FFmpeg
|
libavformat/flvenc.c
Go to the documentation of this file.
389 if (enc->codec_id == AV_CODEC_ID_AAC || enc->codec_id == AV_CODEC_ID_H264 || enc->codec_id == AV_CODEC_ID_MPEG4) {
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
Definition: libavcodec/avcodec.h:100
Definition: dict.h:80
Definition: libavformat/flv.h:62
Definition: libavformat/flv.h:109
Definition: libavformat/flv.h:104
void avpriv_set_pts_info(AVStream *s, int pts_wrap_bits, unsigned int pts_num, unsigned int pts_den)
Set the time base and wrapping info for a given stream.
Definition: libavformat/utils.c:3952
static int write_packet(AVFormatContext *s, AVPacket *pkt)
Definition: libavformat/assenc.c:63
int64_t avio_seek(AVIOContext *s, int64_t offset, int whence)
fseek() equivalent for AVIOContext.
Definition: aviobuf.c:199
Definition: libavformat/flv.h:98
static av_always_inline uint64_t av_double2int(double f)
Reinterpret a double as a 64-bit integer.
Definition: intfloat.h:70
Definition: libavcodec/avcodec.h:115
int64_t avio_skip(AVIOContext *s, int64_t offset)
Skip given number of bytes forward.
Definition: aviobuf.c:256
Definition: libavcodec/avcodec.h:234
AVDictionaryEntry * av_dict_get(AVDictionary *m, const char *key, const AVDictionaryEntry *prev, int flags)
Get a dictionary entry with matching key.
Definition: dict.c:39
Definition: libavcodec/avcodec.h:130
static int get_audio_flags(AVFormatContext *s, AVCodecContext *enc)
Definition: libavformat/flvenc.c:72
#define AVFMT_TS_NONSTRICT
Format does not require strictly increasing timestamps, but they must still be monotonic.
Definition: avformat.h:363
Definition: libavcodec/avcodec.h:194
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
Definition: libavcodec/avcodec.h:417
internal metadata API header see avformat.h or the public API!
Public dictionary API.
Definition: libavformat/flv.h:107
Definition: libavcodec/avcodec.h:383
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
Definition: libavformat/flv.h:86
Definition: libavformat/internal.h:35
Definition: libavcodec/avcodec.h:124
Definition: libavformat/flv.h:108
Definition: libavformat/flv.h:91
static av_always_inline int64_t avio_tell(AVIOContext *s)
ftell() equivalent for AVIOContext.
Definition: avio.h:248
int bits_per_coded_sample
bits per sample/pixel from the demuxer (needed for huffyuv).
Definition: libavcodec/avcodec.h:2546
void avio_write(AVIOContext *s, const unsigned char *buf, int size)
Definition: aviobuf.c:173
struct FLVContext FLVContext
int duration
Duration of this packet in AVStream->time_base units, 0 if unknown.
Definition: libavcodec/avcodec.h:1073
Definition: libavcodec/avcodec.h:189
Definition: libavcodec/avcodec.h:209
Definition: libavformat/flv.h:61
Definition: libavcodec/avcodec.h:415
Definition: libavformat/flv.h:96
static int flv_write_trailer(AVFormatContext *s)
Definition: libavformat/flvenc.c:419
Definition: libavformat/flv.h:84
Definition: libavcodec/avcodec.h:306
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
Definition: libavformat/flv.h:60
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: libavformat/flvdec.c:42
preferred ID for decoding MPEG audio layer 1, 2 or 3
Definition: libavcodec/avcodec.h:382
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
int ff_avc_parse_nal_units_buf(const uint8_t *buf_in, uint8_t **buf, int *size)
Definition: avc.c:92
Definition: libavformat/flv.h:123
#define av_assert1(cond)
assert() equivalent, that does not lie in speed critical code.
Definition: avassert.h:53
Definition: libavformat/flv.h:111
Definition: libavformat/flv.h:125
static int flv_write_packet(AVFormatContext *s, AVPacket *pkt)
Definition: libavformat/flvenc.c:452
Definition: libavformat/flv.h:105
signifies 5512Hz and 8000Hz in the case of NELLYMOSER
Definition: libavformat/flv.h:83
Definition: libavcodec/avcodec.h:107
Definition: libavformat/flv.h:124
Definition: libavformat/flv.h:110
Definition: libavcodec/avcodec.h:300
const char * avcodec_get_name(enum AVCodecID id)
Get the name of a codec.
Definition: libavcodec/utils.c:2416
Definition: libavcodec/avcodec.h:304
FLV common header.
Definition: libavformat/flv.h:55
Definition: libavcodec/avcodec.h:305
unsigned int codec_tag
fourcc (LSB first, so "ABCD" -> ('D'<<24) + ('C'<<16) + ('B'<<8) + 'A').
Definition: libavcodec/avcodec.h:1160
static void put_amf_string(AVIOContext *pb, const char *str)
Definition: libavformat/flvenc.c:162
Definition: libavformat/flv.h:99
Definition: libavformat/flv.h:90
Definition: libavformat/flv.h:130
static void put_amf_double(AVIOContext *pb, double d)
Definition: libavformat/flvenc.c:182
Definition: libavformat/flv.h:79
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
Definition: libavformat/flv.h:56
Definition: libavformat/flv.h:100
const char * av_get_media_type_string(enum AVMediaType media_type)
Return a string describing the media_type enum, NULL if media_type is unknown.
Definition: libavutil/utils.c:64
Definition: libavformat/flv.h:106
Main libavformat public API header.
Definition: libavformat/flv.h:93
Definition: libavformat/flv.h:92
Definition: libavcodec/avcodec.h:299
Definition: avformat.h:377
Definition: libavformat/flv.h:74
static void put_avc_eos_tag(AVIOContext *pb, unsigned ts)
Definition: libavformat/flvenc.c:169
Definition: libavformat/flv.h:85
Definition: libavformat/flv.h:78
int64_t dts
Decompression timestamp in AVStream->time_base units; the time at which the packet is decompressed...
Definition: libavcodec/avcodec.h:1050
int64_t duration
Decoding: duration of the stream, in AV_TIME_BASE fractional seconds.
Definition: avformat.h:1009
Definition: avutil.h:143
Definition: libavcodec/avcodec.h:195
Definition: libavformat/flv.h:97
Definition: libavcodec/avcodec.h:345
Definition: libavformat/flvenc.c:68
struct FLVStreamContext FLVStreamContext
int64_t pts
Presentation timestamp in AVStream->time_base units; the time at which the decompressed packet will b...
Definition: libavcodec/avcodec.h:1044
int ff_isom_write_avcc(AVIOContext *pb, const uint8_t *data, int len)
Definition: avc.c:106
Generated on Sun Mar 23 2025 06:53:06 for FFmpeg by
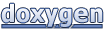