FFmpeg
|
wtvenc.c
Go to the documentation of this file.
42 {'t'_'i'_'m'_'e'_'l'_'i'_'n'_'e'_'.'_'t'_'a'_'b'_'l'_'e'_'.'_'0'_'.'_'h'_'e'_'a'_'d'_'e'_'r'_'.'_'E'_'v'_'e'_'n'_'t'_'s', 0};
44 {'t'_'a'_'b'_'l'_'e'_'.'_'0'_'.'_'h'_'e'_'a'_'d'_'e'_'r'_'.'_'l'_'e'_'g'_'a'_'c'_'y'_'_'_'a'_'t'_'t'_'r'_'i'_'b', 0};
46 {'t'_'a'_'b'_'l'_'e'_'.'_'0'_'.'_'r'_'e'_'d'_'i'_'r'_'e'_'c'_'t'_'o'_'r'_'.'_'l'_'e'_'g'_'a'_'c'_'y'_'_'_'a'_'t'_'t'_'r'_'i'_'b', 0};
150 static void write_chunk_header(AVFormatContext *s, const ff_asf_guid *guid, int length, int stream_id)
298 av_log(s, AV_LOG_ERROR, "write stream codec info failed codec_type(0x%x)\n", st->codec->codec_type);
336 av_log(s, AV_LOG_ERROR, "write stream codec info failed codec_type(0x%x)\n", st->codec->codec_type);
434 if (s->streams[pkt->stream_index]->codec->codec_id == AV_CODEC_ID_MJPEG && !wctx->thumbnail.size) {
440 if (wctx->serial - (wctx->nb_sp_pairs ? wctx->sp_pairs[wctx->nb_sp_pairs - 1].serial : 0) >= 50)
444 if (pkt->pts != AV_NOPTS_VALUE && pkt->pts - (wctx->nb_st_pairs ? wctx->st_pairs[wctx->nb_st_pairs - 1].value : 0) >= 5000000)
492 { timeline_table_0_header_events, sizeof(timeline_table_0_header_events), write_table0_header_events},
493 { ff_timeline_table_0_entries_Events_le16, sizeof(ff_timeline_table_0_entries_Events_le16), NULL},
495 { table_0_header_legacy_attrib, sizeof(table_0_header_legacy_attrib), write_table0_header_legacy_attrib},
559 static int64_t write_fat_sector(AVFormatContext *s, int64_t start_pos, int nb_sectors, int sector_bits, int depth)
676 pos += metadata_header_size("WM/Picture") + attachment_value_size(&wctx->thumbnail, av_dict_get(st->metadata, "title", NULL, 0));
710 } else if (w->length <= (int64_t)(WTV_SECTOR_SIZE / 4) * (WTV_SECTOR_SIZE / 4) * WTV_SECTOR_SIZE) {
713 } else if (w->length <= (int64_t)(WTV_SECTOR_SIZE / 4) * (WTV_SECTOR_SIZE / 4) * WTV_BIGSECTOR_SIZE) {
717 av_log(s, AV_LOG_ERROR, "unsupported file allocation table depth (%"PRIi64" bytes)\n", w->length);
733 w->first_sector = write_fat_sector(s, start_pos, nb_sectors, sector_bits, w->depth) >> WTV_SECTOR_BITS;
static void write_table_entries_time(AVFormatContext *s)
Definition: wtvenc.c:590
Definition: libavcodec/avcodec.h:100
Definition: wtvenc.c:82
Definition: dict.h:80
static int attachment_value_size(const AVPacket *pkt, const AVDictionaryEntry *e)
Definition: wtvenc.c:628
void avpriv_set_pts_info(AVStream *s, int pts_wrap_bits, unsigned int pts_num, unsigned int pts_den)
Set the time base and wrapping info for a given stream.
Definition: libavformat/utils.c:3952
static void write_fat(AVIOContext *pb, int start_sector, int nb_sectors, int shift)
Definition: wtvenc.c:549
Definition: wtvdec.c:308
int64_t avio_seek(AVIOContext *s, int64_t offset, int whence)
fseek() equivalent for AVIOContext.
Definition: aviobuf.c:199
int av_copy_packet(AVPacket *dst, AVPacket *src)
Copy packet, including contents.
Definition: avpacket.c:236
static int64_t write_fat_sector(AVFormatContext *s, int64_t start_pos, int nb_sectors, int sector_bits, int depth)
Definition: wtvenc.c:559
static void write_chunk_header2(AVFormatContext *s, const ff_asf_guid *guid, int stream_id)
Definition: wtvenc.c:172
void * av_realloc(void *ptr, size_t size)
Allocate or reallocate a block of memory.
Definition: mem.c:141
Definition: wtvenc.c:65
AVDictionaryEntry * av_dict_get(AVDictionary *m, const char *key, const AVDictionaryEntry *prev, int flags)
Get a dictionary entry with matching key.
Definition: dict.c:39
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining list
Definition: filter_design.txt:23
static int write_table0_header_time(AVIOContext *pb)
Definition: wtvenc.c:483
static int write_root_table(AVFormatContext *s, int64_t sector_pos)
Definition: wtvenc.c:502
static void write_table_redirector_legacy_attrib(AVFormatContext *s)
Definition: wtvenc.c:660
static void add_serial_pair(WtvSyncEntry **list, int *count, int64_t serial, int64_t value)
Definition: wtvenc.c:111
static const uint8_t table_0_redirector_legacy_attrib[]
Definition: wtvenc.c:45
Definition: wtvenc.c:61
Definition: wtvenc.c:58
Definition: libavformat/internal.h:35
static int write_stream_codec(AVFormatContext *s, AVStream *st)
Definition: wtvenc.c:286
Definition: wtvenc.c:63
Definition: wtvenc.c:75
static av_always_inline int64_t avio_tell(AVIOContext *s)
ftell() equivalent for AVIOContext.
