FFmpeg
|
rtpenc.c
Go to the documentation of this file.
35 { "payload_type", "Specify RTP payload type", offsetof(RTPMuxContext, payload_type), AV_OPT_TYPE_INT, {.i64 = -1 }, -1, 127, AV_OPT_FLAG_ENCODING_PARAM },
36 { "ssrc", "Stream identifier", offsetof(RTPMuxContext, ssrc), AV_OPT_TYPE_INT, { .i64 = 0 }, INT_MIN, INT_MAX, AV_OPT_FLAG_ENCODING_PARAM },
37 { "cname", "CNAME to include in RTCP SR packets", offsetof(RTPMuxContext, cname), AV_OPT_TYPE_STRING, { .str = NULL }, 0, 0, AV_OPT_FLAG_ENCODING_PARAM },
38 { "seq", "Starting sequence number", offsetof(RTPMuxContext, seq), AV_OPT_TYPE_INT, { .i64 = -1 }, -1, 65535, AV_OPT_FLAG_ENCODING_PARAM },
173 s->max_frames_per_packet = av_rescale_q(s1->max_delay, (AVRational){1, 1000000}, st->codec->time_base);
Definition: libavcodec/avcodec.h:367
Definition: libavcodec/avcodec.h:368
int64_t start_time_realtime
Start time of the stream in real world time, in microseconds since the unix epoch (00:00 1st January ...
Definition: avformat.h:1101
Definition: libavcodec/avcodec.h:303
Definition: libavcodec/avcodec.h:301
void avpriv_set_pts_info(AVStream *s, int pts_wrap_bits, unsigned int pts_num, unsigned int pts_den)
Set the time base and wrapping info for a given stream.
Definition: libavformat/utils.c:3952
Definition: opt.h:222
static int rtp_send_samples(AVFormatContext *s1, const uint8_t *buf1, int size, int sample_size_bits)
Definition: rtpenc.c:330
static int write_packet(AVFormatContext *s, AVPacket *pkt)
Definition: libavformat/assenc.c:63
static int rtp_write_packet(AVFormatContext *s1, AVPacket *pkt)
Definition: rtpenc.c:486
void ff_rtp_send_amr(AVFormatContext *s1, const uint8_t *buff, int size)
Packetize AMR frames into RTP packets according to RFC 3267, in octet-aligned mode.
Definition: rtpenc_amr.c:30
Definition: libavcodec/avcodec.h:115
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
Definition: libavcodec/avcodec.h:130
An AV_PKT_DATA_H263_MB_INFO side data packet contains a number of structures with info about macroblo...
Definition: libavcodec/avcodec.h:957
int block_align
number of bytes per packet if constant and known or 0 Used by some WAV based audio codecs...
Definition: libavcodec/avcodec.h:1898
int nal_length_size
Number of bytes used for H.264 NAL length, if the MP4 syntax is used (1, 2 or 4)
Definition: rtpenc.h:60
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
Definition: libavcodec/avcodec.h:417
Definition: libavcodec/avcodec.h:360
const char * class_name
The name of the class; usually it is the same name as the context structure type to which the AVClass...
Definition: log.h:55
AVOptions.
Definition: libavcodec/avcodec.h:383
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
void ff_rtp_send_h263(AVFormatContext *s1, const uint8_t *buf1, int size)
Packetize H.263 frames into RTP packets according to RFC 4629.
Definition: rtpenc_h263.c:43
Definition: opt.h:226
#define CODEC_FLAG_BITEXACT
Use only bitexact stuff (except (I)DCT).
Definition: libavcodec/avcodec.h:715
int bits_per_coded_sample
bits per sample/pixel from the demuxer (needed for huffyuv).
Definition: libavcodec/avcodec.h:2546
Definition: libavcodec/avcodec.h:110
void avio_write(AVIOContext *s, const unsigned char *buf, int size)
Definition: aviobuf.c:173
#define AV_OPT_FLAG_ENCODING_PARAM
a generic parameter which can be set by the user for muxing or encoding
Definition: opt.h:281
uint64_t ff_ntp_time(void)
Get the current time since NTP epoch in microseconds.
Definition: libavformat/utils.c:3679
int ff_rtp_get_payload_type(AVFormatContext *fmt, AVCodecContext *codec, int idx)
Return the payload type for a given stream used in the given format context.
Definition: rtp.c:89
int64_t av_rescale_q(int64_t a, AVRational bq, AVRational cq)
Rescale a 64-bit integer by 2 rational numbers.
