsigned int c1, unsigned int c2)
Definition: flashsv2enc.c:414
static av_cold int flashsv2_encode_init(AVCodecContext *avctx)
Definition: flashsv2enc.c:178
struct FlashSV2Context FlashSV2Context
static int encode_zlib(Block *b, uint8_t *buf, unsigned long *buf_size, int comp)
Definition: flashsv2enc.c:353
static int encode_15_7(Palette *palette, Block *b, const uint8_t *src, int stride, int dist)
Definition: flashsv2enc.c:535
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
static void put_bits(J2kEncoderContext *s, int val, int n)
put n times val bit
Definition: j2kenc.c:160
Definition: flashsv2enc.c:86
external API header
int av_image_check_size(unsigned int w, unsigned int h, int log_offset, void *log_ctx)
Check if the given dimension of an image is valid, meaning that all bytes of the image can be address...
Definition: imgutils.c:231
#define FF_MIN_BUFFER_SIZE
minimum encoding buffer size Used to avoid some checks during header writing.
Definition: libavcodec/avcodec.h:568
static int pixel_color7_slow(Palette *palette, unsigned color)
Definition: flashsv2enc.c:429
static int write_block(Block *b, uint8_t *buf, int buf_size)
Definition: flashsv2enc.c:313
int ticks_per_frame
For some codecs, the time base is closer to the field rate than the frame rate.
Definition: libavcodec/avcodec.h:1262
static int encode_bgr(Block *b, const uint8_t *src, int stride)
Definition: flashsv2enc.c:393
static int reconfigure_at_keyframe(FlashSV2Context *s, const uint8_t *image, int stride)
Definition: flashsv2enc.c:795
int ff_alloc_packet2(AVCodecContext *avctx, AVPacket *avpkt, int size)
Check AVPacket size and/or allocate data.
Definition: libavcodec/utils.c:1377
static int write_palette(FlashSV2Context *s, uint8_t *buf, int buf_size)
Definition: flashsv2enc.c:277
static int flashsv2_encode_frame(AVCodecContext *avctx, AVPacket *pkt, const AVFrame *pict, int *got_packet)
Definition: flashsv2enc.c:851
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
static int write_header(FlashSV2Context *s, uint8_t *buf, int buf_size)
Definition: flashsv2enc.c:283
static int encode_15_7_sl(Palette *palette, uint8_t *dest, const uint8_t *src, int width, int dist)
Definition: flashsv2enc.c:525
static int mark_all_blocks(FlashSV2Context *s, const uint8_t *src, int stride, int keyframe)
Definition: flashsv2enc.c:607
static int pixel_color7_fast(Palette *palette, unsigned c15)
Definition: flashsv2enc.c:424
Definition: flashsv2enc.c:68
static void init_blocks(FlashSV2Context *s, Block *blocks, uint8_t *encbuf, uint8_t *databuf)
Definition: flashsv2enc.c:141
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
int global_quality
Global quality for codecs which cannot change it per frame.
Definition: libavcodec/avcodec.h:1208
int gop_size
the number of pictures in a group of pictures, or 0 for intra_only
Definition: libavcodec/avcodec.h:1321
uint8_t palette_type
0=>default, 1=>custom - changed when palette regenerated.
Definition: flashsv2enc.c:113
common internal api header.
static void flush_put_bits(PutBitContext *s)
Pad the end of the output stream with zeros.
Definition: put_bits.h:81
static int write_all_blocks(FlashSV2Context *s, uint8_t *buf, int buf_size)
Definition: flashsv2enc.c:668
static void init_put_bits(PutBitContext *s, uint8_t *buffer, int buffer_size)
Initialize the PutBitContext s.
Definition: put_bits.h:54
static int encode_all_blocks(FlashSV2Context *s, int keyframe)
Definition: flashsv2enc.c:628
static void comp(unsigned char *dst, int dst_stride, unsigned char *src, int src_stride, int add)
Definition: eamad.c:71
Definition: avutil.h:143
static int optimum_block_height(FlashSV2Context *s)
Definition: flashsv2enc.c:749
static int write_bitstream(FlashSV2Context *s, const uint8_t *src, int stride, uint8_t *buf, int buf_size, int keyframe)
Definition: flashsv2enc.c:686
static int write_pixel_15_7(Palette *palette, uint8_t *dest, const uint8_t *src, int dist)
Definition: flashsv2enc.c:449
struct Palette Palette
bitstream writer API
Generated on Fri Aug 1 2025 06:53:41 for FFmpeg by
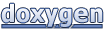