FFmpeg
|
electronicarts.c
Go to the documentation of this file.
Definition: libavcodec/avcodec.h:342
Definition: libavcodec/avcodec.h:100
static int process_audio_header_eacs(AVFormatContext *s)
Definition: electronicarts.c:225
Definition: libavcodec/avcodec.h:303
Definition: libavcodec/avcodec.h:232
void avpriv_set_pts_info(AVStream *s, int pts_wrap_bits, unsigned int pts_num, unsigned int pts_den)
Set the time base and wrapping info for a given stream.
Definition: libavformat/utils.c:3952
int64_t avio_seek(AVIOContext *s, int64_t offset, int whence)
fseek() equivalent for AVIOContext.
Definition: aviobuf.c:199
int64_t avio_skip(AVIOContext *s, int64_t offset)
Skip given number of bytes forward.
Definition: aviobuf.c:256
Definition: avformat.h:456
int block_align
number of bytes per packet if constant and known or 0 Used by some WAV based audio codecs...
Definition: libavcodec/avcodec.h:1898
Definition: libavcodec/avcodec.h:194
void void avpriv_request_sample(void *avc, const char *msg,...) av_printf_format(2
Log a generic warning message about a missing feature.
Definition: libavcodec/avcodec.h:224
AVStream * avformat_new_stream(AVFormatContext *s, const AVCodec *c)
Add a new stream to a media file.
Definition: libavformat/utils.c:3362
Definition: libavcodec/avcodec.h:223
Definition: libavcodec/avcodec.h:353
int av_get_packet(AVIOContext *s, AVPacket *pkt, int size)
Allocate and read the payload of a packet and initialize its fields with default values.
Definition: libavformat/utils.c:328
static av_always_inline int64_t avio_tell(AVIOContext *s)
ftell() equivalent for AVIOContext.
Definition: avio.h:248
int bits_per_coded_sample
bits per sample/pixel from the demuxer (needed for huffyuv).
Definition: libavcodec/avcodec.h:2546
int duration
Duration of this packet in AVStream->time_base units, 0 if unknown.
Definition: libavcodec/avcodec.h:1073
int av_append_packet(AVIOContext *s, AVPacket *pkt, int size)
Read data and append it to the current content of the AVPacket.
Definition: libavformat/utils.c:338
Definition: libavcodec/avcodec.h:225
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
preferred ID for decoding MPEG audio layer 1, 2 or 3
Definition: libavcodec/avcodec.h:382
Definition: avutil.h:144
static int process_video_header_vp6(AVFormatContext *s)
Definition: electronicarts.c:282
Definition: libavcodec/avcodec.h:352
unsigned char * buf
Buffer must have AVPROBE_PADDING_SIZE of extra allocated bytes filled with zero.
Definition: avformat.h:336
struct EaDemuxContext EaDemuxContext
static int process_audio_header_elements(AVFormatContext *s)
Definition: electronicarts.c:102
Definition: libavcodec/avcodec.h:140
Definition: libavcodec/avcodec.h:221
Definition: libavcodec/avcodec.h:305
Definition: electronicarts.c:63
unsigned int codec_tag
fourcc (LSB first, so "ABCD" -> ('D'<<24) + ('C'<<16) + ('B'<<8) + 'A').
Definition: libavcodec/avcodec.h:1160
static int process_video_header_mdec(AVFormatContext *s)
Definition: electronicarts.c:270
static int process_video_header_cmv(AVFormatContext *s)
Definition: electronicarts.c:301
static int process_audio_header_sead(AVFormatContext *s)
Definition: electronicarts.c:257
This structure contains the data a format has to probe a file.
Definition: avformat.h:334
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
static av_always_inline AVRational av_inv_q(AVRational q)
Invert a rational.
Definition: rational.h:122
#define AVPROBE_SCORE_MAX
maximum score, half of that is used for file-extension-based detection
Definition: avformat.h:340
Main libavformat public API header.
int64_t start_time
Decoding: pts of the first frame of the stream in presentation order, in stream time base...
Definition: avformat.h:689
Definition: libavcodec/avcodec.h:299
Definition: libavcodec/avcodec.h:354
Definition: avutil.h:143
static int ea_read_packet(AVFormatContext *s, AVPacket *pkt)
Definition: electronicarts.c:497
Generated on Thu May 29 2025 06:52:46 for FFmpeg by
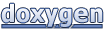