Definition: mathematics.c:130
Definition: libavcodec/avcodec.h:103
Definition: libavcodec/avcodec.h:306
void ff_rtp_send_aac(AVFormatContext *s1, const uint8_t *buff, int size)
Definition: rtpenc_aac.c:25
void ff_rtp_send_jpeg(AVFormatContext *s1, const uint8_t *buff, int size)
Definition: rtpenc_jpeg.c:27
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
preferred ID for decoding MPEG audio layer 1, 2 or 3
Definition: libavcodec/avcodec.h:382
void ff_rtp_send_latm(AVFormatContext *s1, const uint8_t *buff, int size)
Definition: rtpenc_latm.c:25
Definition: avutil.h:144
void ff_rtp_send_vp8(AVFormatContext *s1, const uint8_t *buff, int size)
Definition: rtpenc_vp8.c:26
int64_t av_gcd(int64_t a, int64_t b)
Return the greatest common divisor of a and b.
Definition: mathematics.c:55
static void rtp_send_mpegts_raw(AVFormatContext *s1, const uint8_t *buf1, int size)
Definition: rtpenc.c:433
int64_t av_rescale_q_rnd(int64_t a, AVRational bq, AVRational cq, enum AVRounding rnd)
Rescale a 64-bit integer by 2 rational numbers with specified rounding.
Definition: mathematics.c:122
void ff_rtp_send_xiph(AVFormatContext *s1, const uint8_t *buff, int size)
Packetize Xiph frames into RTP according to RFC 5215 (Vorbis) and the Theora RFC draft.
Definition: rtpenc_xiph.c:31
int void avio_flush(AVIOContext *s)
Force flushing of buffered data to the output s.
Definition: aviobuf.c:193
void ff_rtp_send_data(AVFormatContext *s1, const uint8_t *buf1, int len, int m)
Definition: rtpenc.c:307
Definition: rtpenc.h:27
Definition: libavcodec/avcodec.h:302
Definition: libavcodec/avcodec.h:107
static int rtp_send_ilbc(AVFormatContext *s1, const uint8_t *buf, int size)
Definition: rtpenc.c:456
Definition: libavcodec/avcodec.h:449
void ff_rtp_send_mpegvideo(AVFormatContext *s1, const uint8_t *buf1, int size)
Definition: rtpenc_mpv.c:29
Definition: libavcodec/avcodec.h:243
static void rtp_send_mpegaudio(AVFormatContext *s1, const uint8_t *buf1, int size)
Definition: rtpenc.c:359
Definition: libavcodec/avcodec.h:343
Definition: libavcodec/avcodec.h:300
const char * avcodec_get_name(enum AVCodecID id)
Get the name of a codec.
Definition: libavcodec/utils.c:2416
Definition: libavcodec/avcodec.h:304
int frame_size
Number of samples per channel in an audio frame.
Definition: libavcodec/avcodec.h:1881
Definition: libavcodec/avcodec.h:133
Definition: libavcodec/avcodec.h:305
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
Definition: libavcodec/avcodec.h:381
Definition: libavcodec/avcodec.h:122
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
int av_get_audio_frame_duration(AVCodecContext *avctx, int frame_bytes)
Return audio frame duration.
Definition: libavcodec/utils.c:2708
void ff_rtp_send_h264(AVFormatContext *s1, const uint8_t *buf1, int size)
Definition: rtpenc_h264.c:84
Main libavformat public API header.
static void rtp_send_raw(AVFormatContext *s1, const uint8_t *buf1, int size)
Definition: rtpenc.c:411
Definition: libavcodec/avcodec.h:299
Definition: avformat.h:377
FAKE codec to indicate a raw MPEG-2 TS stream (only used by libavformat)
Definition: libavcodec/avcodec.h:492
void ff_rtp_send_h263_rfc2190(AVFormatContext *s1, const uint8_t *buf1, int size, const uint8_t *mb_info, int mb_info_size)
Definition: rtpenc_h263_rfc2190.c:101
Definition: avutil.h:143
uint8_t * av_packet_get_side_data(AVPacket *pkt, enum AVPacketSideDataType type, int *size)
Get side information from packet.
Definition: avpacket.c:289
uint32_t av_get_random_seed(void)
Get a seed to use in conjunction with random functions.
Definition: random_seed.c:105
Definition: libavcodec/avcodec.h:441
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: avformat.h:679
int64_t pts
Presentation timestamp in AVStream->time_base units; the time at which the decompressed packet will b...
Definition: libavcodec/avcodec.h:1044
Definition: libavcodec/avcodec.h:386
Generated on Fri May 30 2025 06:54:00 for FFmpeg by
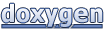