Definition: avio.h:248
Definition: libavcodec/avcodec.h:110
void avio_write(AVIOContext *s, const unsigned char *buf, int size)
Definition: aviobuf.c:173
Definition: wtvdec.c:49
const uint8_t ff_table_0_entries_legacy_attrib_le16[]
Definition: wtv.c:54
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
static void write_chunk_header(AVFormatContext *s, const ff_asf_guid *guid, int length, int stream_id)
Write chunk header.
Definition: wtvenc.c:150
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
Definition: wtvenc.c:60
Definition: wtvenc.c:59
Definition: wtvenc.c:62
int void avio_flush(AVIOContext *s)
Force flushing of buffered data to the output s.
Definition: aviobuf.c:193
const ff_asf_guid ff_mediasubtype_cpfilters_processed
Definition: wtv.c:68
Definition: wtvenc.c:64
static int write_table0_header_legacy_attrib(AVIOContext *pb)
Definition: wtvenc.c:471
FFmpeg Automated Testing Environment ************************************Table of Contents *****************FFmpeg Automated Testing Environment Introduction Using FATE from your FFmpeg source directory Submitting the results to the FFmpeg result aggregation server FATE makefile targets and variables Makefile targets Makefile variables Examples Introduction **************FATE is an extended regression suite on the client side and a means for results aggregation and presentation on the server side The first part of this document explains how you can use FATE from your FFmpeg source directory to test your ffmpeg binary The second part describes how you can run FATE to submit the results to FFmpeg s FATE server In any way you can have a look at the publicly viewable FATE results by visiting this as it can be seen if some test on some platform broke with their recent contribution This usually happens on the platforms the developers could not test on The second part of this document describes how you can run FATE to submit your results to FFmpeg s FATE server If you want to submit your results be sure to check that your combination of OS and compiler is not already listed on the above mentioned website In the third part you can find a comprehensive listing of FATE makefile targets and variables Using FATE from your FFmpeg source directory **********************************************If you want to run FATE on your machine you need to have the samples in place You can get the samples via the build target fate rsync Use this command from the top level source this will cause FATE to fail NOTE To use a custom wrapper to run the pass target exec to configure or set the TARGET_EXEC Make variable Submitting the results to the FFmpeg result aggregation server ****************************************************************To submit your results to the server you should run fate through the shell script tests fate sh from the FFmpeg sources This script needs to be invoked with a configuration file as its first argument tests fate sh path to fate_config A configuration file template with comments describing the individual configuration variables can be found at doc fate_config sh template Create a configuration that suits your based on the configuration template The slot configuration variable can be any string that is not yet but it is suggested that you name it adhering to the following pattern< arch >< os >< compiler >< compiler version > The configuration file itself will be sourced in a shell therefore all shell features may be used This enables you to setup the environment as you need it for your build For your first test runs the fate_recv variable should be empty or commented out This will run everything as normal except that it will omit the submission of the results to the server The following files should be present in $workdir as specified in the configuration file
Definition: fate.txt:34
const uint8_t ff_timeline_table_0_entries_Events_le16[]
Definition: wtv.c:52
static void write_table_entries_attrib(AVFormatContext *s)
Definition: wtvenc.c:633
static const uint8_t timeline_table_0_header_events[]
Definition: wtvenc.c:41
static int finish_file(AVFormatContext *s, enum WtvFileIndex index, int64_t start_pos)
Pad the remainder of a file Write out fat table.
Definition: wtvenc.c:688
static void write_timestamp(AVFormatContext *s, AVPacket *pkt)
Definition: wtvenc.c:411
static int write_stream_codec_info(AVFormatContext *s, AVStream *st)
Definition: wtvenc.c:230
Definition: libavcodec/avcodec.h:384
static const ff_asf_guid * get_codec_guid(enum AVCodecID id, const AVCodecGuid *av_guid)
Definition: wtvenc.c:137
int avio_put_str16le(AVIOContext *s, const char *str)
Convert an UTF-8 string to UTF-16LE and write it.
Definition: aviobuf.c:318
static int write_table0_header_events(AVIOContext *pb)
Definition: wtvenc.c:463
#define type
Definition: riff.h:75
void ff_put_bmp_header(AVIOContext *pb, AVCodecContext *enc, const AVCodecTag *tags, int for_asf)
static void write_tag(AVIOContext *pb, const char *key, const char *value)
Definition: wtvenc.c:622
Main libavformat public API header.
static int write_stream_data(AVFormatContext *s, AVStream *st)
Definition: wtvenc.c:323
int ff_put_wav_header(AVIOContext *pb, AVCodecContext *enc)
Definition: avformat.h:377
static const uint8_t table_0_header_legacy_attrib[]
Definition: wtvenc.c:43
void ff_metadata_conv(AVDictionary **pm, const AVMetadataConv *d_conv, const AVMetadataConv *s_conv)
Definition: libavformat/metadata.c:26
static void write_table_entries_events(AVFormatContext *s)
Definition: wtvenc.c:579
Definition: avutil.h:143
static void write_tag_int32(AVIOContext *pb, const char *key, int value)
Definition: wtvenc.c:616
static void write_metadata_header(AVIOContext *pb, int type, const char *key, int value_size)
Definition: wtvenc.c:603
int64_t pts
Presentation timestamp in AVStream->time_base units; the time at which the decompressed packet will b...
Definition: libavcodec/avcodec.h:1044
Definition: wtvenc.c:57
Definition: wtvenc.c:124
Generated on Sat Feb 1 2025 06:52:32 for FFmpeg by
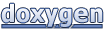